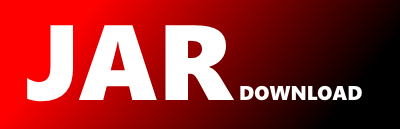
weka.classifiers.AbstractClassifier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weka-dev Show documentation
Show all versions of weka-dev Show documentation
The Waikato Environment for Knowledge Analysis (WEKA), a machine
learning workbench. This version represents the developer version, the
"bleeding edge" of development, you could say. New functionality gets added
to this version.
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* AbstractClassifier.java
* Copyright (C) 1999-2012 University of Waikato, Hamilton, New Zealand
*
*/
package weka.classifiers;
import weka.core.Attribute;
import weka.core.BatchPredictor;
import weka.core.Capabilities;
import weka.core.CapabilitiesHandler;
import weka.core.CapabilitiesIgnorer;
import weka.core.CommandlineRunnable;
import weka.core.Instance;
import weka.core.Instances;
import weka.core.Option;
import weka.core.OptionHandler;
import weka.core.RevisionHandler;
import weka.core.RevisionUtils;
import weka.core.SerializedObject;
import weka.core.Utils;
import java.io.Serializable;
import java.util.Enumeration;
import java.util.Vector;
/**
* Abstract classifier. All schemes for numeric or nominal prediction in Weka
* extend this class. Note that a classifier MUST either implement
* distributionForInstance() or classifyInstance().
*
* @author Eibe Frank ([email protected])
* @author Len Trigg ([email protected])
* @version $Revision: 14258 $
*/
public abstract class AbstractClassifier implements Classifier, BatchPredictor,
Cloneable, Serializable, OptionHandler, CapabilitiesHandler, RevisionHandler,
CapabilitiesIgnorer, CommandlineRunnable {
/** for serialization */
private static final long serialVersionUID = 6502780192411755341L;
/** Whether the classifier is run in debug mode. */
protected boolean m_Debug = false;
/** Whether capabilities should not be checked before classifier is built. */
protected boolean m_DoNotCheckCapabilities = false;
/**
* The number of decimal places used when printing numbers in the model.
*/
public static int NUM_DECIMAL_PLACES_DEFAULT = 2;
protected int m_numDecimalPlaces = NUM_DECIMAL_PLACES_DEFAULT;
/** Default preferred batch size for batch predictions */
public static String BATCH_SIZE_DEFAULT = "100";
protected String m_BatchSize = BATCH_SIZE_DEFAULT;
/**
* Creates a new instance of a classifier given it's class name and (optional)
* arguments to pass to it's setOptions method. If the classifier implements
* OptionHandler and the options parameter is non-null, the classifier will
* have it's options set.
*
* @param classifierName the fully qualified class name of the classifier
* @param options an array of options suitable for passing to setOptions. May
* be null.
* @return the newly created classifier, ready for use.
* @exception Exception if the classifier name is invalid, or the options
* supplied are not acceptable to the classifier
*/
public static Classifier forName(String classifierName, String[] options)
throws Exception {
return ((AbstractClassifier) Utils.forName(Classifier.class, classifierName,
options));
}
/**
* Creates a deep copy of the given classifier using serialization.
*
* @param model the classifier to copy
* @return a deep copy of the classifier
* @exception Exception if an error occurs
*/
public static Classifier makeCopy(Classifier model) throws Exception {
return (Classifier) new SerializedObject(model).getObject();
}
/**
* Creates a given number of deep copies of the given classifier using
* serialization.
*
* @param model the classifier to copy
* @param num the number of classifier copies to create.
* @return an array of classifiers.
* @exception Exception if an error occurs
*/
public static Classifier[] makeCopies(Classifier model, int num)
throws Exception {
if (model == null) {
throw new Exception("No model classifier set");
}
Classifier[] classifiers = new Classifier[num];
SerializedObject so = new SerializedObject(model);
for (int i = 0; i < classifiers.length; i++) {
classifiers[i] = (Classifier) so.getObject();
}
return classifiers;
}
/**
* runs the classifier instance with the given options.
*
* @param classifier the classifier to run
* @param options the commandline options
*/
public static void runClassifier(Classifier classifier, String[] options) {
try {
if (classifier instanceof CommandlineRunnable) {
((CommandlineRunnable)classifier).preExecution();
}
System.out.println(Evaluation.evaluateModel(classifier, options));
} catch (Exception e) {
if (((e.getMessage() != null)
&& (e.getMessage().indexOf("General options") == -1))
|| (e.getMessage() == null)) {
e.printStackTrace();
} else {
System.err.println(e.getMessage());
}
}
if (classifier instanceof CommandlineRunnable) {
try {
((CommandlineRunnable) classifier).postExecution();
} catch (Exception ex) {
ex.printStackTrace();
}
}
}
/**
* Classifies the given test instance. The instance has to belong to a dataset
* when it's being classified. Note that a classifier MUST implement either
* this or distributionForInstance().
*
* @param instance the instance to be classified
* @return the predicted most likely class for the instance or
* Utils.missingValue() if no prediction is made
* @exception Exception if an error occurred during the prediction
*/
@Override
public double classifyInstance(Instance instance) throws Exception {
double[] dist = distributionForInstance(instance);
if (dist == null) {
throw new Exception("Null distribution predicted");
}
switch (instance.classAttribute().type()) {
case Attribute.NOMINAL:
double max = 0;
int maxIndex = 0;
for (int i = 0; i < dist.length; i++) {
if (dist[i] > max) {
maxIndex = i;
max = dist[i];
}
}
if (max > 0) {
return maxIndex;
} else {
return Utils.missingValue();
}
case Attribute.NUMERIC:
case Attribute.DATE:
return dist[0];
default:
return Utils.missingValue();
}
}
/**
* Predicts the class memberships for a given instance. If an instance is
* unclassified, the returned array elements must be all zero. If the class is
* numeric, the array must consist of only one element, which contains the
* predicted value. Note that a classifier MUST implement either this or
* classifyInstance().
*
* @param instance the instance to be classified
* @return an array containing the estimated membership probabilities of the
* test instance in each class or the numeric prediction
* @exception Exception if distribution could not be computed successfully
*/
@Override
public double[] distributionForInstance(Instance instance) throws Exception {
double[] dist = new double[instance.numClasses()];
switch (instance.classAttribute().type()) {
case Attribute.NOMINAL:
double classification = classifyInstance(instance);
if (Utils.isMissingValue(classification)) {
return dist;
} else {
dist[(int) classification] = 1.0;
}
return dist;
case Attribute.NUMERIC:
case Attribute.DATE:
dist[0] = classifyInstance(instance);
return dist;
default:
return dist;
}
}
/**
* Returns an enumeration describing the available options.
*
* @return an enumeration of all the available options.
*/
@Override
public Enumeration
© 2015 - 2024 Weber Informatics LLC | Privacy Policy