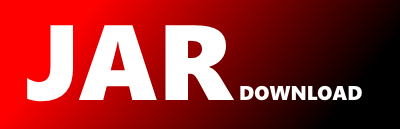
nu.zoom.swing.field.LabeledValidatingField Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of base Show documentation
Show all versions of base Show documentation
This project contains some utility classes that have been used in various projects throughout the years.
package nu.zoom.swing.field;
import java.awt.Color;
import javax.swing.JLabel;
import javax.swing.text.Document;
/**
* A validating field that is tied to a JLabel. If the field fails validation
* the label is colored red. WHen the field is valid the label is set to the
* color it had when creating the field. When validation fails the fields
* tooltip text is changed to the validation failure message.
*/
public abstract class LabeledValidatingField extends ValidatingField {
private static final long serialVersionUID = 2797888906243489866L;
private JLabel fieldLabel;
private Color okForegroundColor;
private String defaultToolTipText;
/**
* Create a new field and tie it to a label.
*
* @param fieldLabel
* The label that is tied to this field. may not be null.
*/
public LabeledValidatingField(JLabel fieldLabel) {
super();
if (fieldLabel == null) {
throw new IllegalArgumentException("Field label may not be null");
}
this.fieldLabel = fieldLabel;
okForegroundColor = fieldLabel.getForeground();
}
/**
* @see #LabeledValidatingField(JLabel)
* @param fieldLabel
* @param doc
* @param text
* @param columns
*/
public LabeledValidatingField(JLabel fieldLabel, Document doc, String text, int columns) {
super(doc, text, columns);
if (fieldLabel == null) {
throw new IllegalArgumentException("Field label may not be null");
}
this.fieldLabel = fieldLabel;
okForegroundColor = fieldLabel.getForeground();
}
/**
* @see #LabeledValidatingField(JLabel)
* @param fieldLabel
* @param columns
*/
public LabeledValidatingField(JLabel fieldLabel, int columns) {
super(columns);
if (fieldLabel == null) {
throw new IllegalArgumentException("Field label may not be null");
}
this.fieldLabel = fieldLabel;
okForegroundColor = fieldLabel.getForeground();
}
/**
* @see #LabeledValidatingField(JLabel)
* @param fieldLabel
* @param text
* @param columns
*/
public LabeledValidatingField(JLabel fieldLabel, String text, int columns) {
super(text, columns);
if (fieldLabel == null) {
throw new IllegalArgumentException("Field label may not be null");
}
this.fieldLabel = fieldLabel;
okForegroundColor = fieldLabel.getForeground();
}
/**
* @see #LabeledValidatingField(JLabel)
* @param fieldLabel
* @param text
*/
public LabeledValidatingField(JLabel fieldLabel, String text) {
super(text);
if (fieldLabel == null) {
throw new IllegalArgumentException("Field label may not be null");
}
this.fieldLabel = fieldLabel;
okForegroundColor = fieldLabel.getForeground();
}
@Override
protected abstract ValidationResult validateDocument();
@Override
protected void validationFailed(String message) {
if (defaultToolTipText == null) {
defaultToolTipText = getToolTipText();
}
setToolTipText(message);
fieldLabel.setToolTipText(message);
fieldLabel.setForeground(Color.RED);
}
@Override
protected void validationPassed() {
setToolTipText(defaultToolTipText);
fieldLabel.setToolTipText(defaultToolTipText);
defaultToolTipText = null;
fieldLabel.setForeground(okForegroundColor);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy