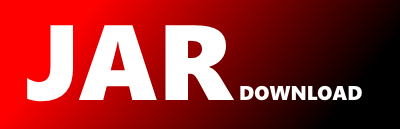
no.skatteetaten.aurora.mockmvc.extensions.mockwebserver.mockWebServer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mockmvc-extensions-kotlin Show documentation
Show all versions of mockmvc-extensions-kotlin Show documentation
Simplifies setup of MockMvc tests combined with generation of restdocs and Spring Cloud Contract stubs
package no.skatteetaten.aurora.mockmvc.extensions.mockwebserver
import com.fasterxml.jackson.databind.ObjectMapper
import com.fasterxml.jackson.module.kotlin.convertValue
import com.fasterxml.jackson.module.kotlin.jacksonObjectMapper
import com.jayway.jsonpath.JsonPath
import okhttp3.mockwebserver.MockResponse
import okhttp3.mockwebserver.MockWebServer
import okhttp3.mockwebserver.RecordedRequest
import org.springframework.core.io.ClassPathResource
import org.springframework.http.HttpHeaders
import org.springframework.http.MediaType
private fun MockWebServer.enqueueJson(status: Int = 200, body: Any, objectMapper: ObjectMapper) {
val json = body as? String ?: objectMapper.writeValueAsString(body)
val response = MockResponse()
.setResponseCode(status)
.addHeader(HttpHeaders.CONTENT_TYPE, MediaType.APPLICATION_JSON_UTF8_VALUE)
.setBody(json)
this.enqueue(response)
}
fun MockWebServer.execute(vararg responses: MockResponse, fn: () -> Unit): List {
fun takeRequests() = (1..responses.size).toList().map { this.takeRequest() }
try {
responses.forEach { this.enqueue(it) }
fn()
return takeRequests()
} catch (t: Throwable) {
takeRequests()
throw t
}
}
fun MockWebServer.execute(
vararg responses: Pair,
objectMapper: ObjectMapper = jacksonObjectMapper(),
fn: () -> Unit
): List {
fun takeRequests() = (1..responses.size).toList().map { this.takeRequest() }
try {
responses.forEach { this.enqueueJson(status = it.first, body = it.second, objectMapper = objectMapper) }
fn()
return takeRequests()
} catch (t: Throwable) {
takeRequests()
throw t
}
}
fun MockWebServer.execute(
vararg responses: Any,
objectMapper: ObjectMapper = jacksonObjectMapper(),
fn: () -> Unit
): List {
fun takeRequests() = (1..responses.size).toList().map { this.takeRequest() }
try {
responses.forEach { this.enqueueJson(body = it, objectMapper = objectMapper) }
fn()
return takeRequests()
} catch (t: Throwable) {
takeRequests()
throw t
}
}
fun MockResponse.setJsonFileAsBody(fileName: String): MockResponse {
val classPath = ClassPathResource("/$fileName")
val json = classPath.file.readText()
this.addHeader(HttpHeaders.CONTENT_TYPE, MediaType.APPLICATION_JSON_UTF8_VALUE)
return this.setBody(json)
}
inline fun RecordedRequest.bodyAsObject(
path: String = "$",
objectMapper: ObjectMapper = jacksonObjectMapper()
): T {
val content: Any = JsonPath.parse(String(body.readByteArray())).read(path)
return objectMapper.convertValue(content)
}
fun RecordedRequest.bodyAsString(): String = this.body.readUtf8()
© 2015 - 2025 Weber Informatics LLC | Privacy Policy