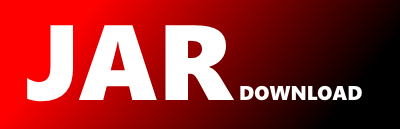
no.nav.tjeneste.virksomhet.notat.v1.informasjon.Notat Maven / Gradle / Ivy
package no.nav.tjeneste.virksomhet.notat.v1.informasjon;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for Notat complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Notat">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="personident" type="{http://nav.no/tjeneste/virksomhet/notat/v1/informasjon}Identifikator"/>
* <element name="sakstype" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="tittel" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="beskrivelse" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="saksnr" type="{http://nav.no/tjeneste/virksomhet/notat/v1/informasjon}Identifikator" minOccurs="0"/>
* <element name="opprinnelse" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="kontekst" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="tiltaksvariant" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="erSensitivt" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="endringsinformasjon" type="{http://nav.no/tjeneste/virksomhet/notat/v1/informasjon}Endringsinformasjon"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Notat", propOrder = {
"personident",
"sakstype",
"tittel",
"beskrivelse",
"saksnr",
"opprinnelse",
"kontekst",
"tiltaksvariant",
"erSensitivt",
"endringsinformasjon"
})
public class Notat {
@XmlElement(required = true)
protected String personident;
protected String sakstype;
@XmlElement(required = true)
protected String tittel;
@XmlElement(required = true)
protected String beskrivelse;
protected String saksnr;
@XmlElement(required = true)
protected String opprinnelse;
protected String kontekst;
protected String tiltaksvariant;
protected boolean erSensitivt;
@XmlElement(required = true)
protected Endringsinformasjon endringsinformasjon;
/**
* Gets the value of the personident property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPersonident() {
return personident;
}
/**
* Sets the value of the personident property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPersonident(String value) {
this.personident = value;
}
/**
* Gets the value of the sakstype property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSakstype() {
return sakstype;
}
/**
* Sets the value of the sakstype property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSakstype(String value) {
this.sakstype = value;
}
/**
* Gets the value of the tittel property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTittel() {
return tittel;
}
/**
* Sets the value of the tittel property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTittel(String value) {
this.tittel = value;
}
/**
* Gets the value of the beskrivelse property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBeskrivelse() {
return beskrivelse;
}
/**
* Sets the value of the beskrivelse property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBeskrivelse(String value) {
this.beskrivelse = value;
}
/**
* Gets the value of the saksnr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSaksnr() {
return saksnr;
}
/**
* Sets the value of the saksnr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSaksnr(String value) {
this.saksnr = value;
}
/**
* Gets the value of the opprinnelse property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOpprinnelse() {
return opprinnelse;
}
/**
* Sets the value of the opprinnelse property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOpprinnelse(String value) {
this.opprinnelse = value;
}
/**
* Gets the value of the kontekst property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getKontekst() {
return kontekst;
}
/**
* Sets the value of the kontekst property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setKontekst(String value) {
this.kontekst = value;
}
/**
* Gets the value of the tiltaksvariant property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTiltaksvariant() {
return tiltaksvariant;
}
/**
* Sets the value of the tiltaksvariant property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTiltaksvariant(String value) {
this.tiltaksvariant = value;
}
/**
* Gets the value of the erSensitivt property.
*
*/
public boolean isErSensitivt() {
return erSensitivt;
}
/**
* Sets the value of the erSensitivt property.
*
*/
public void setErSensitivt(boolean value) {
this.erSensitivt = value;
}
/**
* Gets the value of the endringsinformasjon property.
*
* @return
* possible object is
* {@link Endringsinformasjon }
*
*/
public Endringsinformasjon getEndringsinformasjon() {
return endringsinformasjon;
}
/**
* Sets the value of the endringsinformasjon property.
*
* @param value
* allowed object is
* {@link Endringsinformasjon }
*
*/
public void setEndringsinformasjon(Endringsinformasjon value) {
this.endringsinformasjon = value;
}
public Notat withPersonident(String value) {
setPersonident(value);
return this;
}
public Notat withSakstype(String value) {
setSakstype(value);
return this;
}
public Notat withTittel(String value) {
setTittel(value);
return this;
}
public Notat withBeskrivelse(String value) {
setBeskrivelse(value);
return this;
}
public Notat withSaksnr(String value) {
setSaksnr(value);
return this;
}
public Notat withOpprinnelse(String value) {
setOpprinnelse(value);
return this;
}
public Notat withKontekst(String value) {
setKontekst(value);
return this;
}
public Notat withTiltaksvariant(String value) {
setTiltaksvariant(value);
return this;
}
public Notat withErSensitivt(boolean value) {
setErSensitivt(value);
return this;
}
public Notat withEndringsinformasjon(Endringsinformasjon value) {
setEndringsinformasjon(value);
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy