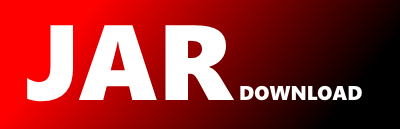
weka.gui.beans.PluginManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weka-dev Show documentation
Show all versions of weka-dev Show documentation
The Waikato Environment for Knowledge Analysis (WEKA), a machine
learning workbench. This version represents the developer version, the
"bleeding edge" of development, you could say. New functionality gets added
to this version.
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* PluginManager.java
* Copyright (C) 2011-2012 University of Waikato, Hamilton, New Zealand
*
*/
package weka.gui.beans;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.InputStream;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import java.util.TreeMap;
/**
* Class that manages a global map of plugins. The knowledge flow uses this to
* manage plugins other than step components and perspectives. Is general
* purpose, so can be used by other Weka components. Provides static methods for
* registering and instantiating plugins.
*
* @author Mark Hall (mhall{[at]}pentaho{[dot]}com)
* @version $Revision: 10221 $
*/
public class PluginManager {
/**
* Global map that is keyed by plugin base class/interface type. The inner Map
* then stores individual plugin instances of the interface type, keyed by
* plugin name/short title with values the actual fully qualified class name
*/
protected static Map> PLUGINS = new HashMap>();
/**
* Set of concrete fully qualified class names or abstract/interface base
* types to "disable". Entries in this list wont ever be returned by any of
* the getPlugin() methods. Registering an abstract/interface base name will
* disable all concrete implementations of that type
*/
protected static Set DISABLED = new HashSet();
/**
* Add the supplied list of fully qualified class names to the disabled list
*
* @param classnames a list of class names to add
*/
public static synchronized void addToDisabledList(List classnames) {
for (String s : classnames) {
addToDisabledList(s);
}
}
/**
* Add the supplied fully qualified class name to the list of disabled plugins
*
* @param classname the fully qualified name of a class to add
*/
public static synchronized void addToDisabledList(String classname) {
DISABLED.add(classname);
}
/**
* Remove the supplied list of fully qualified class names to the disabled
* list
*
* @param classnames a list of class names to remove
*/
public static synchronized void removeFromDisabledList(List classnames) {
for (String s : classnames) {
removeFromDisabledList(s);
}
}
/**
* Remove the supplied fully qualified class name from the list of disabled
* plugins
*
* @param classname the fully qualified name of a class to remove
*/
public static synchronized void removeFromDisabledList(String classname) {
DISABLED.remove(classname);
}
/**
* Returns true if the supplied fully qualified class name is in the disabled
* list
*
* @param classname the name of the class to check
* @return true if the supplied class name is in the disabled list
*/
public static boolean isInDisabledList(String classname) {
return DISABLED.contains(classname);
}
/**
* Add all key value pairs from the supplied property file
*
* @param propsFile the properties file to add
* @throws Exception if a problem occurs
*/
public static synchronized void addFromProperties(File propsFile)
throws Exception {
BufferedInputStream bi = new BufferedInputStream(new FileInputStream(
propsFile));
addFromProperties(bi);
}
/**
* Add all key value pairs from the supplied properties stream
*
* @param propsStream an input stream to a properties file
* @throws Exception if a problem occurs
*/
public static synchronized void addFromProperties(InputStream propsStream)
throws Exception {
Properties expProps = new Properties();
expProps.load(propsStream);
propsStream.close();
propsStream = null;
addFromProperties(expProps);
}
/**
* Add all key value pairs from the supplied properties object
*
* @param props a Properties object
* @throws Exception if a problem occurs
*/
public static synchronized void addFromProperties(Properties props)
throws Exception {
Set
© 2015 - 2024 Weber Informatics LLC | Privacy Policy