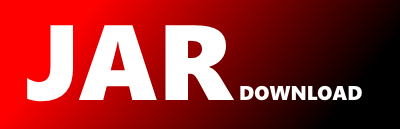
weka.classifiers.trees.RandomForest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weka-dev Show documentation
Show all versions of weka-dev Show documentation
The Waikato Environment for Knowledge Analysis (WEKA), a machine
learning workbench. This version represents the developer version, the
"bleeding edge" of development, you could say. New functionality gets added
to this version.
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* RandomForest.java
* Copyright (C) 2001-2012 University of Waikato, Hamilton, New Zealand
*
*/
package weka.classifiers.trees;
import java.util.Collections;
import java.util.Enumeration;
import java.util.Vector;
import weka.classifiers.AbstractClassifier;
import weka.classifiers.meta.Bagging;
import weka.core.AdditionalMeasureProducer;
import weka.core.Aggregateable;
import weka.core.Capabilities;
import weka.core.Instance;
import weka.core.Instances;
import weka.core.Option;
import weka.core.OptionHandler;
import weka.core.PartitionGenerator;
import weka.core.Randomizable;
import weka.core.RevisionUtils;
import weka.core.TechnicalInformation;
import weka.core.TechnicalInformation.Field;
import weka.core.TechnicalInformation.Type;
import weka.core.TechnicalInformationHandler;
import weka.core.Utils;
import weka.core.WeightedInstancesHandler;
/**
* Class for constructing a forest of random trees.
*
* For more information see:
*
* Leo Breiman (2001). Random Forests. Machine Learning. 45(1):5-32.
*
*
* BibTeX:
*
* @article{Breiman2001,
* author = {Leo Breiman},
* journal = {Machine Learning},
* number = {1},
* pages = {5-32},
* title = {Random Forests},
* volume = {45},
* year = {2001}
* }
*
*
*
* Valid options are:
*
* -I <number of trees>
* Number of trees to build.
* (default 100)
*
* -K <number of features>
* Number of features to consider (<1=int(log_2(#predictors)+1)).
* (default 0)
*
* -S
* Seed for random number generator.
* (default 1)
*
* -depth <num>
* The maximum depth of the trees, 0 for unlimited.
* (default 0)
*
* -O
* Don't calculate the out of bag error.
*
* -print
* Print the individual trees in the output
*
* -num-slots <num>
* Number of execution slots.
* (default 1 - i.e. no parallelism)
*
* -output-debug-info
* If set, classifier is run in debug mode and
* may output additional info to the console
*
* -do-not-check-capabilities
* If set, classifier capabilities are not checked before classifier is built
* (use with caution).
*
*
* @author Richard Kirkby ([email protected])
* @version $Revision: 11003 $
*/
public class RandomForest extends AbstractClassifier implements OptionHandler,
Randomizable, WeightedInstancesHandler, AdditionalMeasureProducer,
TechnicalInformationHandler, PartitionGenerator, Aggregateable {
/** for serialization */
static final long serialVersionUID = 1116839470751428698L;
/** Number of trees in forest. */
protected int m_numTrees = 100;
/**
* Number of features to consider in random feature selection. If less than 1
* will use int(log_2(M)+1) )
*/
protected int m_numFeatures = 0;
/** The random seed. */
protected int m_randomSeed = 1;
/** Final number of features that were considered in last build. */
protected int m_KValue = 0;
/** The bagger. */
protected Bagging m_bagger = null;
/** The maximum depth of the trees (0 = unlimited) */
protected int m_MaxDepth = 0;
/** The number of threads to have executing at any one time */
protected int m_numExecutionSlots = 1;
/** Print the individual trees in the output */
protected boolean m_printTrees = false;
/** Don't calculate the out of bag error */
protected boolean m_dontCalculateOutOfBagError;
/**
* Returns a string describing classifier
*
* @return a description suitable for displaying in the explorer/experimenter
* gui
*/
public String globalInfo() {
return "Class for constructing a forest of random trees.\n\n"
+ "For more information see: \n\n" + getTechnicalInformation().toString();
}
/**
* Returns an instance of a TechnicalInformation object, containing detailed
* information about the technical background of this class, e.g., paper
* reference or book this class is based on.
*
* @return the technical information about this class
*/
@Override
public TechnicalInformation getTechnicalInformation() {
TechnicalInformation result;
result = new TechnicalInformation(Type.ARTICLE);
result.setValue(Field.AUTHOR, "Leo Breiman");
result.setValue(Field.YEAR, "2001");
result.setValue(Field.TITLE, "Random Forests");
result.setValue(Field.JOURNAL, "Machine Learning");
result.setValue(Field.VOLUME, "45");
result.setValue(Field.NUMBER, "1");
result.setValue(Field.PAGES, "5-32");
return result;
}
/**
* Returns the tip text for this property
*
* @return tip text for this property suitable for displaying in the
* explorer/experimenter gui
*/
public String numTreesTipText() {
return "The number of trees to be generated.";
}
/**
* Get the value of numTrees.
*
* @return Value of numTrees.
*/
public int getNumTrees() {
return m_numTrees;
}
/**
* Set the value of numTrees.
*
* @param newNumTrees Value to assign to numTrees.
*/
public void setNumTrees(int newNumTrees) {
m_numTrees = newNumTrees;
}
/**
* Returns the tip text for this property
*
* @return tip text for this property suitable for displaying in the
* explorer/experimenter gui
*/
public String numFeaturesTipText() {
return "The number of attributes to be used in random selection (see RandomTree).";
}
/**
* Get the number of features used in random selection.
*
* @return Value of numFeatures.
*/
public int getNumFeatures() {
return m_numFeatures;
}
/**
* Set the number of features to use in random selection.
*
* @param newNumFeatures Value to assign to numFeatures.
*/
public void setNumFeatures(int newNumFeatures) {
m_numFeatures = newNumFeatures;
}
/**
* Returns the tip text for this property
*
* @return tip text for this property suitable for displaying in the
* explorer/experimenter gui
*/
public String seedTipText() {
return "The random number seed to be used.";
}
/**
* Set the seed for random number generation.
*
* @param seed the seed
*/
@Override
public void setSeed(int seed) {
m_randomSeed = seed;
}
/**
* Gets the seed for the random number generations
*
* @return the seed for the random number generation
*/
@Override
public int getSeed() {
return m_randomSeed;
}
/**
* Returns the tip text for this property
*
* @return tip text for this property suitable for displaying in the
* explorer/experimenter gui
*/
public String maxDepthTipText() {
return "The maximum depth of the trees, 0 for unlimited.";
}
/**
* Get the maximum depth of trh tree, 0 for unlimited.
*
* @return the maximum depth.
*/
public int getMaxDepth() {
return m_MaxDepth;
}
/**
* Set the maximum depth of the tree, 0 for unlimited.
*
* @param value the maximum depth.
*/
public void setMaxDepth(int value) {
m_MaxDepth = value;
}
/**
* Returns the tip text for this property
*
* @return tip text for this property suitable for displaying in the
* explorer/experimenter gui
*/
public String printTreesTipText() {
return "Print the individual trees in the output";
}
/**
* Set whether to print the individual ensemble trees in the output
*
* @param print true if the individual trees are to be printed
*/
public void setPrintTrees(boolean print) {
m_printTrees = print;
}
/**
* Get whether to print the individual ensemble trees in the output
*
* @return true if the individual trees are to be printed
*/
public boolean getPrintTrees() {
return m_printTrees;
}
/**
* Returns the tip text for this property
*
* @return tip text for this property suitable for displaying in the
* explorer/experimenter gui
*/
public String dontCalculateOutOfBagErrorTipText() {
return "If true, then the out of bag error is not computed";
}
/**
* Set whether to turn off the calculation of out of bag error
*
* @param b true to turn off the calculation of out of bag error
*/
public void setDontCalculateOutOfBagError(boolean b) {
m_dontCalculateOutOfBagError = b;
}
/**
* Get whether to turn off the calculation of out of bag error
*
* @return true to turn off the calculation of out of bag error
*/
public boolean getDontCalculateOutOfBagError() {
return m_dontCalculateOutOfBagError;
}
/**
* Gets the out of bag error that was calculated as the classifier was built.
*
* @return the out of bag error
*/
public double measureOutOfBagError() {
if (m_bagger != null && !m_dontCalculateOutOfBagError) {
return m_bagger.measureOutOfBagError();
} else {
return Double.NaN;
}
}
/**
* Set the number of execution slots (threads) to use for building the members
* of the ensemble.
*
* @param numSlots the number of slots to use.
*/
public void setNumExecutionSlots(int numSlots) {
m_numExecutionSlots = numSlots;
}
/**
* Get the number of execution slots (threads) to use for building the members
* of the ensemble.
*
* @return the number of slots to use
*/
public int getNumExecutionSlots() {
return m_numExecutionSlots;
}
/**
* Returns the tip text for this property
*
* @return tip text for this property suitable for displaying in the
* explorer/experimenter gui
*/
public String numExecutionSlotsTipText() {
return "The number of execution slots (threads) to use for "
+ "constructing the ensemble.";
}
/**
* Returns an enumeration of the additional measure names.
*
* @return an enumeration of the measure names
*/
@Override
public Enumeration enumerateMeasures() {
Vector newVector = new Vector(1);
newVector.addElement("measureOutOfBagError");
return newVector.elements();
}
/**
* Returns the value of the named measure.
*
* @param additionalMeasureName the name of the measure to query for its value
* @return the value of the named measure
* @throws IllegalArgumentException if the named measure is not supported
*/
@Override
public double getMeasure(String additionalMeasureName) {
if (additionalMeasureName.equalsIgnoreCase("measureOutOfBagError")) {
return measureOutOfBagError();
} else {
throw new IllegalArgumentException(additionalMeasureName
+ " not supported (RandomForest)");
}
}
/**
* Returns an enumeration describing the available options.
*
* @return an enumeration of all the available options
*/
@Override
public Enumeration
© 2015 - 2024 Weber Informatics LLC | Privacy Policy