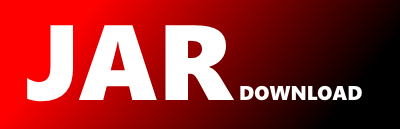
no.nav.tjeneste.virksomhet.behandleinngaaendejournal.v1.ObjectFactory Maven / Gradle / Ivy
package no.nav.tjeneste.virksomhet.behandleinngaaendejournal.v1;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
import no.nav.tjeneste.virksomhet.behandleinngaaendejournal.v1.feil.FerdigstillingIkkeMulig;
import no.nav.tjeneste.virksomhet.behandleinngaaendejournal.v1.feil.JournalpostIkkeInngaeende;
import no.nav.tjeneste.virksomhet.behandleinngaaendejournal.v1.feil.ObjektIkkeFunnet;
import no.nav.tjeneste.virksomhet.behandleinngaaendejournal.v1.feil.OppdateringIkkeMulig;
import no.nav.tjeneste.virksomhet.behandleinngaaendejournal.v1.feil.Sikkerhetsbegrensning;
import no.nav.tjeneste.virksomhet.behandleinngaaendejournal.v1.feil.UgyldigInput;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the no.nav.tjeneste.virksomhet.behandleinngaaendejournal.v1 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _OppdaterJournalpostobjektIkkeFunnet_QNAME = new QName("http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", "oppdaterJournalpostobjektIkkeFunnet");
private final static QName _FerdigstillJournalfoeringobjektIkkeFunnet_QNAME = new QName("http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", "ferdigstillJournalfoeringobjektIkkeFunnet");
private final static QName _OppdaterJournalpostsikkerhetsbegrensning_QNAME = new QName("http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", "oppdaterJournalpostsikkerhetsbegrensning");
private final static QName _FerdigstillJournalfoeringjournalpostIkkeInngaaende_QNAME = new QName("http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", "ferdigstillJournalfoeringjournalpostIkkeInngaaende");
private final static QName _OppdaterJournalpostjournalpostIkkeInngaaende_QNAME = new QName("http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", "oppdaterJournalpostjournalpostIkkeInngaaende");
private final static QName _OppdaterJournalpostoppdateringIkkeMulig_QNAME = new QName("http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", "oppdaterJournalpostoppdateringIkkeMulig");
private final static QName _FerdigstillJournalfoeringferdigstillingIkkeMulig_QNAME = new QName("http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", "ferdigstillJournalfoeringferdigstillingIkkeMulig");
private final static QName _FerdigstillJournalfoeringugyldigInput_QNAME = new QName("http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", "ferdigstillJournalfoeringugyldigInput");
private final static QName _FerdigstillJournalfoeringsikkerhetsbegrensning_QNAME = new QName("http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", "ferdigstillJournalfoeringsikkerhetsbegrensning");
private final static QName _OppdaterJournalpostugyldigInput_QNAME = new QName("http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", "oppdaterJournalpostugyldigInput");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: no.nav.tjeneste.virksomhet.behandleinngaaendejournal.v1
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link FerdigstillJournalfoering }
*
*/
public FerdigstillJournalfoering createFerdigstillJournalfoering() {
return new FerdigstillJournalfoering();
}
/**
* Create an instance of {@link Ping }
*
*/
public Ping createPing() {
return new Ping();
}
/**
* Create an instance of {@link OppdaterJournalpostResponse }
*
*/
public OppdaterJournalpostResponse createOppdaterJournalpostResponse() {
return new OppdaterJournalpostResponse();
}
/**
* Create an instance of {@link OppdaterJournalpost }
*
*/
public OppdaterJournalpost createOppdaterJournalpost() {
return new OppdaterJournalpost();
}
/**
* Create an instance of {@link FerdigstillJournalfoeringResponse }
*
*/
public FerdigstillJournalfoeringResponse createFerdigstillJournalfoeringResponse() {
return new FerdigstillJournalfoeringResponse();
}
/**
* Create an instance of {@link PingResponse }
*
*/
public PingResponse createPingResponse() {
return new PingResponse();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ObjektIkkeFunnet }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", name = "oppdaterJournalpostobjektIkkeFunnet")
public JAXBElement createOppdaterJournalpostobjektIkkeFunnet(ObjektIkkeFunnet value) {
return new JAXBElement(_OppdaterJournalpostobjektIkkeFunnet_QNAME, ObjektIkkeFunnet.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ObjektIkkeFunnet }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", name = "ferdigstillJournalfoeringobjektIkkeFunnet")
public JAXBElement createFerdigstillJournalfoeringobjektIkkeFunnet(ObjektIkkeFunnet value) {
return new JAXBElement(_FerdigstillJournalfoeringobjektIkkeFunnet_QNAME, ObjektIkkeFunnet.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Sikkerhetsbegrensning }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", name = "oppdaterJournalpostsikkerhetsbegrensning")
public JAXBElement createOppdaterJournalpostsikkerhetsbegrensning(Sikkerhetsbegrensning value) {
return new JAXBElement(_OppdaterJournalpostsikkerhetsbegrensning_QNAME, Sikkerhetsbegrensning.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link JournalpostIkkeInngaeende }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", name = "ferdigstillJournalfoeringjournalpostIkkeInngaaende")
public JAXBElement createFerdigstillJournalfoeringjournalpostIkkeInngaaende(JournalpostIkkeInngaeende value) {
return new JAXBElement(_FerdigstillJournalfoeringjournalpostIkkeInngaaende_QNAME, JournalpostIkkeInngaeende.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link JournalpostIkkeInngaeende }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", name = "oppdaterJournalpostjournalpostIkkeInngaaende")
public JAXBElement createOppdaterJournalpostjournalpostIkkeInngaaende(JournalpostIkkeInngaeende value) {
return new JAXBElement(_OppdaterJournalpostjournalpostIkkeInngaaende_QNAME, JournalpostIkkeInngaeende.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link OppdateringIkkeMulig }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", name = "oppdaterJournalpostoppdateringIkkeMulig")
public JAXBElement createOppdaterJournalpostoppdateringIkkeMulig(OppdateringIkkeMulig value) {
return new JAXBElement(_OppdaterJournalpostoppdateringIkkeMulig_QNAME, OppdateringIkkeMulig.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link FerdigstillingIkkeMulig }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", name = "ferdigstillJournalfoeringferdigstillingIkkeMulig")
public JAXBElement createFerdigstillJournalfoeringferdigstillingIkkeMulig(FerdigstillingIkkeMulig value) {
return new JAXBElement(_FerdigstillJournalfoeringferdigstillingIkkeMulig_QNAME, FerdigstillingIkkeMulig.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link UgyldigInput }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", name = "ferdigstillJournalfoeringugyldigInput")
public JAXBElement createFerdigstillJournalfoeringugyldigInput(UgyldigInput value) {
return new JAXBElement(_FerdigstillJournalfoeringugyldigInput_QNAME, UgyldigInput.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Sikkerhetsbegrensning }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", name = "ferdigstillJournalfoeringsikkerhetsbegrensning")
public JAXBElement createFerdigstillJournalfoeringsikkerhetsbegrensning(Sikkerhetsbegrensning value) {
return new JAXBElement(_FerdigstillJournalfoeringsikkerhetsbegrensning_QNAME, Sikkerhetsbegrensning.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link UgyldigInput }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://nav.no/tjeneste/virksomhet/behandleInngaaendeJournal/v1", name = "oppdaterJournalpostugyldigInput")
public JAXBElement createOppdaterJournalpostugyldigInput(UgyldigInput value) {
return new JAXBElement(_OppdaterJournalpostugyldigInput_QNAME, UgyldigInput.class, null, value);
}
}