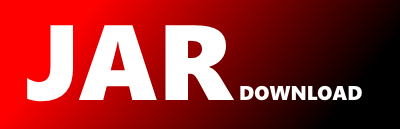
w3c.css.properties.css3.CssColumnWidth Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cssvalidator Show documentation
Show all versions of cssvalidator Show documentation
Backend for the W3C CSS Validation Service
//
// From Sijtsche de Jong ([email protected])
// Rewriten 2010 Yves Lafon
//
// (c) COPYRIGHT 1995-2010 World Wide Web Consortium (MIT, ERCIM, Keio, Beihang)
// Please first read the full copyright statement in file COPYRIGHT.html
package org.w3c.css.properties.css3;
import org.w3c.css.properties.css.CssProperty;
import org.w3c.css.util.ApplContext;
import org.w3c.css.util.InvalidParamException;
import org.w3c.css.values.CssCheckableValue;
import org.w3c.css.values.CssExpression;
import org.w3c.css.values.CssFunction;
import org.w3c.css.values.CssIdent;
import org.w3c.css.values.CssTypes;
import org.w3c.css.values.CssValue;
/**
* @spec https://www.w3.org/TR/2018/WD-css-multicol-1-20180528/#propdef-column-width
* @spec https://www.w3.org/TR/2018/WD-css-sizing-3-20180304/#column-sizing
*/
public class CssColumnWidth extends org.w3c.css.properties.css.CssColumnWidth {
public static final CssIdent[] allowed_values;
static {
// max|min-content from css-sizing
String[] _allowed_values = {"auto", "max-content", "min-content"};
allowed_values = new CssIdent[_allowed_values.length];
int i = 0;
for (String s : _allowed_values) {
allowed_values[i++] = CssIdent.getIdent(s);
}
}
public static CssIdent getAllowedIdent(CssIdent ident) {
for (CssIdent id : allowed_values) {
if (id.equals(ident)) {
return id;
}
}
return null;
}
/**
* Create a new CssColumnWidth
*/
public CssColumnWidth() {
value = initial;
}
/**
* Create a new CssColumnWidth
*
* @param expression The expression for this property
* @throws org.w3c.css.util.InvalidParamException
* Incorrect value
*/
public CssColumnWidth(ApplContext ac, CssExpression expression,
boolean check) throws InvalidParamException {
setByUser();
CssValue val = expression.getValue();
if (check && expression.getCount() > 1) {
throw new InvalidParamException("unrecognize", ac);
}
switch (val.getType()) {
case CssTypes.CSS_NUMBER:
val.getCheckableValue().checkEqualsZero(ac, this);
case CssTypes.CSS_LENGTH:
CssCheckableValue l = val.getCheckableValue();
l.checkPositiveness(ac, this);
// warn for 1px clamping
if (l.isZero()) {
ac.getFrame().addWarning("greaterequal", new String[]{l.toString(), "1px"});
}
value = val;
break;
case CssTypes.CSS_FUNCTION:
value = parseFitContentFunction(ac, (CssFunction) val, this);
break;
case CssTypes.CSS_IDENT:
if (inherit.equals(val)) {
value = inherit;
} else {
CssIdent id = getAllowedIdent((CssIdent) val);
if (id != null) {
value = id;
} else {
throw new InvalidParamException("unrecognize", ac);
}
}
break;
default:
throw new InvalidParamException("value", expression.getValue(),
getPropertyName(), ac);
}
expression.next();
}
public CssColumnWidth(ApplContext ac, CssExpression expression)
throws InvalidParamException {
this(ac, expression, false);
}
/**
* Is the value of this property a default value
* It is used by all macro for the function print
*/
public boolean isDefault() {
return (value == initial);
}
/**
* @spec https://www.w3.org/TR/2018/WD-css-sizing-3-20180304/#valdef-column-width-fit-content-length-percentage
*/
protected static CssFunction parseFitContentFunction(ApplContext ac, CssFunction function,
CssProperty caller)
throws InvalidParamException {
CssExpression exp = function.getParameters();
CssValue val;
if (!"fit-content".equals(function.getName())) {
throw new InvalidParamException("function", ac);
}
if (exp.getCount() > 1) {
throw new InvalidParamException("function", ac);
}
val = exp.getValue();
switch (val.getType()) {
case CssTypes.CSS_NUMBER:
val.getCheckableValue().checkEqualsZero(ac, caller);
case CssTypes.CSS_PERCENTAGE:
case CssTypes.CSS_LENGTH:
break;
default:
throw new InvalidParamException("value", val,
caller.getPropertyName(), ac);
}
return function;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy