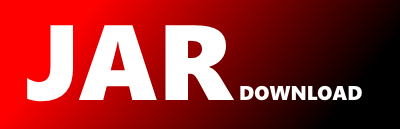
weka.classifiers.mi.MDD Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weka-stable Show documentation
Show all versions of weka-stable Show documentation
The Waikato Environment for Knowledge Analysis (WEKA), a machine
learning workbench. This is the stable version. Apart from bugfixes, this version
does not receive any other updates.
/*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
*/
/*
* MDD.java
* Copyright (C) 2005 University of Waikato, Hamilton, New Zealand
*
*/
package weka.classifiers.mi;
import weka.classifiers.Classifier;
import weka.core.Capabilities;
import weka.core.FastVector;
import weka.core.Instance;
import weka.core.Instances;
import weka.core.MultiInstanceCapabilitiesHandler;
import weka.core.Optimization;
import weka.core.Option;
import weka.core.OptionHandler;
import weka.core.RevisionUtils;
import weka.core.SelectedTag;
import weka.core.Tag;
import weka.core.TechnicalInformation;
import weka.core.TechnicalInformationHandler;
import weka.core.Utils;
import weka.core.Capabilities.Capability;
import weka.core.TechnicalInformation.Field;
import weka.core.TechnicalInformation.Type;
import weka.filters.Filter;
import weka.filters.unsupervised.attribute.Normalize;
import weka.filters.unsupervised.attribute.ReplaceMissingValues;
import weka.filters.unsupervised.attribute.Standardize;
import java.util.Enumeration;
import java.util.Vector;
/**
* Modified Diverse Density algorithm, with collective assumption.
*
* More information about DD:
*
* Oded Maron (1998). Learning from ambiguity.
*
* O. Maron, T. Lozano-Perez (1998). A Framework for Multiple Instance Learning. Neural Information Processing Systems. 10.
*
*
* BibTeX:
*
* @phdthesis{Maron1998,
* author = {Oded Maron},
* school = {Massachusetts Institute of Technology},
* title = {Learning from ambiguity},
* year = {1998}
* }
*
* @article{Maron1998,
* author = {O. Maron and T. Lozano-Perez},
* journal = {Neural Information Processing Systems},
* title = {A Framework for Multiple Instance Learning},
* volume = {10},
* year = {1998}
* }
*
*
*
* Valid options are:
*
* -D
* Turn on debugging output.
*
* -N <num>
* Whether to 0=normalize/1=standardize/2=neither.
* (default 1=standardize)
*
*
* @author Eibe Frank ([email protected])
* @author Xin Xu ([email protected])
* @version $Revision: 9144 $
*/
public class MDD
extends Classifier
implements OptionHandler, MultiInstanceCapabilitiesHandler,
TechnicalInformationHandler {
/** for serialization */
static final long serialVersionUID = -7273119490545290581L;
/** The index of the class attribute */
protected int m_ClassIndex;
protected double[] m_Par;
/** The number of the class labels */
protected int m_NumClasses;
/** Class labels for each bag */
protected int[] m_Classes;
/** MI data */
protected double[][][] m_Data;
/** All attribute names */
protected Instances m_Attributes;
/** The filter used to standardize/normalize all values. */
protected Filter m_Filter =null;
/** Whether to normalize/standardize/neither, default:standardize */
protected int m_filterType = FILTER_STANDARDIZE;
/** Normalize training data */
public static final int FILTER_NORMALIZE = 0;
/** Standardize training data */
public static final int FILTER_STANDARDIZE = 1;
/** No normalization/standardization */
public static final int FILTER_NONE = 2;
/** The filter to apply to the training data */
public static final Tag [] TAGS_FILTER = {
new Tag(FILTER_NORMALIZE, "Normalize training data"),
new Tag(FILTER_STANDARDIZE, "Standardize training data"),
new Tag(FILTER_NONE, "No normalization/standardization"),
};
/** The filter used to get rid of missing values. */
protected ReplaceMissingValues m_Missing = new ReplaceMissingValues();
/**
* Returns a string describing this filter
*
* @return a description of the filter suitable for
* displaying in the explorer/experimenter gui
*/
public String globalInfo() {
return
"Modified Diverse Density algorithm, with collective assumption.\n\n"
+ "More information about DD:\n\n"
+ getTechnicalInformation().toString();
}
/**
* Returns an instance of a TechnicalInformation object, containing
* detailed information about the technical background of this class,
* e.g., paper reference or book this class is based on.
*
* @return the technical information about this class
*/
public TechnicalInformation getTechnicalInformation() {
TechnicalInformation result;
TechnicalInformation additional;
result = new TechnicalInformation(Type.PHDTHESIS);
result.setValue(Field.AUTHOR, "Oded Maron");
result.setValue(Field.YEAR, "1998");
result.setValue(Field.TITLE, "Learning from ambiguity");
result.setValue(Field.SCHOOL, "Massachusetts Institute of Technology");
additional = result.add(Type.ARTICLE);
additional.setValue(Field.AUTHOR, "O. Maron and T. Lozano-Perez");
additional.setValue(Field.YEAR, "1998");
additional.setValue(Field.TITLE, "A Framework for Multiple Instance Learning");
additional.setValue(Field.JOURNAL, "Neural Information Processing Systems");
additional.setValue(Field.VOLUME, "10");
return result;
}
/**
* Returns an enumeration describing the available options
*
* @return an enumeration of all the available options
*/
public Enumeration listOptions() {
Vector result = new Vector();
result.addElement(new Option(
"\tTurn on debugging output.",
"D", 0, "-D"));
result.addElement(new Option(
"\tWhether to 0=normalize/1=standardize/2=neither.\n"
+ "\t(default 1=standardize)",
"N", 1, "-N "));
return result.elements();
}
/**
* Parses a given list of options.
*
* @param options the list of options as an array of strings
* @throws Exception if an option is not supported
*/
public void setOptions(String[] options) throws Exception {
setDebug(Utils.getFlag('D', options));
String nString = Utils.getOption('N', options);
if (nString.length() != 0) {
setFilterType(new SelectedTag(Integer.parseInt(nString), TAGS_FILTER));
} else {
setFilterType(new SelectedTag(FILTER_STANDARDIZE, TAGS_FILTER));
}
}
/**
* Gets the current settings of the classifier.
*
* @return an array of strings suitable for passing to setOptions
*/
public String[] getOptions() {
Vector result;
result = new Vector();
if (getDebug())
result.add("-D");
result.add("-N");
result.add("" + m_filterType);
return (String[]) result.toArray(new String[result.size()]);
}
/**
* Returns the tip text for this property
*
* @return tip text for this property suitable for
* displaying in the explorer/experimenter gui
*/
public String filterTypeTipText() {
return "The filter type for transforming the training data.";
}
/**
* Gets how the training data will be transformed. Will be one of
* FILTER_NORMALIZE, FILTER_STANDARDIZE, FILTER_NONE.
*
* @return the filtering mode
*/
public SelectedTag getFilterType() {
return new SelectedTag(m_filterType, TAGS_FILTER);
}
/**
* Sets how the training data will be transformed. Should be one of
* FILTER_NORMALIZE, FILTER_STANDARDIZE, FILTER_NONE.
*
* @param newType the new filtering mode
*/
public void setFilterType(SelectedTag newType) {
if (newType.getTags() == TAGS_FILTER) {
m_filterType = newType.getSelectedTag().getID();
}
}
private class OptEng
extends Optimization {
/**
* Evaluate objective function
* @param x the current values of variables
* @return the value of the objective function
*/
protected double objectiveFunction(double[] x){
double nll = 0; // -LogLikelihood
for(int i=0; imaxSz){
maxSz=nI;
maxSzIdx=new FastVector(1);
maxSzIdx.addElement(new Integer(h));
}
else if(nI == maxSz)
maxSzIdx.addElement(new Integer(h));
}
}
/* filter the training data */
if (m_filterType == FILTER_STANDARDIZE)
m_Filter = new Standardize();
else if (m_filterType == FILTER_NORMALIZE)
m_Filter = new Normalize();
else
m_Filter = null;
if (m_Filter!=null) {
m_Filter.setInputFormat(datasets);
datasets = Filter.useFilter(datasets, m_Filter);
}
m_Missing.setInputFormat(datasets);
datasets = Filter.useFilter(datasets, m_Missing);
int instIndex=0;
int start=0;
for(int h=0; h--------------");
}
}
}
/**
* Computes the distribution for a given exemplar
*
* @param exmp the exemplar for which distribution is computed
* @return the distribution
* @throws Exception if the distribution can't be computed successfully
*/
public double[] distributionForInstance(Instance exmp)
throws Exception {
// Extract the data
Instances ins = exmp.relationalValue(1);
if(m_Filter!=null)
ins = Filter.useFilter(ins, m_Filter);
ins = Filter.useFilter(ins, m_Missing);
int nI = ins.numInstances(), nA = ins.numAttributes();
double[][] dat = new double [nI][nA];
for(int j=0; j
© 2015 - 2025 Weber Informatics LLC | Privacy Policy