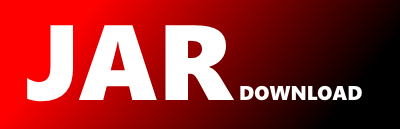
nz.ac.auckland.integration.testing.expectation.ExceptionMockExpectation Maven / Gradle / Ivy
Go to download
JFreeChart is a class library, written in Java, for generating charts.
Utilising the Java2D API, it supports a wide range of chart types including
bar charts, pie charts, line charts, XY-plots, time series plots, Sankey charts
and more.
Please wait ...