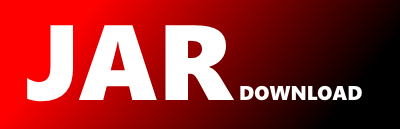
no.nav.gosys.journal.DokumentInfo Maven / Gradle / Ivy
package no.nav.gosys.journal;
import java.util.Calendar;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
import org.w3._2001.xmlschema.Adapter1;
/**
* Java class for DokumentInfo complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="DokumentInfo">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="dokumentInfoId" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="skannetInnholdListe" type="{http://nav.no/virksomhet/gjennomforing/arkiv/journal/v2}SkannetInnhold" maxOccurs="unbounded" minOccurs="0"/>
* <element name="fildetaljerListe" type="{http://nav.no/virksomhet/gjennomforing/arkiv/journal/v2}Fildetaljer" maxOccurs="unbounded" minOccurs="0"/>
* <element name="kategori" type="{http://nav.no/virksomhet/gjennomforing/arkiv/journal/v2}Dokumentkategori" minOccurs="0"/>
* <element name="dokumentFerdigDato" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* <element name="dokumentstatus" type="{http://nav.no/virksomhet/gjennomforing/arkiv/journal/v2}Dokumentstatus" minOccurs="0"/>
* <element name="tittel" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="brevkode" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="brevgruppe" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="originalJournalpostId" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="konfidensialitet" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="sensitivt" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="integritet" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="tilgjengelighet" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="innskrenketPartsinnsyn" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="organInternt" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="konvertertFraSystem" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="sporing" type="{http://nav.no/virksomhet/gjennomforing/arkiv/journal/v2}Sporing" minOccurs="0"/>
* <element name="versjon" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="utvidelse" type="{http://nav.no/virksomhet/gjennomforing/arkiv/journal/v2}DokumentInfoUtvidelse1" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "DokumentInfo", propOrder = {
"dokumentInfoId",
"skannetInnholdListe",
"fildetaljerListe",
"kategori",
"dokumentFerdigDato",
"dokumentstatus",
"tittel",
"brevkode",
"brevgruppe",
"originalJournalpostId",
"konfidensialitet",
"sensitivt",
"integritet",
"tilgjengelighet",
"innskrenketPartsinnsyn",
"organInternt",
"konvertertFraSystem",
"sporing",
"versjon",
"utvidelse"
})
public class DokumentInfo
implements Equals, HashCode, ToString
{
protected Long dokumentInfoId;
protected no.nav.gosys.journal.SkannetInnhold[] skannetInnholdListe;
protected no.nav.gosys.journal.Fildetaljer[] fildetaljerListe;
protected Dokumentkategori kategori;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected Calendar dokumentFerdigDato;
protected Dokumentstatus dokumentstatus;
protected String tittel;
protected String brevkode;
protected String brevgruppe;
protected Long originalJournalpostId;
protected String konfidensialitet;
protected Boolean sensitivt;
protected String integritet;
protected String tilgjengelighet;
protected Boolean innskrenketPartsinnsyn;
protected Boolean organInternt;
protected String konvertertFraSystem;
protected Sporing sporing;
protected Long versjon;
protected DokumentInfoUtvidelse1 utvidelse;
/**
* Gets the value of the dokumentInfoId property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getDokumentInfoId() {
return dokumentInfoId;
}
/**
* Sets the value of the dokumentInfoId property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setDokumentInfoId(Long value) {
this.dokumentInfoId = value;
}
/**
*
*
* @return
* array of
* {@link no.nav.gosys.journal.SkannetInnhold }
*
*/
public no.nav.gosys.journal.SkannetInnhold[] getSkannetInnholdListe() {
if (this.skannetInnholdListe == null) {
return new no.nav.gosys.journal.SkannetInnhold[ 0 ] ;
}
no.nav.gosys.journal.SkannetInnhold[] retVal = new no.nav.gosys.journal.SkannetInnhold[this.skannetInnholdListe.length] ;
System.arraycopy(this.skannetInnholdListe, 0, retVal, 0, this.skannetInnholdListe.length);
return (retVal);
}
/**
*
*
* @return
* one of
* {@link no.nav.gosys.journal.SkannetInnhold }
*
*/
public no.nav.gosys.journal.SkannetInnhold getSkannetInnholdListe(int idx) {
if (this.skannetInnholdListe == null) {
throw new IndexOutOfBoundsException();
}
return this.skannetInnholdListe[idx];
}
public int getSkannetInnholdListeLength() {
if (this.skannetInnholdListe == null) {
return 0;
}
return this.skannetInnholdListe.length;
}
/**
*
*
* @param values
* allowed objects are
* {@link no.nav.gosys.journal.SkannetInnhold }
*
*/
public void setSkannetInnholdListe(no.nav.gosys.journal.SkannetInnhold[] values) {
int len = values.length;
this.skannetInnholdListe = ((no.nav.gosys.journal.SkannetInnhold[]) new no.nav.gosys.journal.SkannetInnhold[len] );
for (int i = 0; (i 0))?this.getSkannetInnholdListe():null);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "skannetInnholdListe", theSkannetInnholdListe), currentHashCode, theSkannetInnholdListe);
}
{
no.nav.gosys.journal.Fildetaljer[] theFildetaljerListe;
theFildetaljerListe = (((this.fildetaljerListe!= null)&&(this.fildetaljerListe.length > 0))?this.getFildetaljerListe():null);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "fildetaljerListe", theFildetaljerListe), currentHashCode, theFildetaljerListe);
}
{
Dokumentkategori theKategori;
theKategori = this.getKategori();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "kategori", theKategori), currentHashCode, theKategori);
}
{
Calendar theDokumentFerdigDato;
theDokumentFerdigDato = this.getDokumentFerdigDato();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "dokumentFerdigDato", theDokumentFerdigDato), currentHashCode, theDokumentFerdigDato);
}
{
Dokumentstatus theDokumentstatus;
theDokumentstatus = this.getDokumentstatus();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "dokumentstatus", theDokumentstatus), currentHashCode, theDokumentstatus);
}
{
String theTittel;
theTittel = this.getTittel();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "tittel", theTittel), currentHashCode, theTittel);
}
{
String theBrevkode;
theBrevkode = this.getBrevkode();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "brevkode", theBrevkode), currentHashCode, theBrevkode);
}
{
String theBrevgruppe;
theBrevgruppe = this.getBrevgruppe();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "brevgruppe", theBrevgruppe), currentHashCode, theBrevgruppe);
}
{
Long theOriginalJournalpostId;
theOriginalJournalpostId = this.getOriginalJournalpostId();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "originalJournalpostId", theOriginalJournalpostId), currentHashCode, theOriginalJournalpostId);
}
{
String theKonfidensialitet;
theKonfidensialitet = this.getKonfidensialitet();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "konfidensialitet", theKonfidensialitet), currentHashCode, theKonfidensialitet);
}
{
Boolean theSensitivt;
theSensitivt = this.getSensitivt();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "sensitivt", theSensitivt), currentHashCode, theSensitivt);
}
{
String theIntegritet;
theIntegritet = this.getIntegritet();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "integritet", theIntegritet), currentHashCode, theIntegritet);
}
{
String theTilgjengelighet;
theTilgjengelighet = this.getTilgjengelighet();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "tilgjengelighet", theTilgjengelighet), currentHashCode, theTilgjengelighet);
}
{
Boolean theInnskrenketPartsinnsyn;
theInnskrenketPartsinnsyn = this.getInnskrenketPartsinnsyn();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "innskrenketPartsinnsyn", theInnskrenketPartsinnsyn), currentHashCode, theInnskrenketPartsinnsyn);
}
{
Boolean theOrganInternt;
theOrganInternt = this.getOrganInternt();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "organInternt", theOrganInternt), currentHashCode, theOrganInternt);
}
{
String theKonvertertFraSystem;
theKonvertertFraSystem = this.getKonvertertFraSystem();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "konvertertFraSystem", theKonvertertFraSystem), currentHashCode, theKonvertertFraSystem);
}
{
Sporing theSporing;
theSporing = this.getSporing();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "sporing", theSporing), currentHashCode, theSporing);
}
{
Long theVersjon;
theVersjon = this.getVersjon();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "versjon", theVersjon), currentHashCode, theVersjon);
}
{
DokumentInfoUtvidelse1 theUtvidelse;
theUtvidelse = this.getUtvidelse();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "utvidelse", theUtvidelse), currentHashCode, theUtvidelse);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof DokumentInfo)) {
return false;
}
if (this == object) {
return true;
}
final DokumentInfo that = ((DokumentInfo) object);
{
Long lhsDokumentInfoId;
lhsDokumentInfoId = this.getDokumentInfoId();
Long rhsDokumentInfoId;
rhsDokumentInfoId = that.getDokumentInfoId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "dokumentInfoId", lhsDokumentInfoId), LocatorUtils.property(thatLocator, "dokumentInfoId", rhsDokumentInfoId), lhsDokumentInfoId, rhsDokumentInfoId)) {
return false;
}
}
{
no.nav.gosys.journal.SkannetInnhold[] lhsSkannetInnholdListe;
lhsSkannetInnholdListe = (((this.skannetInnholdListe!= null)&&(this.skannetInnholdListe.length > 0))?this.getSkannetInnholdListe():null);
no.nav.gosys.journal.SkannetInnhold[] rhsSkannetInnholdListe;
rhsSkannetInnholdListe = (((that.skannetInnholdListe!= null)&&(that.skannetInnholdListe.length > 0))?that.getSkannetInnholdListe():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "skannetInnholdListe", lhsSkannetInnholdListe), LocatorUtils.property(thatLocator, "skannetInnholdListe", rhsSkannetInnholdListe), lhsSkannetInnholdListe, rhsSkannetInnholdListe)) {
return false;
}
}
{
no.nav.gosys.journal.Fildetaljer[] lhsFildetaljerListe;
lhsFildetaljerListe = (((this.fildetaljerListe!= null)&&(this.fildetaljerListe.length > 0))?this.getFildetaljerListe():null);
no.nav.gosys.journal.Fildetaljer[] rhsFildetaljerListe;
rhsFildetaljerListe = (((that.fildetaljerListe!= null)&&(that.fildetaljerListe.length > 0))?that.getFildetaljerListe():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "fildetaljerListe", lhsFildetaljerListe), LocatorUtils.property(thatLocator, "fildetaljerListe", rhsFildetaljerListe), lhsFildetaljerListe, rhsFildetaljerListe)) {
return false;
}
}
{
Dokumentkategori lhsKategori;
lhsKategori = this.getKategori();
Dokumentkategori rhsKategori;
rhsKategori = that.getKategori();
if (!strategy.equals(LocatorUtils.property(thisLocator, "kategori", lhsKategori), LocatorUtils.property(thatLocator, "kategori", rhsKategori), lhsKategori, rhsKategori)) {
return false;
}
}
{
Calendar lhsDokumentFerdigDato;
lhsDokumentFerdigDato = this.getDokumentFerdigDato();
Calendar rhsDokumentFerdigDato;
rhsDokumentFerdigDato = that.getDokumentFerdigDato();
if (!strategy.equals(LocatorUtils.property(thisLocator, "dokumentFerdigDato", lhsDokumentFerdigDato), LocatorUtils.property(thatLocator, "dokumentFerdigDato", rhsDokumentFerdigDato), lhsDokumentFerdigDato, rhsDokumentFerdigDato)) {
return false;
}
}
{
Dokumentstatus lhsDokumentstatus;
lhsDokumentstatus = this.getDokumentstatus();
Dokumentstatus rhsDokumentstatus;
rhsDokumentstatus = that.getDokumentstatus();
if (!strategy.equals(LocatorUtils.property(thisLocator, "dokumentstatus", lhsDokumentstatus), LocatorUtils.property(thatLocator, "dokumentstatus", rhsDokumentstatus), lhsDokumentstatus, rhsDokumentstatus)) {
return false;
}
}
{
String lhsTittel;
lhsTittel = this.getTittel();
String rhsTittel;
rhsTittel = that.getTittel();
if (!strategy.equals(LocatorUtils.property(thisLocator, "tittel", lhsTittel), LocatorUtils.property(thatLocator, "tittel", rhsTittel), lhsTittel, rhsTittel)) {
return false;
}
}
{
String lhsBrevkode;
lhsBrevkode = this.getBrevkode();
String rhsBrevkode;
rhsBrevkode = that.getBrevkode();
if (!strategy.equals(LocatorUtils.property(thisLocator, "brevkode", lhsBrevkode), LocatorUtils.property(thatLocator, "brevkode", rhsBrevkode), lhsBrevkode, rhsBrevkode)) {
return false;
}
}
{
String lhsBrevgruppe;
lhsBrevgruppe = this.getBrevgruppe();
String rhsBrevgruppe;
rhsBrevgruppe = that.getBrevgruppe();
if (!strategy.equals(LocatorUtils.property(thisLocator, "brevgruppe", lhsBrevgruppe), LocatorUtils.property(thatLocator, "brevgruppe", rhsBrevgruppe), lhsBrevgruppe, rhsBrevgruppe)) {
return false;
}
}
{
Long lhsOriginalJournalpostId;
lhsOriginalJournalpostId = this.getOriginalJournalpostId();
Long rhsOriginalJournalpostId;
rhsOriginalJournalpostId = that.getOriginalJournalpostId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "originalJournalpostId", lhsOriginalJournalpostId), LocatorUtils.property(thatLocator, "originalJournalpostId", rhsOriginalJournalpostId), lhsOriginalJournalpostId, rhsOriginalJournalpostId)) {
return false;
}
}
{
String lhsKonfidensialitet;
lhsKonfidensialitet = this.getKonfidensialitet();
String rhsKonfidensialitet;
rhsKonfidensialitet = that.getKonfidensialitet();
if (!strategy.equals(LocatorUtils.property(thisLocator, "konfidensialitet", lhsKonfidensialitet), LocatorUtils.property(thatLocator, "konfidensialitet", rhsKonfidensialitet), lhsKonfidensialitet, rhsKonfidensialitet)) {
return false;
}
}
{
Boolean lhsSensitivt;
lhsSensitivt = this.getSensitivt();
Boolean rhsSensitivt;
rhsSensitivt = that.getSensitivt();
if (!strategy.equals(LocatorUtils.property(thisLocator, "sensitivt", lhsSensitivt), LocatorUtils.property(thatLocator, "sensitivt", rhsSensitivt), lhsSensitivt, rhsSensitivt)) {
return false;
}
}
{
String lhsIntegritet;
lhsIntegritet = this.getIntegritet();
String rhsIntegritet;
rhsIntegritet = that.getIntegritet();
if (!strategy.equals(LocatorUtils.property(thisLocator, "integritet", lhsIntegritet), LocatorUtils.property(thatLocator, "integritet", rhsIntegritet), lhsIntegritet, rhsIntegritet)) {
return false;
}
}
{
String lhsTilgjengelighet;
lhsTilgjengelighet = this.getTilgjengelighet();
String rhsTilgjengelighet;
rhsTilgjengelighet = that.getTilgjengelighet();
if (!strategy.equals(LocatorUtils.property(thisLocator, "tilgjengelighet", lhsTilgjengelighet), LocatorUtils.property(thatLocator, "tilgjengelighet", rhsTilgjengelighet), lhsTilgjengelighet, rhsTilgjengelighet)) {
return false;
}
}
{
Boolean lhsInnskrenketPartsinnsyn;
lhsInnskrenketPartsinnsyn = this.getInnskrenketPartsinnsyn();
Boolean rhsInnskrenketPartsinnsyn;
rhsInnskrenketPartsinnsyn = that.getInnskrenketPartsinnsyn();
if (!strategy.equals(LocatorUtils.property(thisLocator, "innskrenketPartsinnsyn", lhsInnskrenketPartsinnsyn), LocatorUtils.property(thatLocator, "innskrenketPartsinnsyn", rhsInnskrenketPartsinnsyn), lhsInnskrenketPartsinnsyn, rhsInnskrenketPartsinnsyn)) {
return false;
}
}
{
Boolean lhsOrganInternt;
lhsOrganInternt = this.getOrganInternt();
Boolean rhsOrganInternt;
rhsOrganInternt = that.getOrganInternt();
if (!strategy.equals(LocatorUtils.property(thisLocator, "organInternt", lhsOrganInternt), LocatorUtils.property(thatLocator, "organInternt", rhsOrganInternt), lhsOrganInternt, rhsOrganInternt)) {
return false;
}
}
{
String lhsKonvertertFraSystem;
lhsKonvertertFraSystem = this.getKonvertertFraSystem();
String rhsKonvertertFraSystem;
rhsKonvertertFraSystem = that.getKonvertertFraSystem();
if (!strategy.equals(LocatorUtils.property(thisLocator, "konvertertFraSystem", lhsKonvertertFraSystem), LocatorUtils.property(thatLocator, "konvertertFraSystem", rhsKonvertertFraSystem), lhsKonvertertFraSystem, rhsKonvertertFraSystem)) {
return false;
}
}
{
Sporing lhsSporing;
lhsSporing = this.getSporing();
Sporing rhsSporing;
rhsSporing = that.getSporing();
if (!strategy.equals(LocatorUtils.property(thisLocator, "sporing", lhsSporing), LocatorUtils.property(thatLocator, "sporing", rhsSporing), lhsSporing, rhsSporing)) {
return false;
}
}
{
Long lhsVersjon;
lhsVersjon = this.getVersjon();
Long rhsVersjon;
rhsVersjon = that.getVersjon();
if (!strategy.equals(LocatorUtils.property(thisLocator, "versjon", lhsVersjon), LocatorUtils.property(thatLocator, "versjon", rhsVersjon), lhsVersjon, rhsVersjon)) {
return false;
}
}
{
DokumentInfoUtvidelse1 lhsUtvidelse;
lhsUtvidelse = this.getUtvidelse();
DokumentInfoUtvidelse1 rhsUtvidelse;
rhsUtvidelse = that.getUtvidelse();
if (!strategy.equals(LocatorUtils.property(thisLocator, "utvidelse", lhsUtvidelse), LocatorUtils.property(thatLocator, "utvidelse", rhsUtvidelse), lhsUtvidelse, rhsUtvidelse)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
Long theDokumentInfoId;
theDokumentInfoId = this.getDokumentInfoId();
strategy.appendField(locator, this, "dokumentInfoId", buffer, theDokumentInfoId);
}
{
no.nav.gosys.journal.SkannetInnhold[] theSkannetInnholdListe;
theSkannetInnholdListe = (((this.skannetInnholdListe!= null)&&(this.skannetInnholdListe.length > 0))?this.getSkannetInnholdListe():null);
strategy.appendField(locator, this, "skannetInnholdListe", buffer, theSkannetInnholdListe);
}
{
no.nav.gosys.journal.Fildetaljer[] theFildetaljerListe;
theFildetaljerListe = (((this.fildetaljerListe!= null)&&(this.fildetaljerListe.length > 0))?this.getFildetaljerListe():null);
strategy.appendField(locator, this, "fildetaljerListe", buffer, theFildetaljerListe);
}
{
Dokumentkategori theKategori;
theKategori = this.getKategori();
strategy.appendField(locator, this, "kategori", buffer, theKategori);
}
{
Calendar theDokumentFerdigDato;
theDokumentFerdigDato = this.getDokumentFerdigDato();
strategy.appendField(locator, this, "dokumentFerdigDato", buffer, theDokumentFerdigDato);
}
{
Dokumentstatus theDokumentstatus;
theDokumentstatus = this.getDokumentstatus();
strategy.appendField(locator, this, "dokumentstatus", buffer, theDokumentstatus);
}
{
String theTittel;
theTittel = this.getTittel();
strategy.appendField(locator, this, "tittel", buffer, theTittel);
}
{
String theBrevkode;
theBrevkode = this.getBrevkode();
strategy.appendField(locator, this, "brevkode", buffer, theBrevkode);
}
{
String theBrevgruppe;
theBrevgruppe = this.getBrevgruppe();
strategy.appendField(locator, this, "brevgruppe", buffer, theBrevgruppe);
}
{
Long theOriginalJournalpostId;
theOriginalJournalpostId = this.getOriginalJournalpostId();
strategy.appendField(locator, this, "originalJournalpostId", buffer, theOriginalJournalpostId);
}
{
String theKonfidensialitet;
theKonfidensialitet = this.getKonfidensialitet();
strategy.appendField(locator, this, "konfidensialitet", buffer, theKonfidensialitet);
}
{
Boolean theSensitivt;
theSensitivt = this.getSensitivt();
strategy.appendField(locator, this, "sensitivt", buffer, theSensitivt);
}
{
String theIntegritet;
theIntegritet = this.getIntegritet();
strategy.appendField(locator, this, "integritet", buffer, theIntegritet);
}
{
String theTilgjengelighet;
theTilgjengelighet = this.getTilgjengelighet();
strategy.appendField(locator, this, "tilgjengelighet", buffer, theTilgjengelighet);
}
{
Boolean theInnskrenketPartsinnsyn;
theInnskrenketPartsinnsyn = this.getInnskrenketPartsinnsyn();
strategy.appendField(locator, this, "innskrenketPartsinnsyn", buffer, theInnskrenketPartsinnsyn);
}
{
Boolean theOrganInternt;
theOrganInternt = this.getOrganInternt();
strategy.appendField(locator, this, "organInternt", buffer, theOrganInternt);
}
{
String theKonvertertFraSystem;
theKonvertertFraSystem = this.getKonvertertFraSystem();
strategy.appendField(locator, this, "konvertertFraSystem", buffer, theKonvertertFraSystem);
}
{
Sporing theSporing;
theSporing = this.getSporing();
strategy.appendField(locator, this, "sporing", buffer, theSporing);
}
{
Long theVersjon;
theVersjon = this.getVersjon();
strategy.appendField(locator, this, "versjon", buffer, theVersjon);
}
{
DokumentInfoUtvidelse1 theUtvidelse;
theUtvidelse = this.getUtvidelse();
strategy.appendField(locator, this, "utvidelse", buffer, theUtvidelse);
}
return buffer;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy