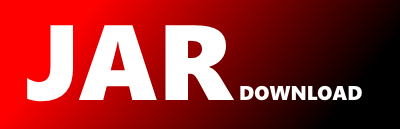
no.nav.tjeneste.virksomhet.varseldittnav.v2.meldinger.WSOpprettVarselRequest Maven / Gradle / Ivy
package no.nav.tjeneste.virksomhet.varseldittnav.v2.meldinger;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
* Java class for opprettVarselRequest complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="opprettVarselRequest">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AktoerId" type="{http://nav.no/tjeneste/virksomhet/varselDittNAV/v2/informasjon}AktoerId"/>
* <element name="varselTekst" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="varselId" type="{http://nav.no/tjeneste/virksomhet/varselDittNAV/v2/informasjon}Identifikator"/>
* <element name="varseltypeId" type="{http://nav.no/tjeneste/virksomhet/varselDittNAV/v2/informasjon}Identifikator"/>
* <element name="varselURL" type="{http://www.w3.org/2001/XMLSchema}anyURI" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "opprettVarselRequest", propOrder = {
"aktoerId",
"varselTekst",
"varselId",
"varseltypeId",
"varselURL"
})
public class WSOpprettVarselRequest
implements Equals, HashCode
{
@XmlElement(name = "AktoerId", required = true)
protected String aktoerId;
@XmlElement(required = true)
protected String varselTekst;
@XmlElement(required = true)
protected String varselId;
@XmlElement(required = true)
protected String varseltypeId;
@XmlSchemaType(name = "anyURI")
protected String varselURL;
/**
* Gets the value of the aktoerId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAktoerId() {
return aktoerId;
}
/**
* Sets the value of the aktoerId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAktoerId(String value) {
this.aktoerId = value;
}
/**
* Gets the value of the varselTekst property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVarselTekst() {
return varselTekst;
}
/**
* Sets the value of the varselTekst property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVarselTekst(String value) {
this.varselTekst = value;
}
/**
* Gets the value of the varselId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVarselId() {
return varselId;
}
/**
* Sets the value of the varselId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVarselId(String value) {
this.varselId = value;
}
/**
* Gets the value of the varseltypeId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVarseltypeId() {
return varseltypeId;
}
/**
* Sets the value of the varseltypeId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVarseltypeId(String value) {
this.varseltypeId = value;
}
/**
* Gets the value of the varselURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVarselURL() {
return varselURL;
}
/**
* Sets the value of the varselURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVarselURL(String value) {
this.varselURL = value;
}
public WSOpprettVarselRequest withAktoerId(String value) {
setAktoerId(value);
return this;
}
public WSOpprettVarselRequest withVarselTekst(String value) {
setVarselTekst(value);
return this;
}
public WSOpprettVarselRequest withVarselId(String value) {
setVarselId(value);
return this;
}
public WSOpprettVarselRequest withVarseltypeId(String value) {
setVarseltypeId(value);
return this;
}
public WSOpprettVarselRequest withVarselURL(String value) {
setVarselURL(value);
return this;
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = 1;
{
String theAktoerId;
theAktoerId = this.getAktoerId();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "aktoerId", theAktoerId), currentHashCode, theAktoerId);
}
{
String theVarselTekst;
theVarselTekst = this.getVarselTekst();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "varselTekst", theVarselTekst), currentHashCode, theVarselTekst);
}
{
String theVarselId;
theVarselId = this.getVarselId();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "varselId", theVarselId), currentHashCode, theVarselId);
}
{
String theVarseltypeId;
theVarseltypeId = this.getVarseltypeId();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "varseltypeId", theVarseltypeId), currentHashCode, theVarseltypeId);
}
{
String theVarselURL;
theVarselURL = this.getVarselURL();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "varselURL", theVarselURL), currentHashCode, theVarselURL);
}
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy strategy) {
if (!(object instanceof WSOpprettVarselRequest)) {
return false;
}
if (this == object) {
return true;
}
final WSOpprettVarselRequest that = ((WSOpprettVarselRequest) object);
{
String lhsAktoerId;
lhsAktoerId = this.getAktoerId();
String rhsAktoerId;
rhsAktoerId = that.getAktoerId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "aktoerId", lhsAktoerId), LocatorUtils.property(thatLocator, "aktoerId", rhsAktoerId), lhsAktoerId, rhsAktoerId)) {
return false;
}
}
{
String lhsVarselTekst;
lhsVarselTekst = this.getVarselTekst();
String rhsVarselTekst;
rhsVarselTekst = that.getVarselTekst();
if (!strategy.equals(LocatorUtils.property(thisLocator, "varselTekst", lhsVarselTekst), LocatorUtils.property(thatLocator, "varselTekst", rhsVarselTekst), lhsVarselTekst, rhsVarselTekst)) {
return false;
}
}
{
String lhsVarselId;
lhsVarselId = this.getVarselId();
String rhsVarselId;
rhsVarselId = that.getVarselId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "varselId", lhsVarselId), LocatorUtils.property(thatLocator, "varselId", rhsVarselId), lhsVarselId, rhsVarselId)) {
return false;
}
}
{
String lhsVarseltypeId;
lhsVarseltypeId = this.getVarseltypeId();
String rhsVarseltypeId;
rhsVarseltypeId = that.getVarseltypeId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "varseltypeId", lhsVarseltypeId), LocatorUtils.property(thatLocator, "varseltypeId", rhsVarseltypeId), lhsVarseltypeId, rhsVarseltypeId)) {
return false;
}
}
{
String lhsVarselURL;
lhsVarselURL = this.getVarselURL();
String rhsVarselURL;
rhsVarselURL = that.getVarselURL();
if (!strategy.equals(LocatorUtils.property(thisLocator, "varselURL", lhsVarselURL), LocatorUtils.property(thatLocator, "varselURL", rhsVarselURL), lhsVarselURL, rhsVarselURL)) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy