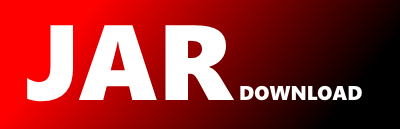
no.nav.gosys.journal.Bruker Maven / Gradle / Ivy
package no.nav.gosys.journal;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
* Java class for Bruker complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Bruker">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="brukerId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="brukerInfoId" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="brukertype" type="{http://nav.no/virksomhet/gjennomforing/arkiv/journal/v2}Brukertype" minOccurs="0"/>
* <element name="sporing" type="{http://nav.no/virksomhet/gjennomforing/arkiv/journal/v2}Sporing" minOccurs="0"/>
* <element name="versjon" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="utvidelse" type="{http://nav.no/virksomhet/gjennomforing/arkiv/journal/v2}BrukerUtvidelse1" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Bruker", propOrder = {
"brukerId",
"brukerInfoId",
"brukertype",
"sporing",
"versjon",
"utvidelse"
})
public class Bruker
implements Equals, HashCode, ToString
{
protected String brukerId;
protected Long brukerInfoId;
protected Brukertype brukertype;
protected Sporing sporing;
protected Long versjon;
protected BrukerUtvidelse1 utvidelse;
/**
* Gets the value of the brukerId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBrukerId() {
return brukerId;
}
/**
* Sets the value of the brukerId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBrukerId(String value) {
this.brukerId = value;
}
/**
* Gets the value of the brukerInfoId property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getBrukerInfoId() {
return brukerInfoId;
}
/**
* Sets the value of the brukerInfoId property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setBrukerInfoId(Long value) {
this.brukerInfoId = value;
}
/**
* Gets the value of the brukertype property.
*
* @return
* possible object is
* {@link Brukertype }
*
*/
public Brukertype getBrukertype() {
return brukertype;
}
/**
* Sets the value of the brukertype property.
*
* @param value
* allowed object is
* {@link Brukertype }
*
*/
public void setBrukertype(Brukertype value) {
this.brukertype = value;
}
/**
* Gets the value of the sporing property.
*
* @return
* possible object is
* {@link Sporing }
*
*/
public Sporing getSporing() {
return sporing;
}
/**
* Sets the value of the sporing property.
*
* @param value
* allowed object is
* {@link Sporing }
*
*/
public void setSporing(Sporing value) {
this.sporing = value;
}
/**
* Gets the value of the versjon property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getVersjon() {
return versjon;
}
/**
* Sets the value of the versjon property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setVersjon(Long value) {
this.versjon = value;
}
/**
* Gets the value of the utvidelse property.
*
* @return
* possible object is
* {@link BrukerUtvidelse1 }
*
*/
public BrukerUtvidelse1 getUtvidelse() {
return utvidelse;
}
/**
* Sets the value of the utvidelse property.
*
* @param value
* allowed object is
* {@link BrukerUtvidelse1 }
*
*/
public void setUtvidelse(BrukerUtvidelse1 value) {
this.utvidelse = value;
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
String theBrukerId;
theBrukerId = this.getBrukerId();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "brukerId", theBrukerId), currentHashCode, theBrukerId);
}
{
Long theBrukerInfoId;
theBrukerInfoId = this.getBrukerInfoId();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "brukerInfoId", theBrukerInfoId), currentHashCode, theBrukerInfoId);
}
{
Brukertype theBrukertype;
theBrukertype = this.getBrukertype();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "brukertype", theBrukertype), currentHashCode, theBrukertype);
}
{
Sporing theSporing;
theSporing = this.getSporing();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "sporing", theSporing), currentHashCode, theSporing);
}
{
Long theVersjon;
theVersjon = this.getVersjon();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "versjon", theVersjon), currentHashCode, theVersjon);
}
{
BrukerUtvidelse1 theUtvidelse;
theUtvidelse = this.getUtvidelse();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "utvidelse", theUtvidelse), currentHashCode, theUtvidelse);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof Bruker)) {
return false;
}
if (this == object) {
return true;
}
final Bruker that = ((Bruker) object);
{
String lhsBrukerId;
lhsBrukerId = this.getBrukerId();
String rhsBrukerId;
rhsBrukerId = that.getBrukerId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "brukerId", lhsBrukerId), LocatorUtils.property(thatLocator, "brukerId", rhsBrukerId), lhsBrukerId, rhsBrukerId)) {
return false;
}
}
{
Long lhsBrukerInfoId;
lhsBrukerInfoId = this.getBrukerInfoId();
Long rhsBrukerInfoId;
rhsBrukerInfoId = that.getBrukerInfoId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "brukerInfoId", lhsBrukerInfoId), LocatorUtils.property(thatLocator, "brukerInfoId", rhsBrukerInfoId), lhsBrukerInfoId, rhsBrukerInfoId)) {
return false;
}
}
{
Brukertype lhsBrukertype;
lhsBrukertype = this.getBrukertype();
Brukertype rhsBrukertype;
rhsBrukertype = that.getBrukertype();
if (!strategy.equals(LocatorUtils.property(thisLocator, "brukertype", lhsBrukertype), LocatorUtils.property(thatLocator, "brukertype", rhsBrukertype), lhsBrukertype, rhsBrukertype)) {
return false;
}
}
{
Sporing lhsSporing;
lhsSporing = this.getSporing();
Sporing rhsSporing;
rhsSporing = that.getSporing();
if (!strategy.equals(LocatorUtils.property(thisLocator, "sporing", lhsSporing), LocatorUtils.property(thatLocator, "sporing", rhsSporing), lhsSporing, rhsSporing)) {
return false;
}
}
{
Long lhsVersjon;
lhsVersjon = this.getVersjon();
Long rhsVersjon;
rhsVersjon = that.getVersjon();
if (!strategy.equals(LocatorUtils.property(thisLocator, "versjon", lhsVersjon), LocatorUtils.property(thatLocator, "versjon", rhsVersjon), lhsVersjon, rhsVersjon)) {
return false;
}
}
{
BrukerUtvidelse1 lhsUtvidelse;
lhsUtvidelse = this.getUtvidelse();
BrukerUtvidelse1 rhsUtvidelse;
rhsUtvidelse = that.getUtvidelse();
if (!strategy.equals(LocatorUtils.property(thisLocator, "utvidelse", lhsUtvidelse), LocatorUtils.property(thatLocator, "utvidelse", rhsUtvidelse), lhsUtvidelse, rhsUtvidelse)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theBrukerId;
theBrukerId = this.getBrukerId();
strategy.appendField(locator, this, "brukerId", buffer, theBrukerId);
}
{
Long theBrukerInfoId;
theBrukerInfoId = this.getBrukerInfoId();
strategy.appendField(locator, this, "brukerInfoId", buffer, theBrukerInfoId);
}
{
Brukertype theBrukertype;
theBrukertype = this.getBrukertype();
strategy.appendField(locator, this, "brukertype", buffer, theBrukertype);
}
{
Sporing theSporing;
theSporing = this.getSporing();
strategy.appendField(locator, this, "sporing", buffer, theSporing);
}
{
Long theVersjon;
theVersjon = this.getVersjon();
strategy.appendField(locator, this, "versjon", buffer, theVersjon);
}
{
BrukerUtvidelse1 theUtvidelse;
theUtvidelse = this.getUtvidelse();
strategy.appendField(locator, this, "utvidelse", buffer, theUtvidelse);
}
return buffer;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy