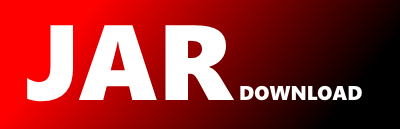
no.nav.melding.virksomhet.henvendelsebehandling.behandlingsresultat.v1.XMLHenvendelse Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.4
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2013.09.18 at 04:46:59 PM CEST
//
package no.nav.melding.virksomhet.henvendelsebehandling.behandlingsresultat.v1;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.joda.time.DateTime;
import org.w3._2001.xmlschema.Adapter1;
/**
* Java class for Henvendelse complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Henvendelse">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="behandlingsId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="aktor" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="henvendelseType" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="tema" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="traad" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="lestDato" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* <element name="opprettetDato" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* <element name="sensitiv" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="behandlingsresultat" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Henvendelse", propOrder = {
"behandlingsId",
"aktor",
"henvendelseType",
"tema",
"traad",
"lestDato",
"opprettetDato",
"sensitiv",
"behandlingsresultat"
})
public class XMLHenvendelse {
protected String behandlingsId;
protected String aktor;
protected String henvendelseType;
protected String tema;
protected String traad;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected DateTime lestDato;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected DateTime opprettetDato;
protected Boolean sensitiv;
protected String behandlingsresultat;
/**
* Gets the value of the behandlingsId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBehandlingsId() {
return behandlingsId;
}
/**
* Sets the value of the behandlingsId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBehandlingsId(String value) {
this.behandlingsId = value;
}
/**
* Gets the value of the aktor property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAktor() {
return aktor;
}
/**
* Sets the value of the aktor property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAktor(String value) {
this.aktor = value;
}
/**
* Gets the value of the henvendelseType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getHenvendelseType() {
return henvendelseType;
}
/**
* Sets the value of the henvendelseType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHenvendelseType(String value) {
this.henvendelseType = value;
}
/**
* Gets the value of the tema property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTema() {
return tema;
}
/**
* Sets the value of the tema property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTema(String value) {
this.tema = value;
}
/**
* Gets the value of the traad property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTraad() {
return traad;
}
/**
* Sets the value of the traad property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTraad(String value) {
this.traad = value;
}
/**
* Gets the value of the lestDato property.
*
* @return
* possible object is
* {@link String }
*
*/
public DateTime getLestDato() {
return lestDato;
}
/**
* Sets the value of the lestDato property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLestDato(DateTime value) {
this.lestDato = value;
}
/**
* Gets the value of the opprettetDato property.
*
* @return
* possible object is
* {@link String }
*
*/
public DateTime getOpprettetDato() {
return opprettetDato;
}
/**
* Sets the value of the opprettetDato property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOpprettetDato(DateTime value) {
this.opprettetDato = value;
}
/**
* Gets the value of the sensitiv property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isSensitiv() {
return sensitiv;
}
/**
* Sets the value of the sensitiv property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setSensitiv(Boolean value) {
this.sensitiv = value;
}
/**
* Gets the value of the behandlingsresultat property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBehandlingsresultat() {
return behandlingsresultat;
}
/**
* Sets the value of the behandlingsresultat property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBehandlingsresultat(String value) {
this.behandlingsresultat = value;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy