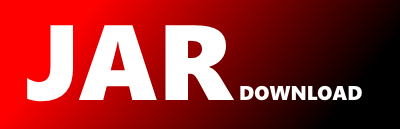
nu.zoom.swing.desktop.preferences.impl.PreferencesImpl Maven / Gradle / Ivy
/*
* Copyright (C) 2005 Johan Maasing johan at zoom.nu Licensed under the Apache
* License, Version 2.0 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law
* or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package nu.zoom.swing.desktop.preferences.impl;
import java.util.HashSet;
import java.util.Set;
import java.util.prefs.BackingStoreException;
import nu.zoom.swing.desktop.common.BackendException;
import nu.zoom.swing.desktop.preferences.InvalidDataTypeException;
import nu.zoom.swing.desktop.preferences.Preferences;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* @version $Revision: 1.6 $
* @author $Author: johan $
*/
public class PreferencesImpl implements Preferences {
private Log log = LogFactory.getLog(getClass());
private java.util.prefs.Preferences rootNode;
public PreferencesImpl() {
rootNode = java.util.prefs.Preferences
.userNodeForPackage(Preferences.class);
log.trace("Created preferences, root node: " + rootNode.absolutePath());
}
/*
* (non-Javadoc)
*
* @see nu.zoom.swing.desktop.preferences.Preferences#getString(java.lang.String)
*/
public String getString(String key) throws InvalidDataTypeException,
BackendException {
assertValidKey(key);
return rootNode.get(key, null);
}
/*
* (non-Javadoc)
*
* @see nu.zoom.swing.desktop.preferences.Preferences#setString(java.lang.String,
* java.lang.String)
*/
public void setString(String key, String value) throws BackendException {
assertValidKey(key);
if (value != null) {
rootNode.put(key, value);
} else {
rootNode.remove(key);
}
try {
rootNode.sync();
} catch (BackingStoreException e) {
throw new BackendException("Unable to flush preference node", e);
}
}
/*
* (non-Javadoc)
*
* @see nu.zoom.swing.desktop.preferences.Preferences#getInteger(java.lang.String)
*/
public Integer getInteger(String key) throws InvalidDataTypeException,
BackendException {
assertValidKey(key);
String sValue = rootNode.get(key, null);
if (sValue == null) {
return null;
} else {
try {
int iValue = Integer.parseInt(sValue);
return new Integer(iValue);
} catch (NumberFormatException e) {
throw new InvalidDataTypeException(sValue
+ " is not an integer");
}
}
}
/*
* (non-Javadoc)
*
* @see nu.zoom.swing.desktop.preferences.Preferences#setInteger(java.lang.String,
* java.lang.Integer)
*/
public void setInteger(String key, Integer value) throws BackendException {
assertValidKey(key);
if (value != null) {
rootNode.put(key, value.toString());
} else {
rootNode.remove(key);
}
try {
rootNode.sync();
} catch (BackingStoreException e) {
throw new BackendException("Unable to flush preference node", e);
}
}
/*
* (non-Javadoc)
*
* @see nu.zoom.swing.desktop.preferences.Preferences#getBytes(java.lang.String)
*/
public byte[] getBytes(String key) throws InvalidDataTypeException,
BackendException {
assertValidKey(key);
return rootNode.getByteArray(key, null);
}
/*
* (non-Javadoc)
*
* @see nu.zoom.swing.desktop.preferences.Preferences#setBytes(java.lang.String,
* byte[])
*/
public void setBytes(String key, byte[] value) throws BackendException {
assertValidKey(key);
if (value != null) {
rootNode.putByteArray(key, value);
} else {
rootNode.remove(key);
}
try {
rootNode.sync();
} catch (BackingStoreException e) {
throw new BackendException("Unable to flush preference node", e);
}
}
/*
* (non-Javadoc)
*
* @see nu.zoom.swing.desktop.preferences.Preferences#getStrings(java.lang.String)
*/
public Set getStrings(String key) throws InvalidDataTypeException,
BackendException {
assertValidKey(key);
java.util.prefs.Preferences node = rootNode.node(key);
HashSet set = new HashSet();
try {
String[] childNodeNames = node.childrenNames();
for (int i = 0; i < childNodeNames.length; i++) {
java.util.prefs.Preferences childNode = node
.node(childNodeNames[i]);
set.add(childNode.get("value", null));
}
} catch (BackingStoreException exc) {
throw new BackendException("Unable to read preference child nodes",
exc);
}
return set;
}
/*
* (non-Javadoc)
*
* @see nu.zoom.swing.desktop.preferences.Preferences#setStrings(java.lang.String,
* java.util.Set)
*/
public void setStrings(String key, Set values)
throws BackendException {
assertValidKey(key);
java.util.prefs.Preferences node = rootNode.node(key);
try {
if (node.nodeExists("")) {
node.removeNode();
}
if (node.nodeExists("")) {
throw new BackendException("Unable to remove Preferences-node "
+ key);
} else {
node = rootNode.node(key);
}
} catch (BackingStoreException e1) {
throw new BackendException("Unable to remove Preferences-node "
+ key);
}
if (values != null) {
int counter = 0;
for (String value : values) {
java.util.prefs.Preferences childNode = node.node("" + counter);
counter++;
childNode.put("value", value);
}
try {
rootNode.sync();
} catch (BackingStoreException e) {
throw new BackendException("Unable to flush preference node", e);
}
}
}
private void assertValidKey(String key) {
if ((key == null) || (key.length() < 1)) {
log.error("Preferences key may not be null or zero length");
throw new IllegalArgumentException(
"Preferences key may not be null or zero length");
}
if (key.indexOf("/") != -1) {
log.error("Preferences key may not contain '/' : " + key);
throw new IllegalArgumentException(
"Preferences key may not contain '/'");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy