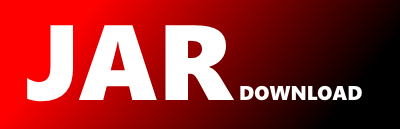
weka.classifiers.meta.Grading Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grading Show documentation
Show all versions of grading Show documentation
Implements Grading. The base classifiers are "graded".
For more information, see
A.K. Seewald, J. Fuernkranz: An Evaluation of Grading Classifiers. In: Advances in Intelligent Data Analysis: 4th International Conference, Berlin/Heidelberg/New York/Tokyo, 115-124, 2001.
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* Grading.java
* Copyright (C) 2000 University of Waikato
*
*/
package weka.classifiers.meta;
import weka.classifiers.Classifier;
import weka.classifiers.AbstractClassifier;
import weka.core.Attribute;
import weka.core.FastVector;
import weka.core.Instance;
import weka.core.DenseInstance;
import weka.core.Instances;
import weka.core.RevisionUtils;
import weka.core.TechnicalInformation;
import weka.core.TechnicalInformationHandler;
import weka.core.Utils;
import weka.core.TechnicalInformation.Field;
import weka.core.TechnicalInformation.Type;
import java.util.Random;
/**
* Implements Grading. The base classifiers are "graded".
*
* For more information, see
*
* A.K. Seewald, J. Fuernkranz: An Evaluation of Grading Classifiers. In: Advances in Intelligent Data Analysis: 4th International Conference, Berlin/Heidelberg/New York/Tokyo, 115-124, 2001.
*
*
* BibTeX:
*
* @inproceedings{Seewald2001,
* address = {Berlin/Heidelberg/New York/Tokyo},
* author = {A.K. Seewald and J. Fuernkranz},
* booktitle = {Advances in Intelligent Data Analysis: 4th International Conference},
* editor = {F. Hoffmann et al.},
* pages = {115-124},
* publisher = {Springer},
* title = {An Evaluation of Grading Classifiers},
* year = {2001}
* }
*
*
*
* Valid options are:
*
* -M <scheme specification>
* Full name of meta classifier, followed by options.
* (default: "weka.classifiers.rules.Zero")
*
* -X <number of folds>
* Sets the number of cross-validation folds.
*
* -S <num>
* Random number seed.
* (default 1)
*
* -B <classifier specification>
* Full class name of classifier to include, followed
* by scheme options. May be specified multiple times.
* (default: "weka.classifiers.rules.ZeroR")
*
* -D
* If set, classifier is run in debug mode and
* may output additional info to the console
*
*
* @author Alexander K. Seewald ([email protected])
* @author Eibe Frank ([email protected])
* @version $Revision: 8109 $
*/
public class Grading
extends Stacking
implements TechnicalInformationHandler {
/** for serialization */
static final long serialVersionUID = 5207837947890081170L;
/** The meta classifiers, one for each base classifier. */
protected Classifier [] m_MetaClassifiers = new Classifier[0];
/** InstPerClass */
protected double [] m_InstPerClass = null;
/**
* Returns a string describing classifier
* @return a description suitable for
* displaying in the explorer/experimenter gui
*/
public String globalInfo() {
return
"Implements Grading. The base classifiers are \"graded\".\n\n"
+ "For more information, see\n\n"
+ getTechnicalInformation().toString();
}
/**
* Returns an instance of a TechnicalInformation object, containing
* detailed information about the technical background of this class,
* e.g., paper reference or book this class is based on.
*
* @return the technical information about this class
*/
public TechnicalInformation getTechnicalInformation() {
TechnicalInformation result;
result = new TechnicalInformation(Type.INPROCEEDINGS);
result.setValue(Field.AUTHOR, "A.K. Seewald and J. Fuernkranz");
result.setValue(Field.TITLE, "An Evaluation of Grading Classifiers");
result.setValue(Field.BOOKTITLE, "Advances in Intelligent Data Analysis: 4th International Conference");
result.setValue(Field.EDITOR, "F. Hoffmann et al.");
result.setValue(Field.YEAR, "2001");
result.setValue(Field.PAGES, "115-124");
result.setValue(Field.PUBLISHER, "Springer");
result.setValue(Field.ADDRESS, "Berlin/Heidelberg/New York/Tokyo");
return result;
}
/**
* Generates the meta data
*
* @param newData the data to work on
* @param random the random number generator used in the generation
* @throws Exception if generation fails
*/
protected void generateMetaLevel(Instances newData, Random random)
throws Exception {
m_MetaFormat = metaFormat(newData);
Instances [] metaData = new Instances[m_Classifiers.length];
for (int i = 0; i < m_Classifiers.length; i++) {
metaData[i] = metaFormat(newData);
}
for (int j = 0; j < m_NumFolds; j++) {
Instances train = newData.trainCV(m_NumFolds, j, random);
Instances test = newData.testCV(m_NumFolds, j);
// Build base classifiers
for (int i = 0; i < m_Classifiers.length; i++) {
getClassifier(i).buildClassifier(train);
for (int k = 0; k < test.numInstances(); k++) {
metaData[i].add(metaInstance(test.instance(k),i));
}
}
}
// calculate InstPerClass
m_InstPerClass = new double[newData.numClasses()];
for (int i=0; i < newData.numClasses(); i++) m_InstPerClass[i]=0.0;
for (int i=0; i < newData.numInstances(); i++) {
m_InstPerClass[(int)newData.instance(i).classValue()]++;
}
m_MetaClassifiers = AbstractClassifier.makeCopies(m_MetaClassifier,
m_Classifiers.length);
for (int i = 0; i < m_Classifiers.length; i++) {
m_MetaClassifiers[i].buildClassifier(metaData[i]);
}
}
/**
* Returns class probabilities for a given instance using the stacked classifier.
* One class will always get all the probability mass (i.e. probability one).
*
* @param instance the instance to be classified
* @throws Exception if instance could not be classified
* successfully
* @return the class distribution for the given instance
*/
public double[] distributionForInstance(Instance instance) throws Exception {
double maxPreds;
int numPreds=0;
int numClassifiers=m_Classifiers.length;
int idxPreds;
double [] predConfs = new double[numClassifiers];
double [] preds;
for (int i=0; iMaxInstPerClass) {
MaxInstPerClass=(int)m_InstPerClass[i];
MaxClass=i;
}
}
}
int predictedIndex;
if (numPreds==1)
predictedIndex = Utils.maxIndex(preds);
else
{
// System.out.print("?");
// System.out.print(instance.toString());
// for (int i=0; imaxVal) {
maxVal=dist[j];
maxIdx=j;
}
}
predConf= (instance.classValue()==maxIdx) ? 1:0;
}
values[idx]=predConf;
metaInstance = new DenseInstance(1, values);
metaInstance.setDataset(m_MetaFormat);
return metaInstance;
}
/**
* Returns the revision string.
*
* @return the revision
*/
public String getRevision() {
return RevisionUtils.extract("$Revision: 8109 $");
}
/**
* Main method for testing this class.
*
* @param argv should contain the following arguments:
* -t training file [-T test file] [-c class index]
*/
public static void main(String [] argv) {
runClassifier(new Grading(), argv);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy