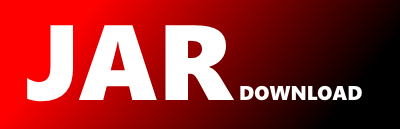
no.ks.fiks.arkiv.v1.innsyn.sok.SokVerdier Maven / Gradle / Ivy
//
// This file was generated by the Eclipse Implementation of JAXB, v2.3.6
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2023.06.23 at 10:32:49 AM CEST
//
package no.ks.fiks.arkiv.v1.innsyn.sok;
import java.util.HashMap;
import java.util.Map;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import com.kscs.util.jaxb.Buildable;
import com.kscs.util.jaxb.PropertyTree;
import com.kscs.util.jaxb.PropertyTreeUse;
/**
* Java class for sokVerdier complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="sokVerdier">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <choice minOccurs="0">
* <element name="stringvalues" type="{https://ks-no.github.io/standarder/fiks-protokoll/fiks-arkiv/sok/v1}stringvalues"/>
* <element name="datevalues" type="{https://ks-no.github.io/standarder/fiks-protokoll/fiks-arkiv/sok/v1}datevalues"/>
* <element name="intvalues" type="{https://ks-no.github.io/standarder/fiks-protokoll/fiks-arkiv/sok/v1}intvalues"/>
* <element name="eksternId" type="{https://ks-no.github.io/standarder/fiks-protokoll/fiks-arkiv/sok/v1}eksternId"/>
* <element name="klassifikasjonvalues" type="{https://ks-no.github.io/standarder/fiks-protokoll/fiks-arkiv/sok/v1}klassifikasjonvalues"/>
* <element name="bbox" type="{https://ks-no.github.io/standarder/fiks-protokoll/fiks-arkiv/sok/v1}bbox"/>
* </choice>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "sokVerdier", propOrder = {
"bbox",
"klassifikasjonvalues",
"eksternId",
"intvalues",
"datevalues",
"stringvalues"
})
public class SokVerdier {
protected Bbox bbox;
protected Klassifikasjonvalues klassifikasjonvalues;
protected EksternId eksternId;
protected Intvalues intvalues;
protected Datevalues datevalues;
protected Stringvalues stringvalues;
/**
* Gets the value of the bbox property.
*
* @return
* possible object is
* {@link Bbox }
*
*/
public Bbox getBbox() {
return bbox;
}
/**
* Sets the value of the bbox property.
*
* @param value
* allowed object is
* {@link Bbox }
*
*/
public void setBbox(Bbox value) {
this.bbox = value;
}
/**
* Gets the value of the klassifikasjonvalues property.
*
* @return
* possible object is
* {@link Klassifikasjonvalues }
*
*/
public Klassifikasjonvalues getKlassifikasjonvalues() {
return klassifikasjonvalues;
}
/**
* Sets the value of the klassifikasjonvalues property.
*
* @param value
* allowed object is
* {@link Klassifikasjonvalues }
*
*/
public void setKlassifikasjonvalues(Klassifikasjonvalues value) {
this.klassifikasjonvalues = value;
}
/**
* Gets the value of the eksternId property.
*
* @return
* possible object is
* {@link EksternId }
*
*/
public EksternId getEksternId() {
return eksternId;
}
/**
* Sets the value of the eksternId property.
*
* @param value
* allowed object is
* {@link EksternId }
*
*/
public void setEksternId(EksternId value) {
this.eksternId = value;
}
/**
* Gets the value of the intvalues property.
*
* @return
* possible object is
* {@link Intvalues }
*
*/
public Intvalues getIntvalues() {
return intvalues;
}
/**
* Sets the value of the intvalues property.
*
* @param value
* allowed object is
* {@link Intvalues }
*
*/
public void setIntvalues(Intvalues value) {
this.intvalues = value;
}
/**
* Gets the value of the datevalues property.
*
* @return
* possible object is
* {@link Datevalues }
*
*/
public Datevalues getDatevalues() {
return datevalues;
}
/**
* Sets the value of the datevalues property.
*
* @param value
* allowed object is
* {@link Datevalues }
*
*/
public void setDatevalues(Datevalues value) {
this.datevalues = value;
}
/**
* Gets the value of the stringvalues property.
*
* @return
* possible object is
* {@link Stringvalues }
*
*/
public Stringvalues getStringvalues() {
return stringvalues;
}
/**
* Sets the value of the stringvalues property.
*
* @param value
* allowed object is
* {@link Stringvalues }
*
*/
public void setStringvalues(Stringvalues value) {
this.stringvalues = value;
}
/**
* Copies all state of this object to a builder. This method is used by the {@link
* #copyOf} method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final SokVerdier.Builder<_B> _other) {
_other.bbox = ((this.bbox == null)?null:this.bbox.newCopyBuilder(_other));
_other.klassifikasjonvalues = ((this.klassifikasjonvalues == null)?null:this.klassifikasjonvalues.newCopyBuilder(_other));
_other.eksternId = ((this.eksternId == null)?null:this.eksternId.newCopyBuilder(_other));
_other.intvalues = ((this.intvalues == null)?null:this.intvalues.newCopyBuilder(_other));
_other.datevalues = ((this.datevalues == null)?null:this.datevalues.newCopyBuilder(_other));
_other.stringvalues = ((this.stringvalues == null)?null:this.stringvalues.newCopyBuilder(_other));
}
public<_B >SokVerdier.Builder<_B> newCopyBuilder(final _B _parentBuilder) {
return new SokVerdier.Builder<_B>(_parentBuilder, this, true);
}
public SokVerdier.Builder newCopyBuilder() {
return newCopyBuilder(null);
}
public static SokVerdier.Builder builder() {
return new SokVerdier.Builder(null, null, false);
}
public static<_B >SokVerdier.Builder<_B> copyOf(final SokVerdier _other) {
final SokVerdier.Builder<_B> _newBuilder = new SokVerdier.Builder<_B>(null, null, false);
_other.copyTo(_newBuilder);
return _newBuilder;
}
/**
* Copies all state of this object to a builder. This method is used by the {@link
* #copyOf} method and should not be called directly by client code.
*
* @param _other
* A builder instance to which the state of this object will be copied.
*/
public<_B >void copyTo(final SokVerdier.Builder<_B> _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final PropertyTree bboxPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("bbox"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(bboxPropertyTree!= null):((bboxPropertyTree == null)||(!bboxPropertyTree.isLeaf())))) {
_other.bbox = ((this.bbox == null)?null:this.bbox.newCopyBuilder(_other, bboxPropertyTree, _propertyTreeUse));
}
final PropertyTree klassifikasjonvaluesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("klassifikasjonvalues"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(klassifikasjonvaluesPropertyTree!= null):((klassifikasjonvaluesPropertyTree == null)||(!klassifikasjonvaluesPropertyTree.isLeaf())))) {
_other.klassifikasjonvalues = ((this.klassifikasjonvalues == null)?null:this.klassifikasjonvalues.newCopyBuilder(_other, klassifikasjonvaluesPropertyTree, _propertyTreeUse));
}
final PropertyTree eksternIdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("eksternId"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(eksternIdPropertyTree!= null):((eksternIdPropertyTree == null)||(!eksternIdPropertyTree.isLeaf())))) {
_other.eksternId = ((this.eksternId == null)?null:this.eksternId.newCopyBuilder(_other, eksternIdPropertyTree, _propertyTreeUse));
}
final PropertyTree intvaluesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("intvalues"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(intvaluesPropertyTree!= null):((intvaluesPropertyTree == null)||(!intvaluesPropertyTree.isLeaf())))) {
_other.intvalues = ((this.intvalues == null)?null:this.intvalues.newCopyBuilder(_other, intvaluesPropertyTree, _propertyTreeUse));
}
final PropertyTree datevaluesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("datevalues"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(datevaluesPropertyTree!= null):((datevaluesPropertyTree == null)||(!datevaluesPropertyTree.isLeaf())))) {
_other.datevalues = ((this.datevalues == null)?null:this.datevalues.newCopyBuilder(_other, datevaluesPropertyTree, _propertyTreeUse));
}
final PropertyTree stringvaluesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("stringvalues"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(stringvaluesPropertyTree!= null):((stringvaluesPropertyTree == null)||(!stringvaluesPropertyTree.isLeaf())))) {
_other.stringvalues = ((this.stringvalues == null)?null:this.stringvalues.newCopyBuilder(_other, stringvaluesPropertyTree, _propertyTreeUse));
}
}
public<_B >SokVerdier.Builder<_B> newCopyBuilder(final _B _parentBuilder, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return new SokVerdier.Builder<_B>(_parentBuilder, this, true, _propertyTree, _propertyTreeUse);
}
public SokVerdier.Builder newCopyBuilder(final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
return newCopyBuilder(null, _propertyTree, _propertyTreeUse);
}
public static<_B >SokVerdier.Builder<_B> copyOf(final SokVerdier _other, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
final SokVerdier.Builder<_B> _newBuilder = new SokVerdier.Builder<_B>(null, null, false);
_other.copyTo(_newBuilder, _propertyTree, _propertyTreeUse);
return _newBuilder;
}
public static SokVerdier.Builder copyExcept(final SokVerdier _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.EXCLUDE);
}
public static SokVerdier.Builder copyOnly(final SokVerdier _other, final PropertyTree _propertyTree) {
return copyOf(_other, _propertyTree, PropertyTreeUse.INCLUDE);
}
public static class Builder<_B >implements Buildable
{
protected final _B _parentBuilder;
protected final SokVerdier _storedValue;
private Bbox.Builder> bbox;
private Klassifikasjonvalues.Builder> klassifikasjonvalues;
private EksternId.Builder> eksternId;
private Intvalues.Builder> intvalues;
private Datevalues.Builder> datevalues;
private Stringvalues.Builder> stringvalues;
public Builder(final _B _parentBuilder, final SokVerdier _other, final boolean _copy) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
this.bbox = ((_other.bbox == null)?null:_other.bbox.newCopyBuilder(this));
this.klassifikasjonvalues = ((_other.klassifikasjonvalues == null)?null:_other.klassifikasjonvalues.newCopyBuilder(this));
this.eksternId = ((_other.eksternId == null)?null:_other.eksternId.newCopyBuilder(this));
this.intvalues = ((_other.intvalues == null)?null:_other.intvalues.newCopyBuilder(this));
this.datevalues = ((_other.datevalues == null)?null:_other.datevalues.newCopyBuilder(this));
this.stringvalues = ((_other.stringvalues == null)?null:_other.stringvalues.newCopyBuilder(this));
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public Builder(final _B _parentBuilder, final SokVerdier _other, final boolean _copy, final PropertyTree _propertyTree, final PropertyTreeUse _propertyTreeUse) {
this._parentBuilder = _parentBuilder;
if (_other!= null) {
if (_copy) {
_storedValue = null;
final PropertyTree bboxPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("bbox"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(bboxPropertyTree!= null):((bboxPropertyTree == null)||(!bboxPropertyTree.isLeaf())))) {
this.bbox = ((_other.bbox == null)?null:_other.bbox.newCopyBuilder(this, bboxPropertyTree, _propertyTreeUse));
}
final PropertyTree klassifikasjonvaluesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("klassifikasjonvalues"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(klassifikasjonvaluesPropertyTree!= null):((klassifikasjonvaluesPropertyTree == null)||(!klassifikasjonvaluesPropertyTree.isLeaf())))) {
this.klassifikasjonvalues = ((_other.klassifikasjonvalues == null)?null:_other.klassifikasjonvalues.newCopyBuilder(this, klassifikasjonvaluesPropertyTree, _propertyTreeUse));
}
final PropertyTree eksternIdPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("eksternId"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(eksternIdPropertyTree!= null):((eksternIdPropertyTree == null)||(!eksternIdPropertyTree.isLeaf())))) {
this.eksternId = ((_other.eksternId == null)?null:_other.eksternId.newCopyBuilder(this, eksternIdPropertyTree, _propertyTreeUse));
}
final PropertyTree intvaluesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("intvalues"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(intvaluesPropertyTree!= null):((intvaluesPropertyTree == null)||(!intvaluesPropertyTree.isLeaf())))) {
this.intvalues = ((_other.intvalues == null)?null:_other.intvalues.newCopyBuilder(this, intvaluesPropertyTree, _propertyTreeUse));
}
final PropertyTree datevaluesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("datevalues"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(datevaluesPropertyTree!= null):((datevaluesPropertyTree == null)||(!datevaluesPropertyTree.isLeaf())))) {
this.datevalues = ((_other.datevalues == null)?null:_other.datevalues.newCopyBuilder(this, datevaluesPropertyTree, _propertyTreeUse));
}
final PropertyTree stringvaluesPropertyTree = ((_propertyTree == null)?null:_propertyTree.get("stringvalues"));
if (((_propertyTreeUse == PropertyTreeUse.INCLUDE)?(stringvaluesPropertyTree!= null):((stringvaluesPropertyTree == null)||(!stringvaluesPropertyTree.isLeaf())))) {
this.stringvalues = ((_other.stringvalues == null)?null:_other.stringvalues.newCopyBuilder(this, stringvaluesPropertyTree, _propertyTreeUse));
}
} else {
_storedValue = _other;
}
} else {
_storedValue = null;
}
}
public _B end() {
return this._parentBuilder;
}
protected<_P extends SokVerdier >_P init(final _P _product) {
_product.bbox = ((this.bbox == null)?null:this.bbox.build());
_product.klassifikasjonvalues = ((this.klassifikasjonvalues == null)?null:this.klassifikasjonvalues.build());
_product.eksternId = ((this.eksternId == null)?null:this.eksternId.build());
_product.intvalues = ((this.intvalues == null)?null:this.intvalues.build());
_product.datevalues = ((this.datevalues == null)?null:this.datevalues.build());
_product.stringvalues = ((this.stringvalues == null)?null:this.stringvalues.build());
return _product;
}
/**
* Sets the new value of "bbox" (any previous value will be replaced)
*
* @param bbox
* New value of the "bbox" property.
*/
public SokVerdier.Builder<_B> withBbox(final Bbox bbox) {
this.bbox = ((bbox == null)?null:new Bbox.Builder>(this, bbox, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the "bbox"
* property.
* Use {@link no.ks.fiks.arkiv.v1.innsyn.sok.Bbox.Builder#end()} to return to the
* current builder.
*
* @return
* A new builder to build the value of the "bbox" property.
* Use {@link no.ks.fiks.arkiv.v1.innsyn.sok.Bbox.Builder#end()} to return to the
* current builder.
*/
public Bbox.Builder extends SokVerdier.Builder<_B>> withBbox() {
if (this.bbox!= null) {
return this.bbox;
}
return this.bbox = new Bbox.Builder>(this, null, false);
}
/**
* Sets the new value of "klassifikasjonvalues" (any previous value will be
* replaced)
*
* @param klassifikasjonvalues
* New value of the "klassifikasjonvalues" property.
*/
public SokVerdier.Builder<_B> withKlassifikasjonvalues(final Klassifikasjonvalues klassifikasjonvalues) {
this.klassifikasjonvalues = ((klassifikasjonvalues == null)?null:new Klassifikasjonvalues.Builder>(this, klassifikasjonvalues, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "klassifikasjonvalues" property.
* Use {@link no.ks.fiks.arkiv.v1.innsyn.sok.Klassifikasjonvalues.Builder#end()} to
* return to the current builder.
*
* @return
* A new builder to build the value of the "klassifikasjonvalues" property.
* Use {@link no.ks.fiks.arkiv.v1.innsyn.sok.Klassifikasjonvalues.Builder#end()} to
* return to the current builder.
*/
public Klassifikasjonvalues.Builder extends SokVerdier.Builder<_B>> withKlassifikasjonvalues() {
if (this.klassifikasjonvalues!= null) {
return this.klassifikasjonvalues;
}
return this.klassifikasjonvalues = new Klassifikasjonvalues.Builder>(this, null, false);
}
/**
* Sets the new value of "eksternId" (any previous value will be replaced)
*
* @param eksternId
* New value of the "eksternId" property.
*/
public SokVerdier.Builder<_B> withEksternId(final EksternId eksternId) {
this.eksternId = ((eksternId == null)?null:new EksternId.Builder>(this, eksternId, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "eksternId" property.
* Use {@link no.ks.fiks.arkiv.v1.innsyn.sok.EksternId.Builder#end()} to return to
* the current builder.
*
* @return
* A new builder to build the value of the "eksternId" property.
* Use {@link no.ks.fiks.arkiv.v1.innsyn.sok.EksternId.Builder#end()} to return to
* the current builder.
*/
public EksternId.Builder extends SokVerdier.Builder<_B>> withEksternId() {
if (this.eksternId!= null) {
return this.eksternId;
}
return this.eksternId = new EksternId.Builder>(this, null, false);
}
/**
* Sets the new value of "intvalues" (any previous value will be replaced)
*
* @param intvalues
* New value of the "intvalues" property.
*/
public SokVerdier.Builder<_B> withIntvalues(final Intvalues intvalues) {
this.intvalues = ((intvalues == null)?null:new Intvalues.Builder>(this, intvalues, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "intvalues" property.
* Use {@link no.ks.fiks.arkiv.v1.innsyn.sok.Intvalues.Builder#end()} to return to
* the current builder.
*
* @return
* A new builder to build the value of the "intvalues" property.
* Use {@link no.ks.fiks.arkiv.v1.innsyn.sok.Intvalues.Builder#end()} to return to
* the current builder.
*/
public Intvalues.Builder extends SokVerdier.Builder<_B>> withIntvalues() {
if (this.intvalues!= null) {
return this.intvalues;
}
return this.intvalues = new Intvalues.Builder>(this, null, false);
}
/**
* Sets the new value of "datevalues" (any previous value will be replaced)
*
* @param datevalues
* New value of the "datevalues" property.
*/
public SokVerdier.Builder<_B> withDatevalues(final Datevalues datevalues) {
this.datevalues = ((datevalues == null)?null:new Datevalues.Builder>(this, datevalues, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "datevalues" property.
* Use {@link no.ks.fiks.arkiv.v1.innsyn.sok.Datevalues.Builder#end()} to return to
* the current builder.
*
* @return
* A new builder to build the value of the "datevalues" property.
* Use {@link no.ks.fiks.arkiv.v1.innsyn.sok.Datevalues.Builder#end()} to return to
* the current builder.
*/
public Datevalues.Builder extends SokVerdier.Builder<_B>> withDatevalues() {
if (this.datevalues!= null) {
return this.datevalues;
}
return this.datevalues = new Datevalues.Builder>(this, null, false);
}
/**
* Sets the new value of "stringvalues" (any previous value will be replaced)
*
* @param stringvalues
* New value of the "stringvalues" property.
*/
public SokVerdier.Builder<_B> withStringvalues(final Stringvalues stringvalues) {
this.stringvalues = ((stringvalues == null)?null:new Stringvalues.Builder>(this, stringvalues, false));
return this;
}
/**
* Returns the existing builder or a new builder to build the value of the
* "stringvalues" property.
* Use {@link no.ks.fiks.arkiv.v1.innsyn.sok.Stringvalues.Builder#end()} to return
* to the current builder.
*
* @return
* A new builder to build the value of the "stringvalues" property.
* Use {@link no.ks.fiks.arkiv.v1.innsyn.sok.Stringvalues.Builder#end()} to return
* to the current builder.
*/
public Stringvalues.Builder extends SokVerdier.Builder<_B>> withStringvalues() {
if (this.stringvalues!= null) {
return this.stringvalues;
}
return this.stringvalues = new Stringvalues.Builder>(this, null, false);
}
@Override
public SokVerdier build() {
if (_storedValue == null) {
return this.init(new SokVerdier());
} else {
return ((SokVerdier) _storedValue);
}
}
public SokVerdier.Builder<_B> copyOf(final SokVerdier _other) {
_other.copyTo(this);
return this;
}
public SokVerdier.Builder<_B> copyOf(final SokVerdier.Builder _other) {
return copyOf(_other.build());
}
}
public static class Select
extends SokVerdier.Selector
{
Select() {
super(null, null, null);
}
public static SokVerdier.Select _root() {
return new SokVerdier.Select();
}
}
public static class Selector , TParent >
extends com.kscs.util.jaxb.Selector
{
private Bbox.Selector> bbox = null;
private Klassifikasjonvalues.Selector> klassifikasjonvalues = null;
private EksternId.Selector> eksternId = null;
private Intvalues.Selector> intvalues = null;
private Datevalues.Selector> datevalues = null;
private Stringvalues.Selector> stringvalues = null;
public Selector(final TRoot root, final TParent parent, final String propertyName) {
super(root, parent, propertyName);
}
@Override
public Map buildChildren() {
final Map products = new HashMap();
products.putAll(super.buildChildren());
if (this.bbox!= null) {
products.put("bbox", this.bbox.init());
}
if (this.klassifikasjonvalues!= null) {
products.put("klassifikasjonvalues", this.klassifikasjonvalues.init());
}
if (this.eksternId!= null) {
products.put("eksternId", this.eksternId.init());
}
if (this.intvalues!= null) {
products.put("intvalues", this.intvalues.init());
}
if (this.datevalues!= null) {
products.put("datevalues", this.datevalues.init());
}
if (this.stringvalues!= null) {
products.put("stringvalues", this.stringvalues.init());
}
return products;
}
public Bbox.Selector> bbox() {
return ((this.bbox == null)?this.bbox = new Bbox.Selector>(this._root, this, "bbox"):this.bbox);
}
public Klassifikasjonvalues.Selector> klassifikasjonvalues() {
return ((this.klassifikasjonvalues == null)?this.klassifikasjonvalues = new Klassifikasjonvalues.Selector>(this._root, this, "klassifikasjonvalues"):this.klassifikasjonvalues);
}
public EksternId.Selector> eksternId() {
return ((this.eksternId == null)?this.eksternId = new EksternId.Selector>(this._root, this, "eksternId"):this.eksternId);
}
public Intvalues.Selector> intvalues() {
return ((this.intvalues == null)?this.intvalues = new Intvalues.Selector>(this._root, this, "intvalues"):this.intvalues);
}
public Datevalues.Selector> datevalues() {
return ((this.datevalues == null)?this.datevalues = new Datevalues.Selector>(this._root, this, "datevalues"):this.datevalues);
}
public Stringvalues.Selector> stringvalues() {
return ((this.stringvalues == null)?this.stringvalues = new Stringvalues.Selector>(this._root, this, "stringvalues"):this.stringvalues);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy