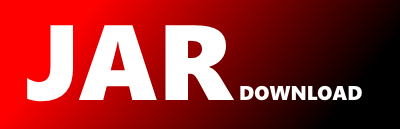
no.nav.sbl.soknadsosialhjelp.soknad.okonomi.opplysning.JsonOkonomiOpplysningUtbetalingKomponent Maven / Gradle / Ivy
package no.nav.sbl.soknadsosialhjelp.soknad.okonomi.opplysning;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"type",
"belop",
"satsType",
"satsAntall",
"satsBelop"
})
public class JsonOkonomiOpplysningUtbetalingKomponent {
/**
* Beskrivelse av hva slags type delutbetaling det er. Eksempler: "Arbeidstaker", "Grunnpensjon" og "Tilleggspensjon".
*
*/
@JsonProperty("type")
@JsonPropertyDescription("Beskrivelse av hva slags type delutbetaling det er. Eksempler: \"Arbeidstaker\", \"Grunnpensjon\" og \"Tilleggspensjon\".")
private String type;
/**
* Beløp for delutbetalingen. Resultat av satsType, satsAntall og satsBelop
*
*/
@JsonProperty("belop")
@JsonPropertyDescription("Bel\u00f8p for delutbetalingen. Resultat av satsType, satsAntall og satsBelop")
private Double belop;
/**
* Beskrivelse av hva slags type sats det er. Eksempel: "Dag" og "Prosent".
*
*/
@JsonProperty("satsType")
@JsonPropertyDescription("Beskrivelse av hva slags type sats det er. Eksempel: \"Dag\" og \"Prosent\".")
private String satsType;
/**
* Antall enheter av satstypen for delutbetalingen.
*
*/
@JsonProperty("satsAntall")
@JsonPropertyDescription("Antall enheter av satstypen for delutbetalingen.")
private Double satsAntall;
/**
* Satsbeløpet for delutbetalingen
*
*/
@JsonProperty("satsBelop")
@JsonPropertyDescription("Satsbel\u00f8pet for delutbetalingen")
private Double satsBelop;
@JsonIgnore
private Map additionalProperties = new HashMap();
/**
* Beskrivelse av hva slags type delutbetaling det er. Eksempler: "Arbeidstaker", "Grunnpensjon" og "Tilleggspensjon".
*
*/
@JsonProperty("type")
public String getType() {
return type;
}
/**
* Beskrivelse av hva slags type delutbetaling det er. Eksempler: "Arbeidstaker", "Grunnpensjon" og "Tilleggspensjon".
*
*/
@JsonProperty("type")
public void setType(String type) {
this.type = type;
}
public JsonOkonomiOpplysningUtbetalingKomponent withType(String type) {
this.type = type;
return this;
}
/**
* Beløp for delutbetalingen. Resultat av satsType, satsAntall og satsBelop
*
*/
@JsonProperty("belop")
public Double getBelop() {
return belop;
}
/**
* Beløp for delutbetalingen. Resultat av satsType, satsAntall og satsBelop
*
*/
@JsonProperty("belop")
public void setBelop(Double belop) {
this.belop = belop;
}
public JsonOkonomiOpplysningUtbetalingKomponent withBelop(Double belop) {
this.belop = belop;
return this;
}
/**
* Beskrivelse av hva slags type sats det er. Eksempel: "Dag" og "Prosent".
*
*/
@JsonProperty("satsType")
public String getSatsType() {
return satsType;
}
/**
* Beskrivelse av hva slags type sats det er. Eksempel: "Dag" og "Prosent".
*
*/
@JsonProperty("satsType")
public void setSatsType(String satsType) {
this.satsType = satsType;
}
public JsonOkonomiOpplysningUtbetalingKomponent withSatsType(String satsType) {
this.satsType = satsType;
return this;
}
/**
* Antall enheter av satstypen for delutbetalingen.
*
*/
@JsonProperty("satsAntall")
public Double getSatsAntall() {
return satsAntall;
}
/**
* Antall enheter av satstypen for delutbetalingen.
*
*/
@JsonProperty("satsAntall")
public void setSatsAntall(Double satsAntall) {
this.satsAntall = satsAntall;
}
public JsonOkonomiOpplysningUtbetalingKomponent withSatsAntall(Double satsAntall) {
this.satsAntall = satsAntall;
return this;
}
/**
* Satsbeløpet for delutbetalingen
*
*/
@JsonProperty("satsBelop")
public Double getSatsBelop() {
return satsBelop;
}
/**
* Satsbeløpet for delutbetalingen
*
*/
@JsonProperty("satsBelop")
public void setSatsBelop(Double satsBelop) {
this.satsBelop = satsBelop;
}
public JsonOkonomiOpplysningUtbetalingKomponent withSatsBelop(Double satsBelop) {
this.satsBelop = satsBelop;
return this;
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
public JsonOkonomiOpplysningUtbetalingKomponent withAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
return this;
}
@Override
public String toString() {
return new ToStringBuilder(this).append("type", type).append("belop", belop).append("satsType", satsType).append("satsAntall", satsAntall).append("satsBelop", satsBelop).append("additionalProperties", additionalProperties).toString();
}
@Override
public int hashCode() {
return new HashCodeBuilder().append(satsType).append(satsAntall).append(additionalProperties).append(type).append(satsBelop).append(belop).toHashCode();
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof JsonOkonomiOpplysningUtbetalingKomponent) == false) {
return false;
}
JsonOkonomiOpplysningUtbetalingKomponent rhs = ((JsonOkonomiOpplysningUtbetalingKomponent) other);
return new EqualsBuilder().append(satsType, rhs.satsType).append(satsAntall, rhs.satsAntall).append(additionalProperties, rhs.additionalProperties).append(type, rhs.type).append(satsBelop, rhs.satsBelop).append(belop, rhs.belop).isEquals();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy