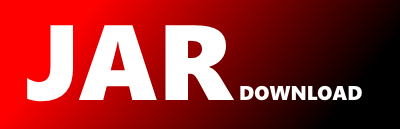
weka.core.xml.XMLBasicSerialization Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weka-dev Show documentation
Show all versions of weka-dev Show documentation
The Waikato Environment for Knowledge Analysis (WEKA), a machine
learning workbench. This version represents the developer version, the
"bleeding edge" of development, you could say. New functionality gets added
to this version.
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* XMLBasicSerialization.java
* Copyright (C) 2004-2012 University of Waikato, Hamilton, New Zealand
*
*/
package weka.core.xml;
import java.awt.Color;
import java.io.StringReader;
import java.io.StringWriter;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Hashtable;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.Map;
import java.util.Properties;
import java.util.Stack;
import java.util.TreeMap;
import java.util.TreeSet;
import java.util.Vector;
import javax.swing.DefaultListModel;
import org.w3c.dom.Element;
import weka.core.RevisionUtils;
import weka.core.Utils;
/**
* This serializer contains some read/write methods for common classes that are
* not beans-conform. Currently supported are:
*
* - java.util.HashMap
* - java.util.LinkedHashMap
* - java.util.HashSet
* - java.util.Hashtable
* - java.util.LinkedList
* - java.util.Properties
* - java.util.Stack
* - java.util.TreeMap
* - java.util.TreeSet
* - java.util.Vector
* - javax.swing.DefaultListModel
* - java.awt.Color
*
*
* Weka classes:
*
* - weka.core.Matrix
* - weka.core.matrix.Matrix
*
*
* @author FracPete (fracpete at waikato dot ac dot nz)
* @version $Revision: 11865 $
*/
public class XMLBasicSerialization extends XMLSerialization {
/** the value for mapping, e.g., Maps */
public final static String VAL_MAPPING = "mapping";
/** the value for a mapping-key, e.g., Maps */
public final static String VAL_KEY = "key";
/** the value for mapping-value, e.g., Maps */
public final static String VAL_VALUE = "value";
/** the matrix cells */
public final static String VAL_CELLS = "cells";
/**
* initializes the serialization
*
* @throws Exception if initialization fails
*/
public XMLBasicSerialization() throws Exception {
super();
}
/**
* generates internally a new XML document and clears also the IgnoreList and
* the mappings for the Read/Write-Methods
*
* @throws Exception if initializing fails
*/
@Override
@SuppressWarnings("deprecation")
public void clear() throws Exception {
super.clear();
// Java classes
m_CustomMethods.register(this, DefaultListModel.class, "DefaultListModel");
m_CustomMethods.register(this, HashMap.class, "Map");
m_CustomMethods.register(this, HashSet.class, "Collection");
m_CustomMethods.register(this, Hashtable.class, "Map");
m_CustomMethods.register(this, LinkedList.class, "Collection");
m_CustomMethods.register(this, Properties.class, "Map");
m_CustomMethods.register(this, Stack.class, "Collection");
m_CustomMethods.register(this, TreeMap.class, "Map");
m_CustomMethods.register(this, LinkedHashMap.class, "Map");
m_CustomMethods.register(this, TreeSet.class, "Collection");
m_CustomMethods.register(this, Vector.class, "Collection");
m_CustomMethods.register(this, Color.class, "Color");
// Weka classes
m_CustomMethods.register(this, weka.core.matrix.Matrix.class, "Matrix");
m_CustomMethods.register(this, weka.core.Matrix.class, "MatrixOld");
m_CustomMethods.register(this, weka.classifiers.CostMatrix.class,
"CostMatrix");
}
/**
* adds the given Color to a DOM structure.
*
* @param parent the parent of this object, e.g. the class this object is a
* member of
* @param o the Object to describe in XML
* @param name the name of the object
* @return the node that was created
* @throws Exception if the DOM creation fails
* @see java.awt.Color
*/
public Element writeColor(Element parent, Object o, String name)
throws Exception {
Element node;
Color c;
if (DEBUG) {
trace(new Throwable(), name);
}
m_CurrentNode = parent;
c = (Color) o;
node = addElement(parent, name, o.getClass().getName(), false);
invokeWriteToXML(node, c.getRed(), "red");
invokeWriteToXML(node, c.getGreen(), "green");
invokeWriteToXML(node, c.getBlue(), "blue");
return node;
}
/**
* builds the Color object from the given DOM node.
*
* @param node the associated XML node
* @return the instance created from the XML description
* @throws Exception if instantiation fails
* @see java.awt.Color
*/
public Object readColor(Element node) throws Exception {
Vector children;
if (DEBUG) {
trace(new Throwable(), node.getAttribute(ATT_NAME));
}
children = XMLDocument.getChildTags(node);
Element redchild = children.get(0);
Element greenchild = children.get(1);
Element bluechild = children.get(2);
Integer red = (Integer) readFromXML(redchild);
Integer green = (Integer) readFromXML(greenchild);
Integer blue = (Integer) readFromXML(bluechild);
return new Color(red, green, blue);
}
/**
* adds the given DefaultListModel to a DOM structure.
*
* @param parent the parent of this object, e.g. the class this object is a
* member of
* @param o the Object to describe in XML
* @param name the name of the object
* @return the node that was created
* @throws Exception if the DOM creation fails
* @see javax.swing.DefaultListModel
*/
public Element writeDefaultListModel(Element parent, Object o, String name)
throws Exception {
Element node;
int i;
DefaultListModel model;
// for debugging only
if (DEBUG) {
trace(new Throwable(), name);
}
m_CurrentNode = parent;
model = (DefaultListModel) o;
node = addElement(parent, name, o.getClass().getName(), false);
for (i = 0; i < model.getSize(); i++) {
invokeWriteToXML(node, model.get(i), Integer.toString(i));
}
return node;
}
/**
* builds the DefaultListModel from the given DOM node.
*
* @param node the associated XML node
* @return the instance created from the XML description
* @throws Exception if instantiation fails
* @see javax.swing.DefaultListModel
*/
public Object readDefaultListModel(Element node) throws Exception {
DefaultListModel model;
Vector children;
Element child;
int i;
int index;
int currIndex;
// for debugging only
if (DEBUG) {
trace(new Throwable(), node.getAttribute(ATT_NAME));
}
m_CurrentNode = node;
children = XMLDocument.getChildTags(node);
model = new DefaultListModel();
// determine highest index for size
index = children.size() - 1;
for (i = 0; i < children.size(); i++) {
child = children.get(i);
currIndex = Integer.parseInt(child.getAttribute(ATT_NAME));
if (currIndex > index) {
index = currIndex;
}
}
model.setSize(index + 1);
// set values
for (i = 0; i < children.size(); i++) {
child = children.get(i);
model.set(Integer.parseInt(child.getAttribute(ATT_NAME)),
invokeReadFromXML(child));
}
return model;
}
/**
* adds the given Collection to a DOM structure.
*
* @param parent the parent of this object, e.g. the class this object is a
* member of
* @param o the Object to describe in XML
* @param name the name of the object
* @return the node that was created
* @throws Exception if the DOM creation fails
* @see java.util.Collection
*/
public Element writeCollection(Element parent, Object o, String name)
throws Exception {
Element node;
Iterator> iter;
int i;
// for debugging only
if (DEBUG) {
trace(new Throwable(), name);
}
m_CurrentNode = parent;
iter = ((Collection>) o).iterator();
node = addElement(parent, name, o.getClass().getName(), false);
i = 0;
while (iter.hasNext()) {
invokeWriteToXML(node, iter.next(), Integer.toString(i));
i++;
}
return node;
}
/**
* builds the Collection from the given DOM node.
*
* @param node the associated XML node
* @return the instance created from the XML description
* @throws Exception if instantiation fails
* @see java.util.Collection
*/
public Object readCollection(Element node) throws Exception {
Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy