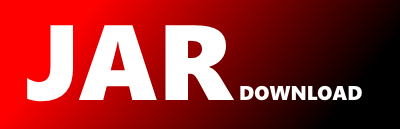
nu.zoom.util.dns.impl.ResolverImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of base Show documentation
Show all versions of base Show documentation
This project contains some utility classes that have been used in various projects throughout the years.
/*
* Copyright 2009 joma7188.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* under the License.
*/
package nu.zoom.util.dns.impl;
import java.net.InetAddress;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.Hashtable;
import java.util.List;
import javax.naming.Context;
import javax.naming.NamingException;
import javax.naming.directory.Attribute;
import javax.naming.directory.Attributes;
import javax.naming.directory.InitialDirContext;
import nu.zoom.util.dns.Resolver;
import nu.zoom.util.dns.SRVRecord;
import nu.zoom.util.dns.UnknownResponseException;
/**
* @see Resolver
* @author joma7188
*/
public class ResolverImpl implements Resolver {
private final Hashtable env = new Hashtable();
public ResolverImpl() {
env.put(Context.INITIAL_CONTEXT_FACTORY, "com.sun.jndi.dns.DnsContextFactory");
}
@Override
public List lookupSRV(String domain) throws NamingException, UnknownResponseException {
ArrayList result = new ArrayList();
InitialDirContext ictx = new InitialDirContext(env);
String[] attrs = {"SRV"};
Attributes attributes = ictx.getAttributes(domain, attrs);
if (attributes != null) {
Attribute attribute = attributes.get("SRV");
if (attribute != null) {
for (int i = 0; i < attribute.size(); i++) {
String srvEntry = "" + attribute.get(i);
String[] srvEntryParts = srvEntry.split(" ");
if (srvEntryParts.length != 4) {
throw new UnknownResponseException("Unable to parse: " + srvEntry);
} else {
int prio = Integer.parseInt(srvEntryParts[0]);
int weight = Integer.parseInt(srvEntryParts[1]);
int port = Integer.parseInt(srvEntryParts[2]);
SRVRecord record = new SRVRecord(srvEntryParts[3], port, prio, weight);
result.add(record);
}
}
}
}
return result;
}
@Override
public List lookupA(String domain) throws NamingException, UnknownResponseException, UnknownHostException {
ArrayList result = new ArrayList();
InitialDirContext ictx = new InitialDirContext(env);
String[] attrs = {"A"};
Attributes attributes = ictx.getAttributes(domain, attrs);
if (attributes != null) {
Attribute attribute = attributes.get("A");
if (attribute != null) {
for (int i = 0; i < attribute.size(); i++) {
String srvEntry = "" + attribute.get(i);
result.add(InetAddress.getByName(srvEntry));
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy