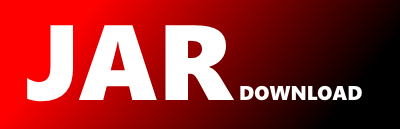
weka.core.RepositoryIndexGenerator Maven / Gradle / Ivy
Show all versions of weka-dev Show documentation
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* RepositoryIndexGenerator.java
* Copyright (C) 2000-2012 University of Waikato, Hamilton, New Zealand
*
*/
package weka.core;
import java.io.BufferedInputStream;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Comparator;
import java.util.Iterator;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import java.util.TreeMap;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
/**
* Class for generating html index files and supporting text files for a Weka
* package meta data repository. To Run
*
*
* java weka.core.RepositoryIndexGenerator
*
* @author Mark Hall (mhall{[at]}pentaho{[dot]}com)
* @version $Revision: 10203 $
*/
public class RepositoryIndexGenerator {
public static String HEADER = "\n"
+ "\n\nWaikato Environment for Knowledge Analysis (WEKA) \n"
+ "\n\n\n\n\n";
public static String BIRD_IMAGE1 = "
\n";
public static String BIRD_IMAGE2 = "
\n";
public static String PENTAHO_IMAGE1 = "
\n\n";
public static String PENTAHO_IMAGE2 = "
\n\n";
private static int[] parseVersion(String version) {
int major = 0;
int minor = 0;
int revision = 0;
int[] majMinRev = new int[3];
try {
String tmpStr = version;
tmpStr = tmpStr.replace('-', '.');
if (tmpStr.indexOf(".") > -1) {
major = Integer.parseInt(tmpStr.substring(0, tmpStr.indexOf(".")));
tmpStr = tmpStr.substring(tmpStr.indexOf(".") + 1);
if (tmpStr.indexOf(".") > -1) {
minor = Integer.parseInt(tmpStr.substring(0, tmpStr.indexOf(".")));
tmpStr = tmpStr.substring(tmpStr.indexOf(".") + 1);
if (!tmpStr.equals("")) {
revision = Integer.parseInt(tmpStr);
} else {
revision = 0;
}
} else {
if (!tmpStr.equals("")) {
minor = Integer.parseInt(tmpStr);
} else {
minor = 0;
}
}
} else {
if (!tmpStr.equals("")) {
major = Integer.parseInt(tmpStr);
} else {
major = 0;
}
}
} catch (Exception e) {
e.printStackTrace();
major = -1;
minor = -1;
revision = -1;
} finally {
majMinRev[0] = major;
majMinRev[1] = minor;
majMinRev[2] = revision;
}
return majMinRev;
}
private static String cleansePropertyValue(String propVal) {
propVal = propVal.replace("<", "<");
propVal = propVal.replace(">", ">");
propVal = propVal.replace("@", "{[at]}");
propVal = propVal.replace("\n", "
");
return propVal;
}
protected static int compare(String version1, String version2) {
// parse both of the versions
int[] majMinRev1 = parseVersion(version1);
int[] majMinRev2 = parseVersion(version2);
int result;
if (majMinRev1[0] < majMinRev2[0]) {
result = -1;
} else if (majMinRev1[0] == majMinRev2[0]) {
if (majMinRev1[1] < majMinRev2[1]) {
result = -1;
} else if (majMinRev1[1] == majMinRev2[1]) {
if (majMinRev1[2] < majMinRev2[2]) {
result = -1;
} else if (majMinRev1[2] == majMinRev2[2]) {
result = 0;
} else {
result = 1;
}
} else {
result = 1;
}
} else {
result = 1;
}
return result;
}
private static String[] processPackage(File packageDirectory)
throws Exception {
System.err.println("Processing " + packageDirectory);
File[] contents = packageDirectory.listFiles();
File latest = null;
ArrayList propsFiles = new ArrayList();
StringBuffer versionsTextBuffer = new StringBuffer();
for (File content : contents) {
if (content.isFile() && content.getName().endsWith(".props")) {
propsFiles.add(content);
if (content.getName().equals("Latest.props")) {
latest = content;
} /*
* else { String versionNumber = contents[i].getName().substring(0,
* contents[i].getName().indexOf(".props"));
* versionsTextBuffer.append(versionNumber + "\n"); }
*/
}
}
File[] sortedPropsFiles = propsFiles.toArray(new File[0]);
Arrays.sort(sortedPropsFiles, new Comparator() {
@Override
public int compare(File first, File second) {
String firstV = first.getName().substring(0,
first.getName().indexOf(".props"));
String secondV = second.getName().substring(0,
second.getName().indexOf(".props"));
if (firstV.equalsIgnoreCase("Latest")) {
return -1;
} else if (secondV.equalsIgnoreCase("Latest")) {
return 1;
}
return -RepositoryIndexGenerator.compare(firstV, secondV);
}
});
StringBuffer indexBuff = new StringBuffer();
indexBuff.append(HEADER + "\n\n");
// indexBuff.append(HEADER + BIRD_IMAGE2);
// indexBuff.append(PENTAHO_IMAGE2);
Properties latestProps = new Properties();
latestProps.load(new BufferedReader(new FileReader(latest)));
String packageName = latestProps.getProperty("PackageName") + ": ";
String packageTitle = latestProps.getProperty("Title");
String packageCategory = latestProps.getProperty("Category");
String latestVersion = latestProps.getProperty("Version");
if (packageCategory == null) {
packageCategory = "";
}
indexBuff.append("" + packageName + packageTitle + "
\n\n");
String author = latestProps.getProperty("Author");
author = cleansePropertyValue(author);
String URL = latestProps.getProperty("URL");
if (URL != null) {
URL = cleansePropertyValue(URL);
}
String maintainer = latestProps.getProperty("Maintainer");
maintainer = cleansePropertyValue(maintainer);
indexBuff.append("\n\n");
if (URL != null && URL.length() > 0) {
indexBuff.append("" + "URL"
+ ": ");
URL = "" + URL + "";
indexBuff.append("" + URL + " \n");
}
indexBuff.append("" + "Author"
+ ": ");
indexBuff.append("" + author + " \n");
indexBuff.append("" + "Maintainer"
+ ": ");
indexBuff.append("" + maintainer + " \n");
indexBuff.append("
\n\n");
String description = latestProps.getProperty("Description");
indexBuff.append("
" + description.replace("\n", "
") + "
\n\n");
indexBuff.append("All available versions:
\n");
for (int i = 0; i < sortedPropsFiles.length; i++) {
if (i > 0) {
String versionNumber = sortedPropsFiles[i].getName().substring(0,
sortedPropsFiles[i].getName().indexOf(".props"));
versionsTextBuffer.append(versionNumber + "\n");
System.err.println(versionNumber);
}
String name = sortedPropsFiles[i].getName();
name = name.substring(0, name.indexOf(".props"));
indexBuff.append("" + name
+ "
\n");
StringBuffer version = new StringBuffer();
version.append(HEADER + "\n\n");
// version.append(HEADER + BIRD_IMAGE2);
// version.append(PENTAHO_IMAGE2);
version.append("
\n");
Properties versionProps = new Properties();
versionProps
.load(new BufferedReader(new FileReader(sortedPropsFiles[i])));
Set
\n\n\n");
String versionHTMLFileName = packageDirectory.getAbsolutePath()
+ File.separator + name + ".html";
BufferedWriter br = new BufferedWriter(
new FileWriter(versionHTMLFileName));
br.write(version.toString());
br.flush();
br.close();
}
indexBuff.append("