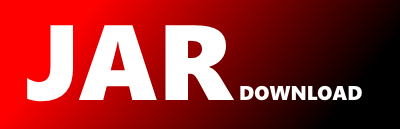
weka.associations.CaRuleGeneration Maven / Gradle / Ivy
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* CaRuleGeneration.java
* Copyright (C) 2004 University of Waikato, Hamilton, New Zealand
*
*/
package weka.associations;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Hashtable;
import java.util.TreeSet;
import weka.core.Instances;
import weka.core.RevisionHandler;
import weka.core.RevisionUtils;
import weka.core.UnassignedClassException;
/**
* Class implementing the rule generation procedure of the predictive apriori
* algorithm for class association rules.
*
* For association rules in gerneral the method is described in: T. Scheffer
* (2001). Finding Association Rules That Trade Support Optimally against
* Confidence. Proc of the 5th European Conf. on Principles and Practice of
* Knowledge Discovery in Databases (PKDD'01), pp. 424-435. Freiburg, Germany:
* Springer-Verlag.
*
*
* The implementation follows the paper expect for adding a rule to the output
* of the n best rules. A rule is added if: the expected predictive
* accuracy of this rule is among the n best and it is not subsumed by a
* rule with at least the same expected predictive accuracy (out of an
* unpublished manuscript from T. Scheffer).
*
* @author Stefan Mutter ([email protected])
* @version $Revision: 10201 $
*/
public class CaRuleGeneration extends RuleGeneration implements Serializable,
RevisionHandler {
/** for serialization */
private static final long serialVersionUID = 3065752149646517703L;
/**
* Constructor
*
* @param itemSet the item set that forms the premise of the rule
*/
public CaRuleGeneration(ItemSet itemSet) {
super(itemSet);
}
/**
* Generates all rules for an item set. The item set is the premise.
*
* @param numRules the number of association rules the use wants to mine. This
* number equals the size n of the list of the best rules.
* @param midPoints the mid points of the intervals
* @param priors Hashtable that contains the prior probabilities
* @param expectation the minimum value of the expected predictive accuracy
* that is needed to get into the list of the best rules
* @param instances the instances for which association rules are generated
* @param best the list of the n best rules. The list is implemented as
* a TreeSet
* @param genTime the maximum time of generation
* @return all the rules with minimum confidence for the given item set
*/
@Override
public TreeSet generateRules(int numRules, double[] midPoints,
Hashtable priors, double expectation, Instances instances,
TreeSet best, int genTime) {
boolean redundant = false;
ArrayList