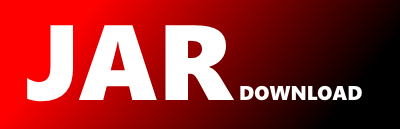
no.udi.mt_1067_nav_data.v1.PersonNavn Maven / Gradle / Ivy
package no.udi.mt_1067_nav_data.v1;
import java.io.Serializable;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
* Grunnleggende opplysninger om navn
*
* Java class for PersonNavn complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="PersonNavn">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Fornavn" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="Mellomnavn" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="Etternavn" type="{http://www.w3.org/2001/XMLSchema}string"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PersonNavn", propOrder = {
"fornavn",
"mellomnavn",
"etternavn"
})
public class PersonNavn
implements Serializable, Equals, HashCode
{
@XmlElement(name = "Fornavn", required = true)
protected String fornavn;
@XmlElement(name = "Mellomnavn")
protected String mellomnavn;
@XmlElement(name = "Etternavn", required = true)
protected String etternavn;
/**
* Gets the value of the fornavn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFornavn() {
return fornavn;
}
/**
* Sets the value of the fornavn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFornavn(String value) {
this.fornavn = value;
}
/**
* Gets the value of the mellomnavn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMellomnavn() {
return mellomnavn;
}
/**
* Sets the value of the mellomnavn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMellomnavn(String value) {
this.mellomnavn = value;
}
/**
* Gets the value of the etternavn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEtternavn() {
return etternavn;
}
/**
* Sets the value of the etternavn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEtternavn(String value) {
this.etternavn = value;
}
public PersonNavn withFornavn(String value) {
setFornavn(value);
return this;
}
public PersonNavn withMellomnavn(String value) {
setMellomnavn(value);
return this;
}
public PersonNavn withEtternavn(String value) {
setEtternavn(value);
return this;
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
String theFornavn;
theFornavn = this.getFornavn();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "fornavn", theFornavn), currentHashCode, theFornavn);
}
{
String theMellomnavn;
theMellomnavn = this.getMellomnavn();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "mellomnavn", theMellomnavn), currentHashCode, theMellomnavn);
}
{
String theEtternavn;
theEtternavn = this.getEtternavn();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "etternavn", theEtternavn), currentHashCode, theEtternavn);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof PersonNavn)) {
return false;
}
if (this == object) {
return true;
}
final PersonNavn that = ((PersonNavn) object);
{
String lhsFornavn;
lhsFornavn = this.getFornavn();
String rhsFornavn;
rhsFornavn = that.getFornavn();
if (!strategy.equals(LocatorUtils.property(thisLocator, "fornavn", lhsFornavn), LocatorUtils.property(thatLocator, "fornavn", rhsFornavn), lhsFornavn, rhsFornavn)) {
return false;
}
}
{
String lhsMellomnavn;
lhsMellomnavn = this.getMellomnavn();
String rhsMellomnavn;
rhsMellomnavn = that.getMellomnavn();
if (!strategy.equals(LocatorUtils.property(thisLocator, "mellomnavn", lhsMellomnavn), LocatorUtils.property(thatLocator, "mellomnavn", rhsMellomnavn), lhsMellomnavn, rhsMellomnavn)) {
return false;
}
}
{
String lhsEtternavn;
lhsEtternavn = this.getEtternavn();
String rhsEtternavn;
rhsEtternavn = that.getEtternavn();
if (!strategy.equals(LocatorUtils.property(thisLocator, "etternavn", lhsEtternavn), LocatorUtils.property(thatLocator, "etternavn", rhsEtternavn), lhsEtternavn, rhsEtternavn)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy