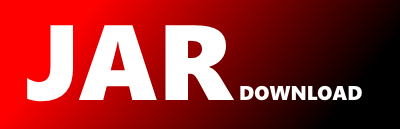
weka.classifiers.bayes.net.search.local.SimulatedAnnealing Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weka-dev Show documentation
Show all versions of weka-dev Show documentation
The Waikato Environment for Knowledge Analysis (WEKA), a machine
learning workbench. This version represents the developer version, the
"bleeding edge" of development, you could say. New functionality gets added
to this version.
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* SimulatedAnnealing.java
* Copyright (C) 2004-2012 University of Waikato, Hamilton, New Zealand
*
*/
package weka.classifiers.bayes.net.search.local;
import java.util.Collections;
import java.util.Enumeration;
import java.util.Random;
import java.util.Vector;
import weka.classifiers.bayes.BayesNet;
import weka.core.Instances;
import weka.core.Option;
import weka.core.RevisionUtils;
import weka.core.TechnicalInformation;
import weka.core.TechnicalInformation.Field;
import weka.core.TechnicalInformation.Type;
import weka.core.TechnicalInformationHandler;
import weka.core.Utils;
/**
* This Bayes Network learning algorithm uses the
* general purpose search method of simulated annealing to find a well scoring
* network structure.
*
* For more information see:
*
* R.R. Bouckaert (1995). Bayesian Belief Networks: from Construction to
* Inference. Utrecht, Netherlands.
*
*
*
* BibTeX:
*
*
* @phdthesis{Bouckaert1995,
* address = {Utrecht, Netherlands},
* author = {R.R. Bouckaert},
* institution = {University of Utrecht},
* title = {Bayesian Belief Networks: from Construction to Inference},
* year = {1995}
* }
*
*
*
*
* Valid options are:
*
*
*
* -A <float>
* Start temperature
*
*
*
* -U <integer>
* Number of runs
*
*
*
* -D <float>
* Delta temperature
*
*
*
* -R <seed>
* Random number seed
*
*
*
* -mbc
* Applies a Markov Blanket correction to the network structure,
* after a network structure is learned. This ensures that all
* nodes in the network are part of the Markov blanket of the
* classifier node.
*
*
*
* -S [BAYES|MDL|ENTROPY|AIC|CROSS_CLASSIC|CROSS_BAYES]
* Score type (BAYES, BDeu, MDL, ENTROPY and AIC)
*
*
*
*
* @author Remco Bouckaert ([email protected])
* @version $Revision: 11267 $
*/
public class SimulatedAnnealing extends LocalScoreSearchAlgorithm implements
TechnicalInformationHandler {
/** for serialization */
static final long serialVersionUID = 6951955606060513191L;
/** start temperature **/
double m_fTStart = 10;
/** change in temperature at every run **/
double m_fDelta = 0.999;
/** number of runs **/
int m_nRuns = 10000;
/** use the arc reversal operator **/
boolean m_bUseArcReversal = false;
/** random number seed **/
int m_nSeed = 1;
/** random number generator **/
Random m_random;
/**
* Returns an instance of a TechnicalInformation object, containing detailed
* information about the technical background of this class, e.g., paper
* reference or book this class is based on.
*
* @return the technical information about this class
*/
@Override
public TechnicalInformation getTechnicalInformation() {
TechnicalInformation result;
result = new TechnicalInformation(Type.PHDTHESIS);
result.setValue(Field.AUTHOR, "R.R. Bouckaert");
result.setValue(Field.YEAR, "1995");
result.setValue(Field.TITLE,
"Bayesian Belief Networks: from Construction to Inference");
result.setValue(Field.INSTITUTION, "University of Utrecht");
result.setValue(Field.ADDRESS, "Utrecht, Netherlands");
return result;
}
/**
*
* @param bayesNet the network
* @param instances the data to use
* @throws Exception if something goes wrong
*/
@Override
public void search(BayesNet bayesNet, Instances instances) throws Exception {
m_random = new Random(m_nSeed);
// determine base scores
double[] fBaseScores = new double[instances.numAttributes()];
double fCurrentScore = 0;
for (int iAttribute = 0; iAttribute < instances.numAttributes(); iAttribute++) {
fBaseScores[iAttribute] = calcNodeScore(iAttribute);
fCurrentScore += fBaseScores[iAttribute];
}
// keep track of best scoring network
double fBestScore = fCurrentScore;
BayesNet bestBayesNet = new BayesNet();
bestBayesNet.m_Instances = instances;
bestBayesNet.initStructure();
copyParentSets(bestBayesNet, bayesNet);
double fTemp = m_fTStart;
for (int iRun = 0; iRun < m_nRuns; iRun++) {
boolean bRunSucces = false;
double fDeltaScore = 0.0;
while (!bRunSucces) {
// pick two nodes at random
int iTailNode = m_random.nextInt(instances.numAttributes());
int iHeadNode = m_random.nextInt(instances.numAttributes());
while (iTailNode == iHeadNode) {
iHeadNode = m_random.nextInt(instances.numAttributes());
}
if (isArc(bayesNet, iHeadNode, iTailNode)) {
bRunSucces = true;
// either try a delete
bayesNet.getParentSet(iHeadNode).deleteParent(iTailNode, instances);
double fScore = calcNodeScore(iHeadNode);
fDeltaScore = fScore - fBaseScores[iHeadNode];
// System.out.println("Try delete " + iTailNode + "->" + iHeadNode +
// " dScore = " + fDeltaScore);
if (fTemp
* Math
.log((Math.abs(m_random.nextInt()) % 10000) / 10000.0 + 1e-100) < fDeltaScore) {
// System.out.println("success!!!");
fCurrentScore += fDeltaScore;
fBaseScores[iHeadNode] = fScore;
} else {
// roll back
bayesNet.getParentSet(iHeadNode).addParent(iTailNode, instances);
}
} else {
// try to add an arc
if (addArcMakesSense(bayesNet, instances, iHeadNode, iTailNode)) {
bRunSucces = true;
double fScore = calcScoreWithExtraParent(iHeadNode, iTailNode);
fDeltaScore = fScore - fBaseScores[iHeadNode];
// System.out.println("Try add " + iTailNode + "->" + iHeadNode +
// " dScore = " + fDeltaScore);
if (fTemp
* Math
.log((Math.abs(m_random.nextInt()) % 10000) / 10000.0 + 1e-100) < fDeltaScore) {
// System.out.println("success!!!");
bayesNet.getParentSet(iHeadNode).addParent(iTailNode, instances);
fBaseScores[iHeadNode] = fScore;
fCurrentScore += fDeltaScore;
}
}
}
}
if (fCurrentScore > fBestScore) {
copyParentSets(bestBayesNet, bayesNet);
}
fTemp = fTemp * m_fDelta;
}
copyParentSets(bayesNet, bestBayesNet);
} // buildStructure
/**
* CopyParentSets copies parent sets of source to dest BayesNet
*
* @param dest destination network
* @param source source network
*/
void copyParentSets(BayesNet dest, BayesNet source) {
int nNodes = source.getNrOfNodes();
// clear parent set first
for (int iNode = 0; iNode < nNodes; iNode++) {
dest.getParentSet(iNode).copy(source.getParentSet(iNode));
}
} // CopyParentSets
/**
* @return double
*/
public double getDelta() {
return m_fDelta;
}
/**
* @return double
*/
public double getTStart() {
return m_fTStart;
}
/**
* @return int
*/
public int getRuns() {
return m_nRuns;
}
/**
* Sets the m_fDelta.
*
* @param fDelta The m_fDelta to set
*/
public void setDelta(double fDelta) {
m_fDelta = fDelta;
}
/**
* Sets the m_fTStart.
*
* @param fTStart The m_fTStart to set
*/
public void setTStart(double fTStart) {
m_fTStart = fTStart;
}
/**
* Sets the m_nRuns.
*
* @param nRuns The m_nRuns to set
*/
public void setRuns(int nRuns) {
m_nRuns = nRuns;
}
/**
* @return random number seed
*/
public int getSeed() {
return m_nSeed;
} // getSeed
/**
* Sets the random number seed
*
* @param nSeed The number of the seed to set
*/
public void setSeed(int nSeed) {
m_nSeed = nSeed;
} // setSeed
/**
* Returns an enumeration describing the available options.
*
* @return an enumeration of all the available options.
*/
@Override
public Enumeration
© 2015 - 2024 Weber Informatics LLC | Privacy Policy