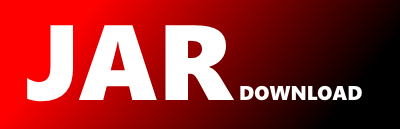
nu.zoom.swing.layout.VerticalPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of base Show documentation
Show all versions of base Show documentation
This project contains some utility classes that have been used in various projects throughout the years.
/*
* Copyright (C) 2005 Johan Maasing johan at zoom.nu Licensed under the Apache
* License, Version 2.0 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law
* or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package nu.zoom.swing.layout;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import javax.swing.JComponent;
import javax.swing.JLabel;
/**
* A panel that lays out components vertically - lika a vertical box layout.
* This panel was written to make it easy to create a grid with label-field
* components stacked in a column. Components added to this panel will grow
* horizontally but not vertically if the size changes. There can be one or two
* components in a single row. To get two components on a row use the addRow
* method that takes two arguments. The first component will be considered a
* label for the second component. The label (the first) component will not grow
* horizontally.
*
* @version $Revision: 1.3 $
* @author $Author: johan $
*/
@SuppressWarnings("serial")
public class VerticalPanel extends JComponent {
private GridBagLayout layout = new GridBagLayout();
private GridBagConstraints labelConstraints;
private GridBagConstraints componentConstraints;
private GridBagConstraints singleConstraints;
/**
* Create the panel.
*/
public VerticalPanel() {
setLayout(layout);
labelConstraints = new GridBagConstraints();
labelConstraints.anchor = GridBagConstraints.FIRST_LINE_START;
labelConstraints.fill = GridBagConstraints.HORIZONTAL;
labelConstraints.gridwidth = GridBagConstraints.RELATIVE;
labelConstraints.ipadx = 2;
labelConstraints.weightx = 0;
labelConstraints.weighty = 0;
componentConstraints = new GridBagConstraints();
componentConstraints.anchor = GridBagConstraints.FIRST_LINE_START;
componentConstraints.fill = GridBagConstraints.HORIZONTAL;
componentConstraints.gridwidth = GridBagConstraints.REMAINDER;
componentConstraints.weightx = 1;
componentConstraints.weighty = 0;
singleConstraints = new GridBagConstraints();
singleConstraints.anchor = GridBagConstraints.FIRST_LINE_START;
singleConstraints.fill = GridBagConstraints.HORIZONTAL;
singleConstraints.gridwidth = GridBagConstraints.REMAINDER;
singleConstraints.weightx = 1;
singleConstraints.weighty = 0;
}
/**
* Add a component that occupies an entire row.
*
* @param component
* The component to add, may not be null.
*/
public void addRow(JComponent component) {
if (component == null) {
throw new IllegalArgumentException("component can not be null");
}
layout.setConstraints(component, singleConstraints);
add(component);
}
/**
* Add two strings to a row. The Strings will be converted to JLabel
* components.
*
* @see #addRow(JComponent, JComponent)
* @param label
* The first (leftmost) value.
* @param value
* The second (rightmost) value
*/
public void addRow(String label, String value) {
addRow(new JLabel(label), new JLabel(value));
}
/**
* Add a row. The label string will be converted to a JLabel.
*
* @see #addRow(JComponent, JComponent)
* @param label
* The left component.
* @param component
* The right component, may not be null.
*/
public void addRow(String label, JComponent component) {
addRow(new JLabel(label), component);
}
/**
* Add a row consisting of two components.
*
* @param label
* The left component, may not be null.
* @param value
* The right component, may not be null.
*/
public void addRow(JComponent label, JComponent value) {
if (label == null) {
throw new IllegalArgumentException("Label can not be null");
}
if (value == null) {
throw new IllegalArgumentException("Value can not be null");
}
layout.setConstraints(label, labelConstraints);
add(label);
layout.setConstraints(value, componentConstraints);
add(value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy