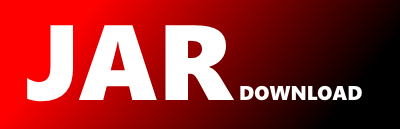
no.udi.common_tilgangskontroll.v1.Rettighet Maven / Gradle / Ivy
package no.udi.common_tilgangskontroll.v1;
import java.io.Serializable;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
* Beskrivelse av en rettighet for en bruker
*
* Java class for Rettighet complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Rettighet">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="ReferanseNokkel" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="Visningsnavn" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="OpprinneligVisningsnavn" type="{http://www.w3.org/2001/XMLSchema}string"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Rettighet", propOrder = {
"referanseNokkel",
"visningsnavn",
"opprinneligVisningsnavn"
})
public class Rettighet
implements Serializable, Equals, HashCode
{
@XmlElement(name = "ReferanseNokkel")
protected String referanseNokkel;
@XmlElement(name = "Visningsnavn", required = true)
protected String visningsnavn;
@XmlElement(name = "OpprinneligVisningsnavn", required = true)
protected String opprinneligVisningsnavn;
/**
* Gets the value of the referanseNokkel property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReferanseNokkel() {
return referanseNokkel;
}
/**
* Sets the value of the referanseNokkel property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setReferanseNokkel(String value) {
this.referanseNokkel = value;
}
/**
* Gets the value of the visningsnavn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVisningsnavn() {
return visningsnavn;
}
/**
* Sets the value of the visningsnavn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVisningsnavn(String value) {
this.visningsnavn = value;
}
/**
* Gets the value of the opprinneligVisningsnavn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOpprinneligVisningsnavn() {
return opprinneligVisningsnavn;
}
/**
* Sets the value of the opprinneligVisningsnavn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOpprinneligVisningsnavn(String value) {
this.opprinneligVisningsnavn = value;
}
public Rettighet withReferanseNokkel(String value) {
setReferanseNokkel(value);
return this;
}
public Rettighet withVisningsnavn(String value) {
setVisningsnavn(value);
return this;
}
public Rettighet withOpprinneligVisningsnavn(String value) {
setOpprinneligVisningsnavn(value);
return this;
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
String theReferanseNokkel;
theReferanseNokkel = this.getReferanseNokkel();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "referanseNokkel", theReferanseNokkel), currentHashCode, theReferanseNokkel);
}
{
String theVisningsnavn;
theVisningsnavn = this.getVisningsnavn();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "visningsnavn", theVisningsnavn), currentHashCode, theVisningsnavn);
}
{
String theOpprinneligVisningsnavn;
theOpprinneligVisningsnavn = this.getOpprinneligVisningsnavn();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "opprinneligVisningsnavn", theOpprinneligVisningsnavn), currentHashCode, theOpprinneligVisningsnavn);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof Rettighet)) {
return false;
}
if (this == object) {
return true;
}
final Rettighet that = ((Rettighet) object);
{
String lhsReferanseNokkel;
lhsReferanseNokkel = this.getReferanseNokkel();
String rhsReferanseNokkel;
rhsReferanseNokkel = that.getReferanseNokkel();
if (!strategy.equals(LocatorUtils.property(thisLocator, "referanseNokkel", lhsReferanseNokkel), LocatorUtils.property(thatLocator, "referanseNokkel", rhsReferanseNokkel), lhsReferanseNokkel, rhsReferanseNokkel)) {
return false;
}
}
{
String lhsVisningsnavn;
lhsVisningsnavn = this.getVisningsnavn();
String rhsVisningsnavn;
rhsVisningsnavn = that.getVisningsnavn();
if (!strategy.equals(LocatorUtils.property(thisLocator, "visningsnavn", lhsVisningsnavn), LocatorUtils.property(thatLocator, "visningsnavn", rhsVisningsnavn), lhsVisningsnavn, rhsVisningsnavn)) {
return false;
}
}
{
String lhsOpprinneligVisningsnavn;
lhsOpprinneligVisningsnavn = this.getOpprinneligVisningsnavn();
String rhsOpprinneligVisningsnavn;
rhsOpprinneligVisningsnavn = that.getOpprinneligVisningsnavn();
if (!strategy.equals(LocatorUtils.property(thisLocator, "opprinneligVisningsnavn", lhsOpprinneligVisningsnavn), LocatorUtils.property(thatLocator, "opprinneligVisningsnavn", rhsOpprinneligVisningsnavn), lhsOpprinneligVisningsnavn, rhsOpprinneligVisningsnavn)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy