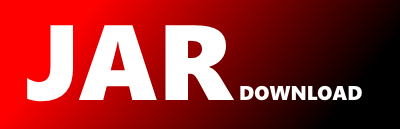
weka.core.packageManagement.PackageManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weka-dev Show documentation
Show all versions of weka-dev Show documentation
The Waikato Environment for Knowledge Analysis (WEKA), a machine
learning workbench. This version represents the developer version, the
"bleeding edge" of development, you could say. New functionality gets added
to this version.
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* PackageManager.java
* Copyright (C) 2015 University of Waikato, Hamilton, New Zealand
*
*/
package weka.core.packageManagement;
import weka.core.Defaults;
import weka.core.Settings;
import java.awt.GraphicsEnvironment;
import java.beans.Beans;
import java.io.File;
import java.io.FileInputStream;
import java.io.PrintStream;
import java.net.Authenticator;
import java.net.InetSocketAddress;
import java.net.PasswordAuthentication;
import java.net.Proxy;
import java.net.ProxySelector;
import java.net.URISyntaxException;
import java.net.URL;
import java.util.List;
import java.util.Map;
import java.util.Properties;
/**
* Abstract base class for package managers. Contains methods to manage the
* location of the central package repository, the home directory for installing
* packages, the name and version of the base software system and a http proxy.
*
* @author Mark Hall (mhall{[at]}pentaho{[dot]}com)
* @version $Revision: 52568 $
*/
public abstract class PackageManager {
public static PackageManager create() {
PackageManager pm = new DefaultPackageManager();
pm.establishProxy();
try {
// See if org.pentaho.packageManagement.manager has been set
String managerName =
System.getProperty("org.pentaho.packageManagement.manager");
if (managerName != null && managerName.length() > 0) {
Object manager =
Beans.instantiate(pm.getClass().getClassLoader(), managerName);
if (manager instanceof PackageManager) {
pm = (PackageManager) manager;
}
} else {
// See if there is a named package manager specified in
// $HOME/PackageManager.props
// that we should try to instantiate
File packageManagerPropsFile = new File(System.getProperty("user.home")
+ File.separator + "PackageManager.props");
if (packageManagerPropsFile.exists()) {
Properties pmProps = new Properties();
pmProps.load(new FileInputStream(packageManagerPropsFile));
managerName =
pmProps.getProperty("org.pentaho.packageManager.manager");
if (managerName != null && managerName.length() > 0) {
Object manager =
Beans.instantiate(pm.getClass().getClassLoader(), managerName);
if (manager instanceof PackageManager) {
pm = (PackageManager) manager;
}
}
}
}
} catch (Exception ex) {
// ignore any problems and just return the default package manager
System.err.println(
"Problem instantiating package manager. Using DefaultPackageManager.");
}
return pm;
}
/** The local directory for storing the user's installed packages */
protected File m_packageHome;
/** The URL to the global package meta data repository */
protected URL m_packageRepository;
/** The name of the base system being managed by this package manager */
protected String m_baseSystemName;
/** The version of the base system being managed by this package manager */
protected Object m_baseSystemVersion;
/** Proxy for http connections */
protected transient Proxy m_httpProxy;
/** The user name for the proxy */
protected transient String m_proxyUsername;
/** The password for the proxy */
protected transient String m_proxyPassword;
/** True if an authenticator has been set */
protected transient boolean m_authenticatorSet;
/**
* Tries to configure a Proxy object for use in an Authenticator if there is a
* proxy defined by the properties http.proxyHost and http.proxyPort, and if
* the user has set values for the properties (note, these are not standard
* java properties) http.proxyUser and http.proxyPassword.
*
*/
public void establishProxy() {
// pick up system-wide proxy if possible
// String useSystemProxies = System.getProperty(
// "weka.packageManager.useSystemProxies", "true");
//
// if (useSystemProxies.toLowerCase().equals("true")) {
// System.setProperty("java.net.useSystemProxies", "true");
// }
// check for user-supplied proxy properties
String proxyHost = System.getProperty("http.proxyHost");
String proxyPort = System.getProperty("http.proxyPort");
if (proxyHost != null && proxyHost.length() > 0) {
int portNum = 80;
if (proxyPort != null && proxyPort.length() > 0) {
portNum = Integer.parseInt(proxyPort);
}
InetSocketAddress sa = new InetSocketAddress(proxyHost, portNum);
setProxy(new Proxy(Proxy.Type.HTTP, sa));
}
// check for authentication
String proxyUserName = System.getProperty("http.proxyUser");
String proxyPassword = System.getProperty("http.proxyPassword");
if (proxyUserName != null && proxyUserName.length() > 0
&& proxyPassword != null && proxyPassword.length() > 0) {
setProxyUsername(proxyUserName);
setProxyPassword(proxyPassword);
}
}
/**
* Sets an new default Authenticator that will return the values set through
* setProxyUsername() and setProxyPassword() (if applicable).
*
* @return true if a proxy is to be used and (if applicable) the Authenticator
* was set successfully.
*/
public synchronized boolean setProxyAuthentication(URL urlToConnectTo) {
if (m_httpProxy == null) {
// try the proxy selector to see if we can get a system-wide one
ProxySelector ps = ProxySelector.getDefault();
List proxyList;
try {
proxyList = ps.select(urlToConnectTo.toURI());
Proxy proxy = proxyList.get(0);
setProxy(proxy);
} catch (URISyntaxException e) {
e.printStackTrace();
}
}
if (m_httpProxy != null) {
if (m_proxyUsername != null && m_proxyPassword != null
&& !m_authenticatorSet) {
Authenticator.setDefault(new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(m_proxyUsername,
m_proxyPassword.toCharArray());
}
});
} else {
if (!m_authenticatorSet && !GraphicsEnvironment.isHeadless()) {
Authenticator
.setDefault(new org.bounce.net.DefaultAuthenticator(null));
m_authenticatorSet = true;
}
}
return true;
}
if (m_httpProxy != null) {
return true;
}
return false;
}
/**
* Set the location (directory) of installed packages.
*
* @param packageHome the file system location of installed packages.
*/
public void setPackageHome(File packageHome) {
m_packageHome = packageHome;
}
/**
* Get the location (directory) of installed packages
*
* @return the directory containing installed packages.
*/
public File getPackageHome() {
return m_packageHome;
}
/**
* Set the name of the main software system for which we manage packages.
*
* @param baseS the name of the base software system
*/
public void setBaseSystemName(String baseS) {
m_baseSystemName = baseS;
}
/**
* Get the name of the main software system for which we manage packages.
*
* @return the name of the base software system.
*/
public String getBaseSystemName() {
return m_baseSystemName;
}
/**
* Set the current version of the base system for which we manage packages.
*
* @param systemV the current version of the main software system.
*/
public void setBaseSystemVersion(Object systemV) {
m_baseSystemVersion = systemV;
}
/**
* Get the current installed version of the main system for which we manage
* packages.
*
* @return the installed version of the base system.
*/
public Object getBaseSystemVersion() {
return m_baseSystemVersion;
}
/**
* Set the URL to the repository of package meta data.
*
* @param repositoryURL the URL to the repository of package meta data.
*/
public void setPackageRepositoryURL(URL repositoryURL) {
m_packageRepository = repositoryURL;
}
/**
* Get the URL to the repository of package meta data.
*
* @return the URL to the repository of package meta data.
*/
public URL getPackageRepositoryURL() {
return m_packageRepository;
}
/**
* Set a proxy to use for accessing the internet (default is no proxy).
*
* @param proxyToUse a proxy to use.
*/
public void setProxy(Proxy proxyToUse) {
m_httpProxy = proxyToUse;
}
/**
* Get the proxy in use.
*
* @return the proxy in use or null if no proxy is being used.
*/
public Proxy getProxy() {
return m_httpProxy;
}
/**
* Set the user name for authentication with the proxy.
*
* @param proxyUsername the user name to use for proxy authentication.
*/
public void setProxyUsername(String proxyUsername) {
m_proxyUsername = proxyUsername;
}
/**
* Set the password for authentication with the proxy.
*
* @param proxyPassword the password to use for proxy authentication.
*/
public void setProxyPassword(String proxyPassword) {
m_proxyPassword = proxyPassword;
}
/**
* Get the default settings of this package manager. Default implementation
* returns null. Subclasses to override if they have default settings
*
* @return the default settings of this package manager
*/
public Defaults getDefaultSettings() {
return null;
}
/**
* Apply the supplied settings. Default implementation does nothing.
* Subclasses should override to take note of settings changes.
*
* @param settings the settings to apply
*/
public void applySettings(Settings settings) {
}
/**
* Gets an array of bytes containing a zip of all the repository meta data and
* supporting files. Does *not* contain any package archives etc., only a
* snapshot of the meta data. Could be used by clients to establish a cache of
* meta data.
*
* @param progress optional varargs parameter, that, if supplied, is expected
* to contain one or more PrintStream objects to write progress to.
* @return a zip compressed array of bytes.
* @throws Exception if the repository meta data can't be returned as a zip
*/
public abstract byte[] getRepositoryPackageMetaDataOnlyAsZip(
PrintStream... progress) throws Exception;
/**
* Get package information from the supplied package archive file.
*
* @param packageArchivePath the path to the package archive file
* @return a Package object encapsulating the package meta data.
* @throws Exception if the package meta data can't be retrieved.
*/
public abstract Package getPackageArchiveInfo(String packageArchivePath)
throws Exception;
/**
* Get package information on the named installed package.
*
* @param packageName the name of the package to get information about.
* @return a Package object encapsulating the package meta data or null if the
* package is not installed.
*
* @throws Exception if the package meta data can't be retrieved.
*/
public abstract Package getInstalledPackageInfo(String packageName)
throws Exception;
/**
* Get package information on the named package from the repository. If
* multiple versions of the package are available, it assumes that the most
* recent is required.
*
* @param packageName the name of the package to get information about.
* @return a Package object encapsulating the package meta data.
* @throws Exception if the package meta data can't be retrieved.
*/
public abstract Package getRepositoryPackageInfo(String packageName)
throws Exception;
/**
* Get package information on the named package from the repository.
*
* @param packageName the name of the package to get information about.
* @param version the version of the package to retrieve (may be null if not
* applicable).
* @return a Package object encapsulating the package meta data.
* @throws Exception if the package meta data can't be retrieved.
*/
public abstract Package getRepositoryPackageInfo(String packageName,
Object version) throws Exception;
/**
* Get a list of available versions of the named package.
*
* @param packageName the name of the package to get versions.
* @return a list of available versions (or null if not applicable)
* @throws Exception if something goes wrong while trying to retrieve the list
* of versions.
*/
public abstract List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy