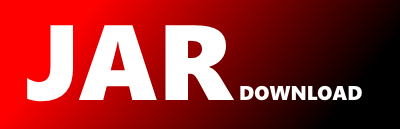
weka.core.converters.AbstractFileSaver Maven / Gradle / Ivy
Show all versions of weka-dev Show documentation
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* AbstractFileSaver.java
* Copyright (C) 2004-2012 University of Waikato, Hamilton, New Zealand
*
*/
package weka.core.converters;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.util.Enumeration;
import java.util.Vector;
import weka.core.Environment;
import weka.core.EnvironmentHandler;
import weka.core.Option;
import weka.core.OptionHandler;
import weka.core.Utils;
/**
* Abstract class for Savers that save to a file
*
* Valid options are:
*
* -i input arff file
* The input filw in arff format.
*
*
* -o the output file
* The output file. The prefix of the output file is sufficient. If no output
* file is given, Saver tries to use standard out.
*
*
*
* @author Richard Kirkby ([email protected])
* @author Stefan Mutter ([email protected])
* @version $Revision: 10233 $
*/
public abstract class AbstractFileSaver extends AbstractSaver implements
OptionHandler, FileSourcedConverter, EnvironmentHandler {
/** ID to avoid warning */
private static final long serialVersionUID = 2399441762235754491L;
/** The destination file. */
private File m_outputFile;
/** The writer. */
private transient BufferedWriter m_writer;
/** The file extension of the destination file. */
private String FILE_EXTENSION;
/** the extension for compressed files */
private final String FILE_EXTENSION_COMPRESSED = ".gz";
/** The prefix for the filename (chosen in the GUI). */
private String m_prefix;
/** The directory of the file (chosen in the GUI). */
private String m_dir;
/**
* Counter. In incremental mode after reading 100 instances they will be
* written to a file.
*/
protected int m_incrementalCounter;
/** use relative file paths */
protected boolean m_useRelativePath = false;
/** Environment variables */
protected transient Environment m_env;
/**
* resets the options
*
*/
@Override
public void resetOptions() {
super.resetOptions();
m_outputFile = null;
m_writer = null;
m_prefix = "";
m_dir = "";
m_incrementalCounter = 0;
}
/**
* Gets the writer
*
* @return the BufferedWriter
*/
public BufferedWriter getWriter() {
return m_writer;
}
/** Sets the writer to null. */
public void resetWriter() {
m_writer = null;
}
/**
* Gets ihe file extension.
*
* @return the file extension as a string.
*/
@Override
public String getFileExtension() {
return FILE_EXTENSION;
}
/**
* Gets all the file extensions used for this type of file
*
* @return the file extensions
*/
@Override
public String[] getFileExtensions() {
return new String[] { getFileExtension() };
}
/**
* Sets ihe file extension.
*
* @param ext the file extension as a string startin with '.'.
*/
protected void setFileExtension(String ext) {
FILE_EXTENSION = ext;
}
/**
* Gets the destination file.
*
* @return the destination file.
*/
@Override
public File retrieveFile() {
return m_outputFile;
}
/**
* Sets the destination file.
*
* @param outputFile the destination file.
* @throws IOException throws an IOException if file cannot be set
*/
@Override
public void setFile(File outputFile) throws IOException {
m_outputFile = outputFile;
setDestination(outputFile);
}
/**
* Sets the file name prefix
*
* @param prefix the file name prefix
*/
@Override
public void setFilePrefix(String prefix) {
m_prefix = prefix;
}
/**
* Gets the file name prefix
*
* @return the prefix of the filename
*/
@Override
public String filePrefix() {
return m_prefix;
}
/**
* Sets the directory where the instances should be stored
*
* @param dir a string containing the directory path and name
*/
@Override
public void setDir(String dir) {
m_dir = dir;
}
/**
* Gets the directory
*
* @return a string with the file name
*/
@Override
public String retrieveDir() {
return m_dir;
}
/**
* Set the environment variables to use.
*
* @param env the environment variables to use
*/
@Override
public void setEnvironment(Environment env) {
m_env = env;
if (m_outputFile != null) {
try {
// try and resolve any new environment variables
setFile(m_outputFile);
} catch (IOException ex) {
// we won't complain about it here...
}
}
}
/**
* Returns an enumeration describing the available options.
*
* @return an enumeration of all the available options.
*/
@Override
public Enumeration