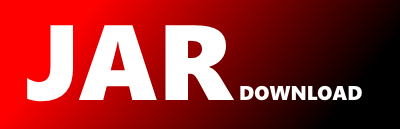
no.udi.mt_1067_nav_data.v1.Avgjorelse Maven / Gradle / Ivy
package no.udi.mt_1067_nav_data.v1;
import java.io.Serializable;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
* Java class for Avgjorelse complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Avgjorelse">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AvgjorelseId" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="OmgjortavAvgjorelseId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="Avgjorelsestype" type="{http://udi.no/MT_1067_NAV_Data/v1}Avgjorelsestype" minOccurs="0"/>
* <element name="ErPositiv" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="Tillatelse" type="{http://udi.no/MT_1067_NAV_Data/v1}Tillatelse" minOccurs="0"/>
* <element name="Utfall" type="{http://udi.no/MT_1067_NAV_Data/v1}Utfall" minOccurs="0"/>
* <element name="AvgjorelseDato" type="{http://www.w3.org/2001/XMLSchema}dateTime"/>
* <element name="EffektueringsDato" type="{http://www.w3.org/2001/XMLSchema}dateTime"/>
* <element name="IverksettelseDato" type="{http://www.w3.org/2001/XMLSchema}dateTime"/>
* <element name="UtreisefristDato" type="{http://www.w3.org/2001/XMLSchema}dateTime"/>
* <element name="Saksnummer" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="Etat" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="Flyktingstatus" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* <element name="UavklartFlyktningstatus" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Avgjorelse", propOrder = {
"avgjorelseId",
"omgjortavAvgjorelseId",
"avgjorelsestype",
"erPositiv",
"tillatelse",
"utfall",
"avgjorelseDato",
"effektueringsDato",
"iverksettelseDato",
"utreisefristDato",
"saksnummer",
"etat",
"flyktingstatus",
"uavklartFlyktningstatus"
})
public class Avgjorelse
implements Serializable, Equals, HashCode
{
@XmlElement(name = "AvgjorelseId", required = true)
protected String avgjorelseId;
@XmlElement(name = "OmgjortavAvgjorelseId")
protected String omgjortavAvgjorelseId;
@XmlElement(name = "Avgjorelsestype")
protected Avgjorelsestype avgjorelsestype;
@XmlElement(name = "ErPositiv")
protected boolean erPositiv;
@XmlElement(name = "Tillatelse")
protected Tillatelse tillatelse;
@XmlElement(name = "Utfall")
protected Utfall utfall;
@XmlElement(name = "AvgjorelseDato", required = true, nillable = true)
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar avgjorelseDato;
@XmlElement(name = "EffektueringsDato", required = true, nillable = true)
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar effektueringsDato;
@XmlElement(name = "IverksettelseDato", required = true, nillable = true)
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar iverksettelseDato;
@XmlElement(name = "UtreisefristDato", required = true, nillable = true)
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar utreisefristDato;
@XmlElement(name = "Saksnummer", required = true)
protected String saksnummer;
@XmlElement(name = "Etat")
protected String etat;
@XmlElement(name = "Flyktingstatus", required = true, type = Boolean.class, nillable = true)
protected Boolean flyktingstatus;
@XmlElement(name = "UavklartFlyktningstatus", required = true, type = Boolean.class, nillable = true)
protected Boolean uavklartFlyktningstatus;
/**
* Gets the value of the avgjorelseId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAvgjorelseId() {
return avgjorelseId;
}
/**
* Sets the value of the avgjorelseId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAvgjorelseId(String value) {
this.avgjorelseId = value;
}
/**
* Gets the value of the omgjortavAvgjorelseId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOmgjortavAvgjorelseId() {
return omgjortavAvgjorelseId;
}
/**
* Sets the value of the omgjortavAvgjorelseId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOmgjortavAvgjorelseId(String value) {
this.omgjortavAvgjorelseId = value;
}
/**
* Gets the value of the avgjorelsestype property.
*
* @return
* possible object is
* {@link Avgjorelsestype }
*
*/
public Avgjorelsestype getAvgjorelsestype() {
return avgjorelsestype;
}
/**
* Sets the value of the avgjorelsestype property.
*
* @param value
* allowed object is
* {@link Avgjorelsestype }
*
*/
public void setAvgjorelsestype(Avgjorelsestype value) {
this.avgjorelsestype = value;
}
/**
* Gets the value of the erPositiv property.
*
*/
public boolean isErPositiv() {
return erPositiv;
}
/**
* Sets the value of the erPositiv property.
*
*/
public void setErPositiv(boolean value) {
this.erPositiv = value;
}
/**
* Gets the value of the tillatelse property.
*
* @return
* possible object is
* {@link Tillatelse }
*
*/
public Tillatelse getTillatelse() {
return tillatelse;
}
/**
* Sets the value of the tillatelse property.
*
* @param value
* allowed object is
* {@link Tillatelse }
*
*/
public void setTillatelse(Tillatelse value) {
this.tillatelse = value;
}
/**
* Gets the value of the utfall property.
*
* @return
* possible object is
* {@link Utfall }
*
*/
public Utfall getUtfall() {
return utfall;
}
/**
* Sets the value of the utfall property.
*
* @param value
* allowed object is
* {@link Utfall }
*
*/
public void setUtfall(Utfall value) {
this.utfall = value;
}
/**
* Gets the value of the avgjorelseDato property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getAvgjorelseDato() {
return avgjorelseDato;
}
/**
* Sets the value of the avgjorelseDato property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setAvgjorelseDato(XMLGregorianCalendar value) {
this.avgjorelseDato = value;
}
/**
* Gets the value of the effektueringsDato property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getEffektueringsDato() {
return effektueringsDato;
}
/**
* Sets the value of the effektueringsDato property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setEffektueringsDato(XMLGregorianCalendar value) {
this.effektueringsDato = value;
}
/**
* Gets the value of the iverksettelseDato property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getIverksettelseDato() {
return iverksettelseDato;
}
/**
* Sets the value of the iverksettelseDato property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setIverksettelseDato(XMLGregorianCalendar value) {
this.iverksettelseDato = value;
}
/**
* Gets the value of the utreisefristDato property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getUtreisefristDato() {
return utreisefristDato;
}
/**
* Sets the value of the utreisefristDato property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setUtreisefristDato(XMLGregorianCalendar value) {
this.utreisefristDato = value;
}
/**
* Gets the value of the saksnummer property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSaksnummer() {
return saksnummer;
}
/**
* Sets the value of the saksnummer property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSaksnummer(String value) {
this.saksnummer = value;
}
/**
* Gets the value of the etat property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEtat() {
return etat;
}
/**
* Sets the value of the etat property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEtat(String value) {
this.etat = value;
}
/**
* Gets the value of the flyktingstatus property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isFlyktingstatus() {
return flyktingstatus;
}
/**
* Sets the value of the flyktingstatus property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setFlyktingstatus(Boolean value) {
this.flyktingstatus = value;
}
/**
* Gets the value of the uavklartFlyktningstatus property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isUavklartFlyktningstatus() {
return uavklartFlyktningstatus;
}
/**
* Sets the value of the uavklartFlyktningstatus property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setUavklartFlyktningstatus(Boolean value) {
this.uavklartFlyktningstatus = value;
}
public Avgjorelse withAvgjorelseId(String value) {
setAvgjorelseId(value);
return this;
}
public Avgjorelse withOmgjortavAvgjorelseId(String value) {
setOmgjortavAvgjorelseId(value);
return this;
}
public Avgjorelse withAvgjorelsestype(Avgjorelsestype value) {
setAvgjorelsestype(value);
return this;
}
public Avgjorelse withErPositiv(boolean value) {
setErPositiv(value);
return this;
}
public Avgjorelse withTillatelse(Tillatelse value) {
setTillatelse(value);
return this;
}
public Avgjorelse withUtfall(Utfall value) {
setUtfall(value);
return this;
}
public Avgjorelse withAvgjorelseDato(XMLGregorianCalendar value) {
setAvgjorelseDato(value);
return this;
}
public Avgjorelse withEffektueringsDato(XMLGregorianCalendar value) {
setEffektueringsDato(value);
return this;
}
public Avgjorelse withIverksettelseDato(XMLGregorianCalendar value) {
setIverksettelseDato(value);
return this;
}
public Avgjorelse withUtreisefristDato(XMLGregorianCalendar value) {
setUtreisefristDato(value);
return this;
}
public Avgjorelse withSaksnummer(String value) {
setSaksnummer(value);
return this;
}
public Avgjorelse withEtat(String value) {
setEtat(value);
return this;
}
public Avgjorelse withFlyktingstatus(Boolean value) {
setFlyktingstatus(value);
return this;
}
public Avgjorelse withUavklartFlyktningstatus(Boolean value) {
setUavklartFlyktningstatus(value);
return this;
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
String theAvgjorelseId;
theAvgjorelseId = this.getAvgjorelseId();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "avgjorelseId", theAvgjorelseId), currentHashCode, theAvgjorelseId);
}
{
String theOmgjortavAvgjorelseId;
theOmgjortavAvgjorelseId = this.getOmgjortavAvgjorelseId();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "omgjortavAvgjorelseId", theOmgjortavAvgjorelseId), currentHashCode, theOmgjortavAvgjorelseId);
}
{
Avgjorelsestype theAvgjorelsestype;
theAvgjorelsestype = this.getAvgjorelsestype();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "avgjorelsestype", theAvgjorelsestype), currentHashCode, theAvgjorelsestype);
}
{
boolean theErPositiv;
theErPositiv = (true?this.isErPositiv():false);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "erPositiv", theErPositiv), currentHashCode, theErPositiv);
}
{
Tillatelse theTillatelse;
theTillatelse = this.getTillatelse();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "tillatelse", theTillatelse), currentHashCode, theTillatelse);
}
{
Utfall theUtfall;
theUtfall = this.getUtfall();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "utfall", theUtfall), currentHashCode, theUtfall);
}
{
XMLGregorianCalendar theAvgjorelseDato;
theAvgjorelseDato = this.getAvgjorelseDato();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "avgjorelseDato", theAvgjorelseDato), currentHashCode, theAvgjorelseDato);
}
{
XMLGregorianCalendar theEffektueringsDato;
theEffektueringsDato = this.getEffektueringsDato();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "effektueringsDato", theEffektueringsDato), currentHashCode, theEffektueringsDato);
}
{
XMLGregorianCalendar theIverksettelseDato;
theIverksettelseDato = this.getIverksettelseDato();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "iverksettelseDato", theIverksettelseDato), currentHashCode, theIverksettelseDato);
}
{
XMLGregorianCalendar theUtreisefristDato;
theUtreisefristDato = this.getUtreisefristDato();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "utreisefristDato", theUtreisefristDato), currentHashCode, theUtreisefristDato);
}
{
String theSaksnummer;
theSaksnummer = this.getSaksnummer();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "saksnummer", theSaksnummer), currentHashCode, theSaksnummer);
}
{
String theEtat;
theEtat = this.getEtat();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "etat", theEtat), currentHashCode, theEtat);
}
{
Boolean theFlyktingstatus;
theFlyktingstatus = this.isFlyktingstatus();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "flyktingstatus", theFlyktingstatus), currentHashCode, theFlyktingstatus);
}
{
Boolean theUavklartFlyktningstatus;
theUavklartFlyktningstatus = this.isUavklartFlyktningstatus();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "uavklartFlyktningstatus", theUavklartFlyktningstatus), currentHashCode, theUavklartFlyktningstatus);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof Avgjorelse)) {
return false;
}
if (this == object) {
return true;
}
final Avgjorelse that = ((Avgjorelse) object);
{
String lhsAvgjorelseId;
lhsAvgjorelseId = this.getAvgjorelseId();
String rhsAvgjorelseId;
rhsAvgjorelseId = that.getAvgjorelseId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "avgjorelseId", lhsAvgjorelseId), LocatorUtils.property(thatLocator, "avgjorelseId", rhsAvgjorelseId), lhsAvgjorelseId, rhsAvgjorelseId)) {
return false;
}
}
{
String lhsOmgjortavAvgjorelseId;
lhsOmgjortavAvgjorelseId = this.getOmgjortavAvgjorelseId();
String rhsOmgjortavAvgjorelseId;
rhsOmgjortavAvgjorelseId = that.getOmgjortavAvgjorelseId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "omgjortavAvgjorelseId", lhsOmgjortavAvgjorelseId), LocatorUtils.property(thatLocator, "omgjortavAvgjorelseId", rhsOmgjortavAvgjorelseId), lhsOmgjortavAvgjorelseId, rhsOmgjortavAvgjorelseId)) {
return false;
}
}
{
Avgjorelsestype lhsAvgjorelsestype;
lhsAvgjorelsestype = this.getAvgjorelsestype();
Avgjorelsestype rhsAvgjorelsestype;
rhsAvgjorelsestype = that.getAvgjorelsestype();
if (!strategy.equals(LocatorUtils.property(thisLocator, "avgjorelsestype", lhsAvgjorelsestype), LocatorUtils.property(thatLocator, "avgjorelsestype", rhsAvgjorelsestype), lhsAvgjorelsestype, rhsAvgjorelsestype)) {
return false;
}
}
{
boolean lhsErPositiv;
lhsErPositiv = (true?this.isErPositiv():false);
boolean rhsErPositiv;
rhsErPositiv = (true?that.isErPositiv():false);
if (!strategy.equals(LocatorUtils.property(thisLocator, "erPositiv", lhsErPositiv), LocatorUtils.property(thatLocator, "erPositiv", rhsErPositiv), lhsErPositiv, rhsErPositiv)) {
return false;
}
}
{
Tillatelse lhsTillatelse;
lhsTillatelse = this.getTillatelse();
Tillatelse rhsTillatelse;
rhsTillatelse = that.getTillatelse();
if (!strategy.equals(LocatorUtils.property(thisLocator, "tillatelse", lhsTillatelse), LocatorUtils.property(thatLocator, "tillatelse", rhsTillatelse), lhsTillatelse, rhsTillatelse)) {
return false;
}
}
{
Utfall lhsUtfall;
lhsUtfall = this.getUtfall();
Utfall rhsUtfall;
rhsUtfall = that.getUtfall();
if (!strategy.equals(LocatorUtils.property(thisLocator, "utfall", lhsUtfall), LocatorUtils.property(thatLocator, "utfall", rhsUtfall), lhsUtfall, rhsUtfall)) {
return false;
}
}
{
XMLGregorianCalendar lhsAvgjorelseDato;
lhsAvgjorelseDato = this.getAvgjorelseDato();
XMLGregorianCalendar rhsAvgjorelseDato;
rhsAvgjorelseDato = that.getAvgjorelseDato();
if (!strategy.equals(LocatorUtils.property(thisLocator, "avgjorelseDato", lhsAvgjorelseDato), LocatorUtils.property(thatLocator, "avgjorelseDato", rhsAvgjorelseDato), lhsAvgjorelseDato, rhsAvgjorelseDato)) {
return false;
}
}
{
XMLGregorianCalendar lhsEffektueringsDato;
lhsEffektueringsDato = this.getEffektueringsDato();
XMLGregorianCalendar rhsEffektueringsDato;
rhsEffektueringsDato = that.getEffektueringsDato();
if (!strategy.equals(LocatorUtils.property(thisLocator, "effektueringsDato", lhsEffektueringsDato), LocatorUtils.property(thatLocator, "effektueringsDato", rhsEffektueringsDato), lhsEffektueringsDato, rhsEffektueringsDato)) {
return false;
}
}
{
XMLGregorianCalendar lhsIverksettelseDato;
lhsIverksettelseDato = this.getIverksettelseDato();
XMLGregorianCalendar rhsIverksettelseDato;
rhsIverksettelseDato = that.getIverksettelseDato();
if (!strategy.equals(LocatorUtils.property(thisLocator, "iverksettelseDato", lhsIverksettelseDato), LocatorUtils.property(thatLocator, "iverksettelseDato", rhsIverksettelseDato), lhsIverksettelseDato, rhsIverksettelseDato)) {
return false;
}
}
{
XMLGregorianCalendar lhsUtreisefristDato;
lhsUtreisefristDato = this.getUtreisefristDato();
XMLGregorianCalendar rhsUtreisefristDato;
rhsUtreisefristDato = that.getUtreisefristDato();
if (!strategy.equals(LocatorUtils.property(thisLocator, "utreisefristDato", lhsUtreisefristDato), LocatorUtils.property(thatLocator, "utreisefristDato", rhsUtreisefristDato), lhsUtreisefristDato, rhsUtreisefristDato)) {
return false;
}
}
{
String lhsSaksnummer;
lhsSaksnummer = this.getSaksnummer();
String rhsSaksnummer;
rhsSaksnummer = that.getSaksnummer();
if (!strategy.equals(LocatorUtils.property(thisLocator, "saksnummer", lhsSaksnummer), LocatorUtils.property(thatLocator, "saksnummer", rhsSaksnummer), lhsSaksnummer, rhsSaksnummer)) {
return false;
}
}
{
String lhsEtat;
lhsEtat = this.getEtat();
String rhsEtat;
rhsEtat = that.getEtat();
if (!strategy.equals(LocatorUtils.property(thisLocator, "etat", lhsEtat), LocatorUtils.property(thatLocator, "etat", rhsEtat), lhsEtat, rhsEtat)) {
return false;
}
}
{
Boolean lhsFlyktingstatus;
lhsFlyktingstatus = this.isFlyktingstatus();
Boolean rhsFlyktingstatus;
rhsFlyktingstatus = that.isFlyktingstatus();
if (!strategy.equals(LocatorUtils.property(thisLocator, "flyktingstatus", lhsFlyktingstatus), LocatorUtils.property(thatLocator, "flyktingstatus", rhsFlyktingstatus), lhsFlyktingstatus, rhsFlyktingstatus)) {
return false;
}
}
{
Boolean lhsUavklartFlyktningstatus;
lhsUavklartFlyktningstatus = this.isUavklartFlyktningstatus();
Boolean rhsUavklartFlyktningstatus;
rhsUavklartFlyktningstatus = that.isUavklartFlyktningstatus();
if (!strategy.equals(LocatorUtils.property(thisLocator, "uavklartFlyktningstatus", lhsUavklartFlyktningstatus), LocatorUtils.property(thatLocator, "uavklartFlyktningstatus", rhsUavklartFlyktningstatus), lhsUavklartFlyktningstatus, rhsUavklartFlyktningstatus)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy