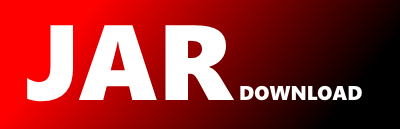
no.nav.gosys.journal.Sporingsdetalj Maven / Gradle / Ivy
package no.nav.gosys.journal;
import java.util.Calendar;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
import org.w3._2001.xmlschema.Adapter1;
/**
* Java class for Sporingsdetalj complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Sporingsdetalj">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="ident" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="identnavn" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="dato" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* <element name="kildenavn" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="utvidelse" type="{http://nav.no/virksomhet/gjennomforing/arkiv/journal/v2}SporingsdetaljUtvidelse1" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Sporingsdetalj", propOrder = {
"ident",
"identnavn",
"dato",
"kildenavn",
"utvidelse"
})
public class Sporingsdetalj
implements Equals, HashCode, ToString
{
protected String ident;
protected String identnavn;
@XmlElement(type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected Calendar dato;
protected String kildenavn;
protected SporingsdetaljUtvidelse1 utvidelse;
/**
* Gets the value of the ident property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIdent() {
return ident;
}
/**
* Sets the value of the ident property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIdent(String value) {
this.ident = value;
}
/**
* Gets the value of the identnavn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIdentnavn() {
return identnavn;
}
/**
* Sets the value of the identnavn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIdentnavn(String value) {
this.identnavn = value;
}
/**
* Gets the value of the dato property.
*
* @return
* possible object is
* {@link String }
*
*/
public Calendar getDato() {
return dato;
}
/**
* Sets the value of the dato property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDato(Calendar value) {
this.dato = value;
}
/**
* Gets the value of the kildenavn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKildenavn() {
return kildenavn;
}
/**
* Sets the value of the kildenavn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKildenavn(String value) {
this.kildenavn = value;
}
/**
* Gets the value of the utvidelse property.
*
* @return
* possible object is
* {@link SporingsdetaljUtvidelse1 }
*
*/
public SporingsdetaljUtvidelse1 getUtvidelse() {
return utvidelse;
}
/**
* Sets the value of the utvidelse property.
*
* @param value
* allowed object is
* {@link SporingsdetaljUtvidelse1 }
*
*/
public void setUtvidelse(SporingsdetaljUtvidelse1 value) {
this.utvidelse = value;
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
String theIdent;
theIdent = this.getIdent();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "ident", theIdent), currentHashCode, theIdent);
}
{
String theIdentnavn;
theIdentnavn = this.getIdentnavn();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "identnavn", theIdentnavn), currentHashCode, theIdentnavn);
}
{
Calendar theDato;
theDato = this.getDato();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "dato", theDato), currentHashCode, theDato);
}
{
String theKildenavn;
theKildenavn = this.getKildenavn();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "kildenavn", theKildenavn), currentHashCode, theKildenavn);
}
{
SporingsdetaljUtvidelse1 theUtvidelse;
theUtvidelse = this.getUtvidelse();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "utvidelse", theUtvidelse), currentHashCode, theUtvidelse);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof Sporingsdetalj)) {
return false;
}
if (this == object) {
return true;
}
final Sporingsdetalj that = ((Sporingsdetalj) object);
{
String lhsIdent;
lhsIdent = this.getIdent();
String rhsIdent;
rhsIdent = that.getIdent();
if (!strategy.equals(LocatorUtils.property(thisLocator, "ident", lhsIdent), LocatorUtils.property(thatLocator, "ident", rhsIdent), lhsIdent, rhsIdent)) {
return false;
}
}
{
String lhsIdentnavn;
lhsIdentnavn = this.getIdentnavn();
String rhsIdentnavn;
rhsIdentnavn = that.getIdentnavn();
if (!strategy.equals(LocatorUtils.property(thisLocator, "identnavn", lhsIdentnavn), LocatorUtils.property(thatLocator, "identnavn", rhsIdentnavn), lhsIdentnavn, rhsIdentnavn)) {
return false;
}
}
{
Calendar lhsDato;
lhsDato = this.getDato();
Calendar rhsDato;
rhsDato = that.getDato();
if (!strategy.equals(LocatorUtils.property(thisLocator, "dato", lhsDato), LocatorUtils.property(thatLocator, "dato", rhsDato), lhsDato, rhsDato)) {
return false;
}
}
{
String lhsKildenavn;
lhsKildenavn = this.getKildenavn();
String rhsKildenavn;
rhsKildenavn = that.getKildenavn();
if (!strategy.equals(LocatorUtils.property(thisLocator, "kildenavn", lhsKildenavn), LocatorUtils.property(thatLocator, "kildenavn", rhsKildenavn), lhsKildenavn, rhsKildenavn)) {
return false;
}
}
{
SporingsdetaljUtvidelse1 lhsUtvidelse;
lhsUtvidelse = this.getUtvidelse();
SporingsdetaljUtvidelse1 rhsUtvidelse;
rhsUtvidelse = that.getUtvidelse();
if (!strategy.equals(LocatorUtils.property(thisLocator, "utvidelse", lhsUtvidelse), LocatorUtils.property(thatLocator, "utvidelse", rhsUtvidelse), lhsUtvidelse, rhsUtvidelse)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theIdent;
theIdent = this.getIdent();
strategy.appendField(locator, this, "ident", buffer, theIdent);
}
{
String theIdentnavn;
theIdentnavn = this.getIdentnavn();
strategy.appendField(locator, this, "identnavn", buffer, theIdentnavn);
}
{
Calendar theDato;
theDato = this.getDato();
strategy.appendField(locator, this, "dato", buffer, theDato);
}
{
String theKildenavn;
theKildenavn = this.getKildenavn();
strategy.appendField(locator, this, "kildenavn", buffer, theKildenavn);
}
{
SporingsdetaljUtvidelse1 theUtvidelse;
theUtvidelse = this.getUtvidelse();
strategy.appendField(locator, this, "utvidelse", buffer, theUtvidelse);
}
return buffer;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy