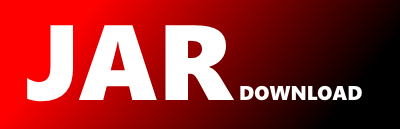
no.nav.gosys.pensjonsak.Sak Maven / Gradle / Ivy
package no.nav.gosys.pensjonsak;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
* Java class for Sak complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Sak">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="sakId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="fnr" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="sakstype" type="{http://nav.no/virksomhet/gjennomforing/sak/pensjon/v1}Sakstype" minOccurs="0"/>
* <element name="saksstatus" type="{http://nav.no/virksomhet/gjennomforing/sak/pensjon/v1}Saksstatus" minOccurs="0"/>
* <element name="ansvarligEnhetId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="sporing" type="{http://nav.no/virksomhet/gjennomforing/sak/pensjon/v1}Sporing" minOccurs="0"/>
* <element name="inhabil" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="utvidelse" type="{http://nav.no/virksomhet/gjennomforing/sak/pensjon/v1}SakUtvidelse1" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Sak", propOrder = {
"sakId",
"fnr",
"sakstype",
"saksstatus",
"ansvarligEnhetId",
"sporing",
"inhabil",
"utvidelse"
})
public class Sak
implements Equals, HashCode, ToString
{
protected String sakId;
protected String fnr;
protected Sakstype sakstype;
protected Saksstatus saksstatus;
protected String ansvarligEnhetId;
protected Sporing sporing;
protected Boolean inhabil;
protected SakUtvidelse1 utvidelse;
/**
* Gets the value of the sakId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSakId() {
return sakId;
}
/**
* Sets the value of the sakId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSakId(String value) {
this.sakId = value;
}
/**
* Gets the value of the fnr property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFnr() {
return fnr;
}
/**
* Sets the value of the fnr property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFnr(String value) {
this.fnr = value;
}
/**
* Gets the value of the sakstype property.
*
* @return
* possible object is
* {@link Sakstype }
*
*/
public Sakstype getSakstype() {
return sakstype;
}
/**
* Sets the value of the sakstype property.
*
* @param value
* allowed object is
* {@link Sakstype }
*
*/
public void setSakstype(Sakstype value) {
this.sakstype = value;
}
/**
* Gets the value of the saksstatus property.
*
* @return
* possible object is
* {@link Saksstatus }
*
*/
public Saksstatus getSaksstatus() {
return saksstatus;
}
/**
* Sets the value of the saksstatus property.
*
* @param value
* allowed object is
* {@link Saksstatus }
*
*/
public void setSaksstatus(Saksstatus value) {
this.saksstatus = value;
}
/**
* Gets the value of the ansvarligEnhetId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAnsvarligEnhetId() {
return ansvarligEnhetId;
}
/**
* Sets the value of the ansvarligEnhetId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAnsvarligEnhetId(String value) {
this.ansvarligEnhetId = value;
}
/**
* Gets the value of the sporing property.
*
* @return
* possible object is
* {@link Sporing }
*
*/
public Sporing getSporing() {
return sporing;
}
/**
* Sets the value of the sporing property.
*
* @param value
* allowed object is
* {@link Sporing }
*
*/
public void setSporing(Sporing value) {
this.sporing = value;
}
/**
* Gets the value of the inhabil property.
* This getter has been renamed from isInhabil() to getInhabil() by cxf-xjc-boolean plugin.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean getInhabil() {
return inhabil;
}
/**
* Sets the value of the inhabil property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setInhabil(Boolean value) {
this.inhabil = value;
}
/**
* Gets the value of the utvidelse property.
*
* @return
* possible object is
* {@link SakUtvidelse1 }
*
*/
public SakUtvidelse1 getUtvidelse() {
return utvidelse;
}
/**
* Sets the value of the utvidelse property.
*
* @param value
* allowed object is
* {@link SakUtvidelse1 }
*
*/
public void setUtvidelse(SakUtvidelse1 value) {
this.utvidelse = value;
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
String theSakId;
theSakId = this.getSakId();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "sakId", theSakId), currentHashCode, theSakId);
}
{
String theFnr;
theFnr = this.getFnr();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "fnr", theFnr), currentHashCode, theFnr);
}
{
Sakstype theSakstype;
theSakstype = this.getSakstype();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "sakstype", theSakstype), currentHashCode, theSakstype);
}
{
Saksstatus theSaksstatus;
theSaksstatus = this.getSaksstatus();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "saksstatus", theSaksstatus), currentHashCode, theSaksstatus);
}
{
String theAnsvarligEnhetId;
theAnsvarligEnhetId = this.getAnsvarligEnhetId();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "ansvarligEnhetId", theAnsvarligEnhetId), currentHashCode, theAnsvarligEnhetId);
}
{
Sporing theSporing;
theSporing = this.getSporing();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "sporing", theSporing), currentHashCode, theSporing);
}
{
Boolean theInhabil;
theInhabil = this.getInhabil();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "inhabil", theInhabil), currentHashCode, theInhabil);
}
{
SakUtvidelse1 theUtvidelse;
theUtvidelse = this.getUtvidelse();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "utvidelse", theUtvidelse), currentHashCode, theUtvidelse);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof Sak)) {
return false;
}
if (this == object) {
return true;
}
final Sak that = ((Sak) object);
{
String lhsSakId;
lhsSakId = this.getSakId();
String rhsSakId;
rhsSakId = that.getSakId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "sakId", lhsSakId), LocatorUtils.property(thatLocator, "sakId", rhsSakId), lhsSakId, rhsSakId)) {
return false;
}
}
{
String lhsFnr;
lhsFnr = this.getFnr();
String rhsFnr;
rhsFnr = that.getFnr();
if (!strategy.equals(LocatorUtils.property(thisLocator, "fnr", lhsFnr), LocatorUtils.property(thatLocator, "fnr", rhsFnr), lhsFnr, rhsFnr)) {
return false;
}
}
{
Sakstype lhsSakstype;
lhsSakstype = this.getSakstype();
Sakstype rhsSakstype;
rhsSakstype = that.getSakstype();
if (!strategy.equals(LocatorUtils.property(thisLocator, "sakstype", lhsSakstype), LocatorUtils.property(thatLocator, "sakstype", rhsSakstype), lhsSakstype, rhsSakstype)) {
return false;
}
}
{
Saksstatus lhsSaksstatus;
lhsSaksstatus = this.getSaksstatus();
Saksstatus rhsSaksstatus;
rhsSaksstatus = that.getSaksstatus();
if (!strategy.equals(LocatorUtils.property(thisLocator, "saksstatus", lhsSaksstatus), LocatorUtils.property(thatLocator, "saksstatus", rhsSaksstatus), lhsSaksstatus, rhsSaksstatus)) {
return false;
}
}
{
String lhsAnsvarligEnhetId;
lhsAnsvarligEnhetId = this.getAnsvarligEnhetId();
String rhsAnsvarligEnhetId;
rhsAnsvarligEnhetId = that.getAnsvarligEnhetId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "ansvarligEnhetId", lhsAnsvarligEnhetId), LocatorUtils.property(thatLocator, "ansvarligEnhetId", rhsAnsvarligEnhetId), lhsAnsvarligEnhetId, rhsAnsvarligEnhetId)) {
return false;
}
}
{
Sporing lhsSporing;
lhsSporing = this.getSporing();
Sporing rhsSporing;
rhsSporing = that.getSporing();
if (!strategy.equals(LocatorUtils.property(thisLocator, "sporing", lhsSporing), LocatorUtils.property(thatLocator, "sporing", rhsSporing), lhsSporing, rhsSporing)) {
return false;
}
}
{
Boolean lhsInhabil;
lhsInhabil = this.getInhabil();
Boolean rhsInhabil;
rhsInhabil = that.getInhabil();
if (!strategy.equals(LocatorUtils.property(thisLocator, "inhabil", lhsInhabil), LocatorUtils.property(thatLocator, "inhabil", rhsInhabil), lhsInhabil, rhsInhabil)) {
return false;
}
}
{
SakUtvidelse1 lhsUtvidelse;
lhsUtvidelse = this.getUtvidelse();
SakUtvidelse1 rhsUtvidelse;
rhsUtvidelse = that.getUtvidelse();
if (!strategy.equals(LocatorUtils.property(thisLocator, "utvidelse", lhsUtvidelse), LocatorUtils.property(thatLocator, "utvidelse", rhsUtvidelse), lhsUtvidelse, rhsUtvidelse)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theSakId;
theSakId = this.getSakId();
strategy.appendField(locator, this, "sakId", buffer, theSakId);
}
{
String theFnr;
theFnr = this.getFnr();
strategy.appendField(locator, this, "fnr", buffer, theFnr);
}
{
Sakstype theSakstype;
theSakstype = this.getSakstype();
strategy.appendField(locator, this, "sakstype", buffer, theSakstype);
}
{
Saksstatus theSaksstatus;
theSaksstatus = this.getSaksstatus();
strategy.appendField(locator, this, "saksstatus", buffer, theSaksstatus);
}
{
String theAnsvarligEnhetId;
theAnsvarligEnhetId = this.getAnsvarligEnhetId();
strategy.appendField(locator, this, "ansvarligEnhetId", buffer, theAnsvarligEnhetId);
}
{
Sporing theSporing;
theSporing = this.getSporing();
strategy.appendField(locator, this, "sporing", buffer, theSporing);
}
{
Boolean theInhabil;
theInhabil = this.getInhabil();
strategy.appendField(locator, this, "inhabil", buffer, theInhabil);
}
{
SakUtvidelse1 theUtvidelse;
theUtvidelse = this.getUtvidelse();
strategy.appendField(locator, this, "utvidelse", buffer, theUtvidelse);
}
return buffer;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy