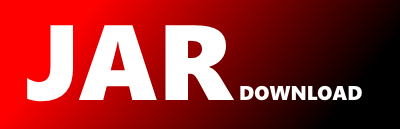
weka.experiment.RegressionSplitEvaluator Maven / Gradle / Ivy
/*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
*/
/*
* RegressionSplitEvaluator.java
* Copyright (C) 1999 University of Waikato, Hamilton, New Zealand
*
*/
package weka.experiment;
import weka.classifiers.Classifier;
import weka.classifiers.Evaluation;
import weka.classifiers.rules.ZeroR;
import weka.core.AdditionalMeasureProducer;
import weka.core.Attribute;
import weka.core.Instance;
import weka.core.Instances;
import weka.core.Option;
import weka.core.OptionHandler;
import weka.core.RevisionHandler;
import weka.core.RevisionUtils;
import weka.core.Summarizable;
import weka.core.Utils;
import java.io.ByteArrayOutputStream;
import java.io.ObjectOutputStream;
import java.io.ObjectStreamClass;
import java.io.Serializable;
import java.lang.management.ManagementFactory;
import java.lang.management.ThreadMXBean;
import java.util.Enumeration;
import java.util.Vector;
/**
* A SplitEvaluator that produces results for a classification scheme on a numeric class attribute.
*
*
* Valid options are:
*
* -W <class name>
* The full class name of the classifier.
* eg: weka.classifiers.bayes.NaiveBayes
*
*
* Options specific to classifier weka.classifiers.rules.ZeroR:
*
*
* -D
* If set, classifier is run in debug mode and
* may output additional info to the console
*
*
* @author Len Trigg ([email protected])
* @version $Revision: 7510 $
*/
public class RegressionSplitEvaluator
implements SplitEvaluator, OptionHandler, AdditionalMeasureProducer,
RevisionHandler {
/** for serialization */
static final long serialVersionUID = -328181640503349202L;
/** The template classifier */
protected Classifier m_Template = new ZeroR();
/** The classifier used for evaluation */
protected Classifier m_Classifier;
/** The names of any additional measures to look for in SplitEvaluators */
protected String [] m_AdditionalMeasures = null;
/** Array of booleans corresponding to the measures in m_AdditionalMeasures
indicating which of the AdditionalMeasures the current classifier
can produce */
protected boolean [] m_doesProduce = null;
/** Holds the statistics for the most recent application of the classifier */
protected String m_result = null;
/** The classifier options (if any) */
protected String m_ClassifierOptions = "";
/** The classifier version */
protected String m_ClassifierVersion = "";
/** The length of a key */
private static final int KEY_SIZE = 3;
/** The length of a result */
private static final int RESULT_SIZE = 21;
/**
* No args constructor.
*/
public RegressionSplitEvaluator() {
updateOptions();
}
/**
* Returns a string describing this split evaluator
* @return a description of the split evaluator suitable for
* displaying in the explorer/experimenter gui
*/
public String globalInfo() {
return "A SplitEvaluator that produces results for a classification "
+"scheme on a numeric class attribute.";
}
/**
* Returns an enumeration describing the available options..
*
* @return an enumeration of all the available options.
*/
public Enumeration listOptions() {
Vector newVector = new Vector(1);
newVector.addElement(new Option(
"\tThe full class name of the classifier.\n"
+"\teg: weka.classifiers.bayes.NaiveBayes",
"W", 1,
"-W "));
if ((m_Template != null) &&
(m_Template instanceof OptionHandler)) {
newVector.addElement(new Option(
"",
"", 0, "\nOptions specific to classifier "
+ m_Template.getClass().getName() + ":"));
Enumeration enu = ((OptionHandler)m_Template).listOptions();
while (enu.hasMoreElements()) {
newVector.addElement(enu.nextElement());
}
}
return newVector.elements();
}
/**
* Parses a given list of options.
*
* Valid options are:
*
* -W <class name>
* The full class name of the classifier.
* eg: weka.classifiers.bayes.NaiveBayes
*
*
* Options specific to classifier weka.classifiers.rules.ZeroR:
*
*
* -D
* If set, classifier is run in debug mode and
* may output additional info to the console
*
*
* All option after -- will be passed to the classifier.
*
* @param options the list of options as an array of strings
* @throws Exception if an option is not supported
*/
public void setOptions(String[] options) throws Exception {
String cName = Utils.getOption('W', options);
if (cName.length() == 0) {
throw new Exception("A classifier must be specified with"
+ " the -W option.");
}
// Do it first without options, so if an exception is thrown during
// the option setting, listOptions will contain options for the actual
// Classifier.
setClassifier(Classifier.forName(cName, null));
if (getClassifier() instanceof OptionHandler) {
((OptionHandler) getClassifier())
.setOptions(Utils.partitionOptions(options));
updateOptions();
}
}
/**
* Gets the current settings of the Classifier.
*
* @return an array of strings suitable for passing to setOptions
*/
public String [] getOptions() {
String [] classifierOptions = new String [0];
if ((m_Template != null) &&
(m_Template instanceof OptionHandler)) {
classifierOptions = ((OptionHandler)m_Template).getOptions();
}
String [] options = new String [classifierOptions.length + 3];
int current = 0;
if (getClassifier() != null) {
options[current++] = "-W";
options[current++] = getClassifier().getClass().getName();
}
options[current++] = "--";
System.arraycopy(classifierOptions, 0, options, current,
classifierOptions.length);
current += classifierOptions.length;
while (current < options.length) {
options[current++] = "";
}
return options;
}
/**
* Set a list of method names for additional measures to look for
* in Classifiers. This could contain many measures (of which only a
* subset may be produceable by the current Classifier) if an experiment
* is the type that iterates over a set of properties.
* @param additionalMeasures an array of method names.
*/
public void setAdditionalMeasures(String [] additionalMeasures) {
m_AdditionalMeasures = additionalMeasures;
// determine which (if any) of the additional measures this classifier
// can produce
if (m_AdditionalMeasures != null && m_AdditionalMeasures.length > 0) {
m_doesProduce = new boolean [m_AdditionalMeasures.length];
if (m_Template instanceof AdditionalMeasureProducer) {
Enumeration en = ((AdditionalMeasureProducer)m_Template).
enumerateMeasures();
while (en.hasMoreElements()) {
String mname = (String)en.nextElement();
for (int j=0;j classifier";
}
result.append(toString());
result.append("Classifier model: \n"+m_Classifier.toString()+'\n');
// append the performance statistics
if (m_result != null) {
result.append(m_result);
if (m_doesProduce != null) {
for (int i=0;i classifier";
}
return result + m_Template.getClass().getName() + " "
+ m_ClassifierOptions + "(version " + m_ClassifierVersion + ")";
}
/**
* Returns the revision string.
*
* @return the revision
*/
public String getRevision() {
return RevisionUtils.extract("$Revision: 7510 $");
}
} // RegressionSplitEvaluator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy