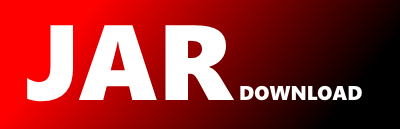
no.nav.tjeneste.virksomhet.sak.v1.informasjon.Sak Maven / Gradle / Ivy
package no.nav.tjeneste.virksomhet.sak.v1.informasjon;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* Saksinformasjon.
*
* Java class for Sak complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Sak">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="sakId" type="{http://nav.no/tjeneste/virksomhet/sak/v1/informasjon}Identifikator"/>
* <element name="sakstype" type="{http://nav.no/tjeneste/virksomhet/sak/v1/informasjon}Sakstyper"/>
* <element name="fagomraade" type="{http://nav.no/tjeneste/virksomhet/sak/v1/informasjon}Fagomraader"/>
* <element name="fagsystem" type="{http://nav.no/tjeneste/virksomhet/sak/v1/informasjon}Fagsystemer"/>
* <element name="fagsystemSakId" type="{http://nav.no/tjeneste/virksomhet/sak/v1/informasjon}Identifikator" minOccurs="0"/>
* <element name="gjelderBrukerListe" type="{http://nav.no/tjeneste/virksomhet/sak/v1/informasjon}Aktoer" maxOccurs="unbounded"/>
* </sequence>
* <attGroup ref="{http://nav.no/tjeneste/virksomhet/sak/v1/metadata}Sporing"/>
* <attGroup ref="{http://nav.no/tjeneste/virksomhet/sak/v1/metadata}Versjon"/>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Sak", propOrder = {
"sakId",
"sakstype",
"fagomraade",
"fagsystem",
"fagsystemSakId",
"gjelderBrukerListe"
})
public class Sak {
@XmlElement(required = true)
protected String sakId;
@XmlElement(required = true)
protected Sakstyper sakstype;
@XmlElement(required = true)
protected Fagomraader fagomraade;
@XmlElement(required = true)
protected Fagsystemer fagsystem;
protected String fagsystemSakId;
@XmlElement(required = true)
protected List gjelderBrukerListe;
@XmlAttribute(name = "endringstidspunkt")
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar endringstidspunkt;
@XmlAttribute(name = "endretAv")
protected String endretAv;
@XmlAttribute(name = "opprettelsetidspunkt")
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar opprettelsetidspunkt;
@XmlAttribute(name = "opprettetAv")
protected String opprettetAv;
@XmlAttribute(name = "versjonsnummer")
protected String versjonsnummer;
@XmlAttribute(name = "versjoneringsdato")
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar versjoneringsdato;
/**
* Gets the value of the sakId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSakId() {
return sakId;
}
/**
* Sets the value of the sakId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSakId(String value) {
this.sakId = value;
}
/**
* Gets the value of the sakstype property.
*
* @return
* possible object is
* {@link Sakstyper }
*
*/
public Sakstyper getSakstype() {
return sakstype;
}
/**
* Sets the value of the sakstype property.
*
* @param value
* allowed object is
* {@link Sakstyper }
*
*/
public void setSakstype(Sakstyper value) {
this.sakstype = value;
}
/**
* Gets the value of the fagomraade property.
*
* @return
* possible object is
* {@link Fagomraader }
*
*/
public Fagomraader getFagomraade() {
return fagomraade;
}
/**
* Sets the value of the fagomraade property.
*
* @param value
* allowed object is
* {@link Fagomraader }
*
*/
public void setFagomraade(Fagomraader value) {
this.fagomraade = value;
}
/**
* Gets the value of the fagsystem property.
*
* @return
* possible object is
* {@link Fagsystemer }
*
*/
public Fagsystemer getFagsystem() {
return fagsystem;
}
/**
* Sets the value of the fagsystem property.
*
* @param value
* allowed object is
* {@link Fagsystemer }
*
*/
public void setFagsystem(Fagsystemer value) {
this.fagsystem = value;
}
/**
* Gets the value of the fagsystemSakId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFagsystemSakId() {
return fagsystemSakId;
}
/**
* Sets the value of the fagsystemSakId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFagsystemSakId(String value) {
this.fagsystemSakId = value;
}
/**
* Gets the value of the gjelderBrukerListe property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the gjelderBrukerListe property.
*
*
* For example, to add a new item, do as follows:
*
* getGjelderBrukerListe().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Aktoer }
*
*
*/
public List getGjelderBrukerListe() {
if (gjelderBrukerListe == null) {
gjelderBrukerListe = new ArrayList();
}
return this.gjelderBrukerListe;
}
/**
* Gets the value of the endringstidspunkt property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getEndringstidspunkt() {
return endringstidspunkt;
}
/**
* Sets the value of the endringstidspunkt property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setEndringstidspunkt(XMLGregorianCalendar value) {
this.endringstidspunkt = value;
}
/**
* Gets the value of the endretAv property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEndretAv() {
return endretAv;
}
/**
* Sets the value of the endretAv property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEndretAv(String value) {
this.endretAv = value;
}
/**
* Gets the value of the opprettelsetidspunkt property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getOpprettelsetidspunkt() {
return opprettelsetidspunkt;
}
/**
* Sets the value of the opprettelsetidspunkt property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setOpprettelsetidspunkt(XMLGregorianCalendar value) {
this.opprettelsetidspunkt = value;
}
/**
* Gets the value of the opprettetAv property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOpprettetAv() {
return opprettetAv;
}
/**
* Sets the value of the opprettetAv property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOpprettetAv(String value) {
this.opprettetAv = value;
}
/**
* Gets the value of the versjonsnummer property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVersjonsnummer() {
return versjonsnummer;
}
/**
* Sets the value of the versjonsnummer property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVersjonsnummer(String value) {
this.versjonsnummer = value;
}
/**
* Gets the value of the versjoneringsdato property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getVersjoneringsdato() {
return versjoneringsdato;
}
/**
* Sets the value of the versjoneringsdato property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setVersjoneringsdato(XMLGregorianCalendar value) {
this.versjoneringsdato = value;
}
}