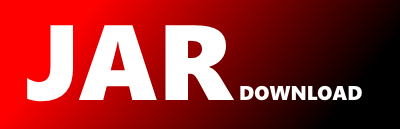
weka.core.neighboursearch.LinearNNSearch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weka-stable Show documentation
Show all versions of weka-stable Show documentation
The Waikato Environment for Knowledge Analysis (WEKA), a machine
learning workbench. This is the stable version. Apart from bugfixes, this version
does not receive any other updates.
/*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
*/
/*
* LinearNNSearch.java
* Copyright (C) 1999-2007 University of Waikato
*/
package weka.core.neighboursearch;
import weka.core.Instance;
import weka.core.Instances;
import weka.core.Option;
import weka.core.RevisionUtils;
import weka.core.Utils;
import java.util.Enumeration;
import java.util.Vector;
/**
* Class implementing the brute force search algorithm for nearest neighbour search.
*
*
* Valid options are:
*
* -S
* Skip identical instances (distances equal to zero).
*
*
*
* @author Ashraf M. Kibriya (amk14[at-the-rate]cs[dot]waikato[dot]ac[dot]nz)
* @version $Revision: 1.2 $
*/
public class LinearNNSearch
extends NearestNeighbourSearch {
/** for serialization. */
private static final long serialVersionUID = 1915484723703917241L;
/** Array holding the distances of the nearest neighbours. It is filled up
* both by nearestNeighbour() and kNearestNeighbours().
*/
protected double[] m_Distances;
/** Whether to skip instances from the neighbours that are identical to the query instance. */
protected boolean m_SkipIdentical = false;
/**
* Constructor. Needs setInstances(Instances)
* to be called before the class is usable.
*/
public LinearNNSearch() {
super();
}
/**
* Constructor that uses the supplied set of
* instances.
*
* @param insts the instances to use
*/
public LinearNNSearch(Instances insts) {
super(insts);
m_DistanceFunction.setInstances(insts);
}
/**
* Returns a string describing this nearest neighbour search algorithm.
*
* @return a description of the algorithm for displaying in the
* explorer/experimenter gui
*/
public String globalInfo() {
return
"Class implementing the brute force search algorithm for nearest "
+ "neighbour search.";
}
/**
* Returns an enumeration describing the available options.
*
* @return an enumeration of all the available options.
*/
public Enumeration listOptions() {
Vector result = new Vector();
result.add(new Option(
"\tSkip identical instances (distances equal to zero).\n",
"S", 1,"-S"));
return result.elements();
}
/**
* Parses a given list of options.
*
* Valid options are:
*
* -S
* Skip identical instances (distances equal to zero).
*
*
*
* @param options the list of options as an array of strings
* @throws Exception if an option is not supported
*/
public void setOptions(String[] options) throws Exception {
super.setOptions(options);
setSkipIdentical(Utils.getFlag('S', options));
}
/**
* Gets the current settings.
*
* @return an array of strings suitable for passing to setOptions()
*/
public String[] getOptions() {
Vector result;
String[] options;
int i;
result = new Vector();
options = super.getOptions();
for (i = 0; i < options.length; i++)
result.add(options[i]);
if (getSkipIdentical())
result.add("-S");
return result.toArray(new String[result.size()]);
}
/**
* Returns the tip text for this property.
*
* @return tip text for this property suitable for
* displaying in the explorer/experimenter gui
*/
public String skipIdenticalTipText() {
return "Whether to skip identical instances (with distance 0 to the target)";
}
/**
* Sets the property to skip identical instances (with distance zero from
* the target) from the set of neighbours returned.
*
* @param skip if true, identical intances are skipped
*/
public void setSkipIdentical(boolean skip) {
m_SkipIdentical = skip;
}
/**
* Gets whether if identical instances are skipped from the neighbourhood.
*
* @return true if identical instances are skipped
*/
public boolean getSkipIdentical() {
return m_SkipIdentical;
}
/**
* Returns the nearest instance in the current neighbourhood to the supplied
* instance.
*
* @param target The instance to find the nearest neighbour for.
* @return the nearest instance
* @throws Exception if the nearest neighbour could not be found.
*/
public Instance nearestNeighbour(Instance target) throws Exception {
return (kNearestNeighbours(target, 1)).instance(0);
}
/**
* Returns k nearest instances in the current neighbourhood to the supplied
* instance.
*
* @param target The instance to find the k nearest neighbours for.
* @param kNN The number of nearest neighbours to find.
* @return the k nearest neighbors
* @throws Exception if the neighbours could not be found.
*/
public Instances kNearestNeighbours(Instance target, int kNN) throws Exception {
//debug
boolean print=false;
if(m_Stats!=null)
m_Stats.searchStart();
MyHeap heap = new MyHeap(kNN);
double distance; int firstkNN=0;
for(int i=0; i0) {
h = heap.getKthNearest();
indices[indices.length-i] = h.index;
m_Distances[indices.length-i] = h.distance;
i++;
}
while(heap.size()>0) {
h = heap.get();
indices[indices.length-i] = h.index;
m_Distances[indices.length-i] = h.distance;
i++;
}
m_DistanceFunction.postProcessDistances(m_Distances);
for(int k=0; k
© 2015 - 2025 Weber Informatics LLC | Privacy Policy