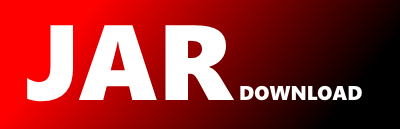
no.nav.gosys.journal.JournalpostDokumentInfoRelasjon Maven / Gradle / Ivy
package no.nav.gosys.journal;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
* Java class for JournalpostDokumentInfoRelasjon complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="JournalpostDokumentInfoRelasjon">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="journalpostDokumentInfoRelasjonId" type="{http://www.w3.org/2001/XMLSchema}long" minOccurs="0"/>
* <element name="dokumentInfo" type="{http://nav.no/virksomhet/gjennomforing/arkiv/journal/v2}DokumentInfo" minOccurs="0"/>
* <element name="tilknyttetJournalpostSom" type="{http://nav.no/virksomhet/gjennomforing/arkiv/journal/v2}TilknyttetJournalpostSom" minOccurs="0"/>
* <element name="tilknyttetAvNavn" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="sporing" type="{http://nav.no/virksomhet/gjennomforing/arkiv/journal/v2}Sporing" minOccurs="0"/>
* <element name="versjon" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="utvidelse" type="{http://nav.no/virksomhet/gjennomforing/arkiv/journal/v2}JournalpostDokumentInfoRelasjonUtvidelse1" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "JournalpostDokumentInfoRelasjon", propOrder = {
"journalpostDokumentInfoRelasjonId",
"dokumentInfo",
"tilknyttetJournalpostSom",
"tilknyttetAvNavn",
"sporing",
"versjon",
"utvidelse"
})
public class JournalpostDokumentInfoRelasjon
implements Equals, HashCode, ToString
{
protected Long journalpostDokumentInfoRelasjonId;
protected DokumentInfo dokumentInfo;
protected TilknyttetJournalpostSom tilknyttetJournalpostSom;
protected String tilknyttetAvNavn;
protected Sporing sporing;
protected String versjon;
protected JournalpostDokumentInfoRelasjonUtvidelse1 utvidelse;
/**
* Gets the value of the journalpostDokumentInfoRelasjonId property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getJournalpostDokumentInfoRelasjonId() {
return journalpostDokumentInfoRelasjonId;
}
/**
* Sets the value of the journalpostDokumentInfoRelasjonId property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setJournalpostDokumentInfoRelasjonId(Long value) {
this.journalpostDokumentInfoRelasjonId = value;
}
/**
* Gets the value of the dokumentInfo property.
*
* @return
* possible object is
* {@link DokumentInfo }
*
*/
public DokumentInfo getDokumentInfo() {
return dokumentInfo;
}
/**
* Sets the value of the dokumentInfo property.
*
* @param value
* allowed object is
* {@link DokumentInfo }
*
*/
public void setDokumentInfo(DokumentInfo value) {
this.dokumentInfo = value;
}
/**
* Gets the value of the tilknyttetJournalpostSom property.
*
* @return
* possible object is
* {@link TilknyttetJournalpostSom }
*
*/
public TilknyttetJournalpostSom getTilknyttetJournalpostSom() {
return tilknyttetJournalpostSom;
}
/**
* Sets the value of the tilknyttetJournalpostSom property.
*
* @param value
* allowed object is
* {@link TilknyttetJournalpostSom }
*
*/
public void setTilknyttetJournalpostSom(TilknyttetJournalpostSom value) {
this.tilknyttetJournalpostSom = value;
}
/**
* Gets the value of the tilknyttetAvNavn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTilknyttetAvNavn() {
return tilknyttetAvNavn;
}
/**
* Sets the value of the tilknyttetAvNavn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTilknyttetAvNavn(String value) {
this.tilknyttetAvNavn = value;
}
/**
* Gets the value of the sporing property.
*
* @return
* possible object is
* {@link Sporing }
*
*/
public Sporing getSporing() {
return sporing;
}
/**
* Sets the value of the sporing property.
*
* @param value
* allowed object is
* {@link Sporing }
*
*/
public void setSporing(Sporing value) {
this.sporing = value;
}
/**
* Gets the value of the versjon property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVersjon() {
return versjon;
}
/**
* Sets the value of the versjon property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVersjon(String value) {
this.versjon = value;
}
/**
* Gets the value of the utvidelse property.
*
* @return
* possible object is
* {@link JournalpostDokumentInfoRelasjonUtvidelse1 }
*
*/
public JournalpostDokumentInfoRelasjonUtvidelse1 getUtvidelse() {
return utvidelse;
}
/**
* Sets the value of the utvidelse property.
*
* @param value
* allowed object is
* {@link JournalpostDokumentInfoRelasjonUtvidelse1 }
*
*/
public void setUtvidelse(JournalpostDokumentInfoRelasjonUtvidelse1 value) {
this.utvidelse = value;
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
Long theJournalpostDokumentInfoRelasjonId;
theJournalpostDokumentInfoRelasjonId = this.getJournalpostDokumentInfoRelasjonId();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "journalpostDokumentInfoRelasjonId", theJournalpostDokumentInfoRelasjonId), currentHashCode, theJournalpostDokumentInfoRelasjonId);
}
{
DokumentInfo theDokumentInfo;
theDokumentInfo = this.getDokumentInfo();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "dokumentInfo", theDokumentInfo), currentHashCode, theDokumentInfo);
}
{
TilknyttetJournalpostSom theTilknyttetJournalpostSom;
theTilknyttetJournalpostSom = this.getTilknyttetJournalpostSom();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "tilknyttetJournalpostSom", theTilknyttetJournalpostSom), currentHashCode, theTilknyttetJournalpostSom);
}
{
String theTilknyttetAvNavn;
theTilknyttetAvNavn = this.getTilknyttetAvNavn();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "tilknyttetAvNavn", theTilknyttetAvNavn), currentHashCode, theTilknyttetAvNavn);
}
{
Sporing theSporing;
theSporing = this.getSporing();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "sporing", theSporing), currentHashCode, theSporing);
}
{
String theVersjon;
theVersjon = this.getVersjon();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "versjon", theVersjon), currentHashCode, theVersjon);
}
{
JournalpostDokumentInfoRelasjonUtvidelse1 theUtvidelse;
theUtvidelse = this.getUtvidelse();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "utvidelse", theUtvidelse), currentHashCode, theUtvidelse);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof JournalpostDokumentInfoRelasjon)) {
return false;
}
if (this == object) {
return true;
}
final JournalpostDokumentInfoRelasjon that = ((JournalpostDokumentInfoRelasjon) object);
{
Long lhsJournalpostDokumentInfoRelasjonId;
lhsJournalpostDokumentInfoRelasjonId = this.getJournalpostDokumentInfoRelasjonId();
Long rhsJournalpostDokumentInfoRelasjonId;
rhsJournalpostDokumentInfoRelasjonId = that.getJournalpostDokumentInfoRelasjonId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "journalpostDokumentInfoRelasjonId", lhsJournalpostDokumentInfoRelasjonId), LocatorUtils.property(thatLocator, "journalpostDokumentInfoRelasjonId", rhsJournalpostDokumentInfoRelasjonId), lhsJournalpostDokumentInfoRelasjonId, rhsJournalpostDokumentInfoRelasjonId)) {
return false;
}
}
{
DokumentInfo lhsDokumentInfo;
lhsDokumentInfo = this.getDokumentInfo();
DokumentInfo rhsDokumentInfo;
rhsDokumentInfo = that.getDokumentInfo();
if (!strategy.equals(LocatorUtils.property(thisLocator, "dokumentInfo", lhsDokumentInfo), LocatorUtils.property(thatLocator, "dokumentInfo", rhsDokumentInfo), lhsDokumentInfo, rhsDokumentInfo)) {
return false;
}
}
{
TilknyttetJournalpostSom lhsTilknyttetJournalpostSom;
lhsTilknyttetJournalpostSom = this.getTilknyttetJournalpostSom();
TilknyttetJournalpostSom rhsTilknyttetJournalpostSom;
rhsTilknyttetJournalpostSom = that.getTilknyttetJournalpostSom();
if (!strategy.equals(LocatorUtils.property(thisLocator, "tilknyttetJournalpostSom", lhsTilknyttetJournalpostSom), LocatorUtils.property(thatLocator, "tilknyttetJournalpostSom", rhsTilknyttetJournalpostSom), lhsTilknyttetJournalpostSom, rhsTilknyttetJournalpostSom)) {
return false;
}
}
{
String lhsTilknyttetAvNavn;
lhsTilknyttetAvNavn = this.getTilknyttetAvNavn();
String rhsTilknyttetAvNavn;
rhsTilknyttetAvNavn = that.getTilknyttetAvNavn();
if (!strategy.equals(LocatorUtils.property(thisLocator, "tilknyttetAvNavn", lhsTilknyttetAvNavn), LocatorUtils.property(thatLocator, "tilknyttetAvNavn", rhsTilknyttetAvNavn), lhsTilknyttetAvNavn, rhsTilknyttetAvNavn)) {
return false;
}
}
{
Sporing lhsSporing;
lhsSporing = this.getSporing();
Sporing rhsSporing;
rhsSporing = that.getSporing();
if (!strategy.equals(LocatorUtils.property(thisLocator, "sporing", lhsSporing), LocatorUtils.property(thatLocator, "sporing", rhsSporing), lhsSporing, rhsSporing)) {
return false;
}
}
{
String lhsVersjon;
lhsVersjon = this.getVersjon();
String rhsVersjon;
rhsVersjon = that.getVersjon();
if (!strategy.equals(LocatorUtils.property(thisLocator, "versjon", lhsVersjon), LocatorUtils.property(thatLocator, "versjon", rhsVersjon), lhsVersjon, rhsVersjon)) {
return false;
}
}
{
JournalpostDokumentInfoRelasjonUtvidelse1 lhsUtvidelse;
lhsUtvidelse = this.getUtvidelse();
JournalpostDokumentInfoRelasjonUtvidelse1 rhsUtvidelse;
rhsUtvidelse = that.getUtvidelse();
if (!strategy.equals(LocatorUtils.property(thisLocator, "utvidelse", lhsUtvidelse), LocatorUtils.property(thatLocator, "utvidelse", rhsUtvidelse), lhsUtvidelse, rhsUtvidelse)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
Long theJournalpostDokumentInfoRelasjonId;
theJournalpostDokumentInfoRelasjonId = this.getJournalpostDokumentInfoRelasjonId();
strategy.appendField(locator, this, "journalpostDokumentInfoRelasjonId", buffer, theJournalpostDokumentInfoRelasjonId);
}
{
DokumentInfo theDokumentInfo;
theDokumentInfo = this.getDokumentInfo();
strategy.appendField(locator, this, "dokumentInfo", buffer, theDokumentInfo);
}
{
TilknyttetJournalpostSom theTilknyttetJournalpostSom;
theTilknyttetJournalpostSom = this.getTilknyttetJournalpostSom();
strategy.appendField(locator, this, "tilknyttetJournalpostSom", buffer, theTilknyttetJournalpostSom);
}
{
String theTilknyttetAvNavn;
theTilknyttetAvNavn = this.getTilknyttetAvNavn();
strategy.appendField(locator, this, "tilknyttetAvNavn", buffer, theTilknyttetAvNavn);
}
{
Sporing theSporing;
theSporing = this.getSporing();
strategy.appendField(locator, this, "sporing", buffer, theSporing);
}
{
String theVersjon;
theVersjon = this.getVersjon();
strategy.appendField(locator, this, "versjon", buffer, theVersjon);
}
{
JournalpostDokumentInfoRelasjonUtvidelse1 theUtvidelse;
theUtvidelse = this.getUtvidelse();
strategy.appendField(locator, this, "utvidelse", buffer, theUtvidelse);
}
return buffer;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy