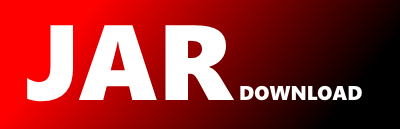
weka.classifiers.bayes.NaiveBayesMultinomial Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weka-dev Show documentation
Show all versions of weka-dev Show documentation
The Waikato Environment for Knowledge Analysis (WEKA), a machine
learning workbench. This version represents the developer version, the
"bleeding edge" of development, you could say. New functionality gets added
to this version.
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* NaiveBayesMultinomial.java
* Copyright (C) 2003-2012 University of Waikato, Hamilton, New Zealand
*/
package weka.classifiers.bayes;
import weka.classifiers.AbstractClassifier;
import weka.core.Capabilities;
import weka.core.Capabilities.Capability;
import weka.core.Instance;
import weka.core.Instances;
import weka.core.RevisionUtils;
import weka.core.TechnicalInformation;
import weka.core.TechnicalInformation.Field;
import weka.core.TechnicalInformation.Type;
import weka.core.TechnicalInformationHandler;
import weka.core.Utils;
import weka.core.WeightedInstancesHandler;
/**
* Class for building and using a multinomial Naive Bayes classifier. For more information see,
*
* Andrew Mccallum, Kamal Nigam: A Comparison of Event Models for Naive Bayes Text Classification. In: AAAI-98 Workshop on 'Learning for Text Categorization', 1998.
*
* The core equation for this classifier:
*
* P[Ci|D] = (P[D|Ci] x P[Ci]) / P[D] (Bayes rule)
*
* where Ci is class i and D is a document.
*
*
* BibTeX:
*
* @inproceedings{Mccallum1998,
* author = {Andrew Mccallum and Kamal Nigam},
* booktitle = {AAAI-98 Workshop on 'Learning for Text Categorization'},
* title = {A Comparison of Event Models for Naive Bayes Text Classification},
* year = {1998}
* }
*
*
*
* Valid options are:
*
* -D
* If set, classifier is run in debug mode and
* may output additional info to the console
*
*
* @author Andrew Golightly ([email protected])
* @author Bernhard Pfahringer ([email protected])
* @version $Revision: 11301 $
*/
public class NaiveBayesMultinomial
extends AbstractClassifier
implements WeightedInstancesHandler,TechnicalInformationHandler {
/** for serialization */
static final long serialVersionUID = 5932177440181257085L;
/**
* probability that a word (w) exists in a class (H) (i.e. Pr[w|H])
* The matrix is in the this format: probOfWordGivenClass[class][wordAttribute]
* NOTE: the values are actually the log of Pr[w|H]
*/
protected double[][] m_probOfWordGivenClass;
/** the probability of a class (i.e. Pr[H]) */
protected double[] m_probOfClass;
/** number of unique words */
protected int m_numAttributes;
/** number of class values */
protected int m_numClasses;
/** cache lnFactorial computations */
protected double[] m_lnFactorialCache = new double[]{0.0,0.0};
/** copy of header information for use in toString method */
protected Instances m_headerInfo;
/**
* Returns a string describing this classifier
* @return a description of the classifier suitable for
* displaying in the explorer/experimenter gui
*/
public String globalInfo() {
return
"Class for building and using a multinomial Naive Bayes classifier. "
+ "For more information see,\n\n"
+ getTechnicalInformation().toString() + "\n\n"
+ "The core equation for this classifier:\n\n"
+ "P[Ci|D] = (P[D|Ci] x P[Ci]) / P[D] (Bayes rule)\n\n"
+ "where Ci is class i and D is a document.";
}
/**
* Returns an instance of a TechnicalInformation object, containing
* detailed information about the technical background of this class,
* e.g., paper reference or book this class is based on.
*
* @return the technical information about this class
*/
public TechnicalInformation getTechnicalInformation() {
TechnicalInformation result;
result = new TechnicalInformation(Type.INPROCEEDINGS);
result.setValue(Field.AUTHOR, "Andrew Mccallum and Kamal Nigam");
result.setValue(Field.YEAR, "1998");
result.setValue(Field.TITLE, "A Comparison of Event Models for Naive Bayes Text Classification");
result.setValue(Field.BOOKTITLE, "AAAI-98 Workshop on 'Learning for Text Categorization'");
return result;
}
/**
* Returns default capabilities of the classifier.
*
* @return the capabilities of this classifier
*/
public Capabilities getCapabilities() {
Capabilities result = super.getCapabilities();
result.disableAll();
// attributes
result.enable(Capability.NUMERIC_ATTRIBUTES);
// class
result.enable(Capability.NOMINAL_CLASS);
result.enable(Capability.MISSING_CLASS_VALUES);
return result;
}
/**
* Generates the classifier.
*
* @param instances set of instances serving as training data
* @throws Exception if the classifier has not been generated successfully
*/
public void buildClassifier(Instances instances) throws Exception
{
// can classifier handle the data?
getCapabilities().testWithFail(instances);
// remove instances with missing class
instances = new Instances(instances);
instances.deleteWithMissingClass();
m_headerInfo = new Instances(instances, 0);
m_numClasses = instances.numClasses();
m_numAttributes = instances.numAttributes();
m_probOfWordGivenClass = new double[m_numClasses][];
/*
initialising the matrix of word counts
NOTE: Laplace estimator introduced in case a word that does not appear for a class in the
training set does so for the test set
*/
for(int c = 0; c enumInsts = instances.enumerateInstances();
while (enumInsts.hasMoreElements())
{
instance = (Instance) enumInsts.nextElement();
classIndex = (int)instance.value(instance.classIndex());
docsPerClass[classIndex] += instance.weight();
for(int a = 0; a
© 2015 - 2024 Weber Informatics LLC | Privacy Policy