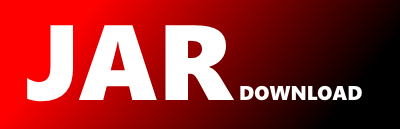
nu.zoom.swing.desktop.preferences.Preferences Maven / Gradle / Ivy
/*
* Copyright (C) 2005 Johan Maasing johan at zoom.nu Licensed under the Apache
* License, Version 2.0 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law
* or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package nu.zoom.swing.desktop.preferences;
import java.util.Set;
import nu.zoom.swing.desktop.common.BackendException;
/**
* Used by the desktop to store GUI-preferences. Acts a little lika a persistent
* Hashmap in that key-value pairs are used to set/get information. The standard
* Workbench implementation of this is to use the JDK Preferences API.
*
* @author $Author: johan $
* @version $Revision: 1.6 $
*/
public interface Preferences
{
/**
* Get a String value.
*
* @param key
* The key which was used to store the value. May not contain '/'
* slash characters. May not be null or zero length. Max 80 characters.
* @return The value. Null if no value is found.
* @throws InvalidDataTypeException
* If the value can not be converted to a string.
* @throws BackendException
*/
public String getString(String key) throws InvalidDataTypeException,
BackendException;
/**
* Store a value.
*
* @param key
* The key which was used to store the value. May not contain '/'
* slash characters. May not be null or zero length. Max 80 characters.
* @param value
* The value to store. Can be null to erase a previously stored
* value.
* @throws BackendException
*/
public void setString(String key, String value) throws BackendException;
/**
* Get an Integer value.
*
* @param key
* The key which was used to store the value. May not contain '/'
* slash characters. May not be null or zero length. Max 80 characters.
* @return The value. Null if no value is found.
* @throws InvalidDataTypeException
* If the value can not be converted to an Integer.
* @throws InvalidDataTypeException
* @throws BackendException
*/
public Integer getInteger(String key) throws InvalidDataTypeException,
BackendException;
/**
* Store a set of Strings.
*
* @param key
* The key which was used to store the value. May not contain '/'
* slash characters. May not be null or zero length. Max 80 characters.
* @param values
* The values.
* @throws BackendException
*/
public void setStrings(String key, Set values) throws BackendException;
/**
* @param key
* The key which was used to store the value. May not contain '/'
* slash characters. May not be null or zero length. Max 80 characters.
* @return The value set. Can be null or empty set if no value was found.
* @throws InvalidDataTypeException
* @throws BackendException
*/
public Set getStrings(String key) throws InvalidDataTypeException,
BackendException;
/**
* Store a value.
*
* @param key
* The key which was used to store the value. May not contain '/'
* slash characters. May not be null or zero length. Max 80 characters.
* @param value
* The value to store. Can be null to erase a previously stored
* value.
* @throws BackendException
*/
public void setInteger(String key, Integer value) throws BackendException;
/**
* Get a byte array value.
*
* @param key
* The key which was used to store the value. May not contain '/'
* slash characters. May not be null or zero length. Max 80 characters.
* @return The value. Null if no value is found.
* @throws InvalidDataTypeException
* If the value can not be converted to a byte array.
* @throws InvalidDataTypeException
* @throws BackendException
*/
public byte[] getBytes(String key) throws InvalidDataTypeException,
BackendException;
/**
* Store a value.
*
* @param key
* The key which was used to store the value. May not contain '/'
* slash characters. May not be null or zero length. Max 80 characters.
* @param value
* The value to store. Can be null to erase a previously stored
* value.
* @throws BackendException
*/
public void setBytes(String key, byte[] value) throws BackendException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy