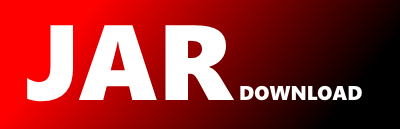
weka.classifiers.mi.MIBoost Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weka-stable Show documentation
Show all versions of weka-stable Show documentation
The Waikato Environment for Knowledge Analysis (WEKA), a machine
learning workbench. This is the stable version. Apart from bugfixes, this version
does not receive any other updates.
/*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
*/
/*
* MIBoost.java
* Copyright (C) 2005 University of Waikato, Hamilton, New Zealand
*
*/
package weka.classifiers.mi;
import weka.classifiers.Classifier;
import weka.classifiers.SingleClassifierEnhancer;
import weka.core.Capabilities;
import weka.core.Instance;
import weka.core.Instances;
import weka.core.MultiInstanceCapabilitiesHandler;
import weka.core.Optimization;
import weka.core.Option;
import weka.core.OptionHandler;
import weka.core.RevisionUtils;
import weka.core.TechnicalInformation;
import weka.core.TechnicalInformationHandler;
import weka.core.Utils;
import weka.core.WeightedInstancesHandler;
import weka.core.Capabilities.Capability;
import weka.core.TechnicalInformation.Field;
import weka.core.TechnicalInformation.Type;
import weka.filters.Filter;
import weka.filters.unsupervised.attribute.Discretize;
import weka.filters.unsupervised.attribute.MultiInstanceToPropositional;
import java.util.Enumeration;
import java.util.Vector;
/**
* MI AdaBoost method, considers the geometric mean of posterior of instances inside a bag (arithmatic mean of log-posterior) and the expectation for a bag is taken inside the loss function.
*
* For more information about Adaboost, see:
*
* Yoav Freund, Robert E. Schapire: Experiments with a new boosting algorithm. In: Thirteenth International Conference on Machine Learning, San Francisco, 148-156, 1996.
*
*
* BibTeX:
*
* @inproceedings{Freund1996,
* address = {San Francisco},
* author = {Yoav Freund and Robert E. Schapire},
* booktitle = {Thirteenth International Conference on Machine Learning},
* pages = {148-156},
* publisher = {Morgan Kaufmann},
* title = {Experiments with a new boosting algorithm},
* year = {1996}
* }
*
*
*
* Valid options are:
*
* -D
* Turn on debugging output.
*
* -B <num>
* The number of bins in discretization
* (default 0, no discretization)
*
* -R <num>
* Maximum number of boost iterations.
* (default 10)
*
* -W <class name>
* Full name of classifier to boost.
* eg: weka.classifiers.bayes.NaiveBayes
*
* -D
* If set, classifier is run in debug mode and
* may output additional info to the console
*
*
* @author Eibe Frank ([email protected])
* @author Xin Xu ([email protected])
* @version $Revision: 9144 $
*/
public class MIBoost
extends SingleClassifierEnhancer
implements OptionHandler, MultiInstanceCapabilitiesHandler,
TechnicalInformationHandler {
/** for serialization */
static final long serialVersionUID = -3808427225599279539L;
/** the models for the iterations */
protected Classifier[] m_Models;
/** The number of the class labels */
protected int m_NumClasses;
/** Class labels for each bag */
protected int[] m_Classes;
/** attributes name for the new dataset used to build the model */
protected Instances m_Attributes;
/** Number of iterations */
private int m_NumIterations = 100;
/** Voting weights of models */
protected double[] m_Beta;
/** the maximum number of boost iterations */
protected int m_MaxIterations = 10;
/** the number of discretization bins */
protected int m_DiscretizeBin = 0;
/** filter used for discretization */
protected Discretize m_Filter = null;
/** filter used to convert the MI dataset into single-instance dataset */
protected MultiInstanceToPropositional m_ConvertToSI = new MultiInstanceToPropositional();
/**
* Returns a string describing this filter
*
* @return a description of the filter suitable for
* displaying in the explorer/experimenter gui
*/
public String globalInfo() {
return
"MI AdaBoost method, considers the geometric mean of posterior "
+ "of instances inside a bag (arithmatic mean of log-posterior) and "
+ "the expectation for a bag is taken inside the loss function.\n\n"
+ "For more information about Adaboost, see:\n\n"
+ getTechnicalInformation().toString();
}
/**
* Returns an instance of a TechnicalInformation object, containing
* detailed information about the technical background of this class,
* e.g., paper reference or book this class is based on.
*
* @return the technical information about this class
*/
public TechnicalInformation getTechnicalInformation() {
TechnicalInformation result;
result = new TechnicalInformation(Type.INPROCEEDINGS);
result.setValue(Field.AUTHOR, "Yoav Freund and Robert E. Schapire");
result.setValue(Field.TITLE, "Experiments with a new boosting algorithm");
result.setValue(Field.BOOKTITLE, "Thirteenth International Conference on Machine Learning");
result.setValue(Field.YEAR, "1996");
result.setValue(Field.PAGES, "148-156");
result.setValue(Field.PUBLISHER, "Morgan Kaufmann");
result.setValue(Field.ADDRESS, "San Francisco");
return result;
}
/**
* Returns an enumeration describing the available options
*
* @return an enumeration of all the available options
*/
public Enumeration listOptions() {
Vector result = new Vector();
result.addElement(new Option(
"\tTurn on debugging output.",
"D", 0, "-D"));
result.addElement(new Option(
"\tThe number of bins in discretization\n"
+ "\t(default 0, no discretization)",
"B", 1, "-B "));
result.addElement(new Option(
"\tMaximum number of boost iterations.\n"
+ "\t(default 10)",
"R", 1, "-R "));
result.addElement(new Option(
"\tFull name of classifier to boost.\n"
+ "\teg: weka.classifiers.bayes.NaiveBayes",
"W", 1, "-W "));
Enumeration enu = ((OptionHandler)m_Classifier).listOptions();
while (enu.hasMoreElements()) {
result.addElement(enu.nextElement());
}
return result.elements();
}
/**
* Parses a given list of options.
*
* Valid options are:
*
* -D
* Turn on debugging output.
*
* -B <num>
* The number of bins in discretization
* (default 0, no discretization)
*
* -R <num>
* Maximum number of boost iterations.
* (default 10)
*
* -W <class name>
* Full name of classifier to boost.
* eg: weka.classifiers.bayes.NaiveBayes
*
* -D
* If set, classifier is run in debug mode and
* may output additional info to the console
*
*
* @param options the list of options as an array of strings
* @throws Exception if an option is not supported
*/
public void setOptions(String[] options) throws Exception {
setDebug(Utils.getFlag('D', options));
String bin = Utils.getOption('B', options);
if (bin.length() != 0) {
setDiscretizeBin(Integer.parseInt(bin));
} else {
setDiscretizeBin(0);
}
String boostIterations = Utils.getOption('R', options);
if (boostIterations.length() != 0) {
setMaxIterations(Integer.parseInt(boostIterations));
} else {
setMaxIterations(10);
}
super.setOptions(options);
}
/**
* Gets the current settings of the classifier.
*
* @return an array of strings suitable for passing to setOptions
*/
public String[] getOptions() {
Vector result;
String[] options;
int i;
result = new Vector();
result.add("-R");
result.add("" + getMaxIterations());
result.add("-B");
result.add("" + getDiscretizeBin());
options = super.getOptions();
for (i = 0; i < options.length; i++)
result.add(options[i]);
return (String[]) result.toArray(new String[result.size()]);
}
/**
* Returns the tip text for this property
*
* @return tip text for this property suitable for
* displaying in the explorer/experimenter gui
*/
public String maxIterationsTipText() {
return "The maximum number of boost iterations.";
}
/**
* Set the maximum number of boost iterations
*
* @param maxIterations the maximum number of boost iterations
*/
public void setMaxIterations(int maxIterations) {
m_MaxIterations = maxIterations;
}
/**
* Get the maximum number of boost iterations
*
* @return the maximum number of boost iterations
*/
public int getMaxIterations() {
return m_MaxIterations;
}
/**
* Returns the tip text for this property
*
* @return tip text for this property suitable for
* displaying in the explorer/experimenter gui
*/
public String discretizeBinTipText() {
return "The number of bins in discretization.";
}
/**
* Set the number of bins in discretization
*
* @param bin the number of bins in discretization
*/
public void setDiscretizeBin(int bin) {
m_DiscretizeBin = bin;
}
/**
* Get the number of bins in discretization
*
* @return the number of bins in discretization
*/
public int getDiscretizeBin() {
return m_DiscretizeBin;
}
private class OptEng
extends Optimization {
private double[] weights, errs;
public void setWeights(double[] w){
weights = w;
}
public void setErrs(double[] e){
errs = e;
}
/**
* Evaluate objective function
* @param x the current values of variables
* @return the value of the objective function
* @throws Exception if result is NaN
*/
protected double objectiveFunction(double[] x) throws Exception{
double obj=0;
for(int i=0; i 0){
m_Filter = new Discretize();
m_Filter.setInputFormat(new Instances(data, 0));
m_Filter.setBins(m_DiscretizeBin);
data = Filter.useFilter(data, m_Filter);
}
// Main algorithm
int dataIdx;
iterations:
for(int m=0; m < m_MaxIterations; m++){
if(m_Debug)
System.err.println("\nIteration "+m);
// Build a model
m_Models[m].buildClassifier(data);
// Prediction of each bag
double[] err=new double[(int)N], weights=new double[(int)N];
boolean perfect = true, tooWrong=true;
dataIdx = 0;
for(int n=0; n 0.5)
perfect = false;
if(err[n] < 0.5)
tooWrong = false;
}
if(perfect || tooWrong){ // No or 100% classification error, cannot find beta
if (m == 0)
m_Beta[m] = 1.0;
else
m_Beta[m] = 0;
m_NumIterations = m+1;
if(m_Debug) System.err.println("No errors");
break iterations;
}
double[] x = new double[1];
x[0] = 0;
double[][] b = new double[2][x.length];
b[0][0] = Double.NaN;
b[1][0] = Double.NaN;
OptEng opt = new OptEng();
opt.setWeights(weights);
opt.setErrs(err);
//opt.setDebug(m_Debug);
if (m_Debug)
System.out.println("Start searching for c... ");
x = opt.findArgmin(x, b);
while(x==null){
x = opt.getVarbValues();
if (m_Debug)
System.out.println("200 iterations finished, not enough!");
x = opt.findArgmin(x, b);
}
if (m_Debug)
System.out.println("Finished.");
m_Beta[m] = x[0];
if(m_Debug)
System.err.println("c = "+m_Beta[m]);
// Stop if error too small or error too big and ignore this model
if (Double.isInfinite(m_Beta[m])
|| Utils.smOrEq(m_Beta[m], 0)
) {
if (m == 0)
m_Beta[m] = 1.0;
else
m_Beta[m] = 0;
m_NumIterations = m+1;
if(m_Debug)
System.err.println("Errors out of range!");
break iterations;
}
// Update weights of data and class label of wfData
dataIdx=0;
double totWeights=0;
for(int r=0; r 0)
insts = Filter.useFilter(insts, m_Filter);
for(int y=0; y
© 2015 - 2025 Weber Informatics LLC | Privacy Policy