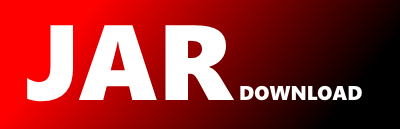
weka.gui.visualize.PlotData2D Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of weka-stable Show documentation
Show all versions of weka-stable Show documentation
The Waikato Environment for Knowledge Analysis (WEKA), a machine
learning workbench. This is the stable version. Apart from bugfixes, this version
does not receive any other updates.
/*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* PlotData2D.java
* Copyright (C) 2000-2012 University of Waikato, Hamilton, New Zealand
*
*/
package weka.gui.visualize;
import java.awt.Color;
import java.io.Serializable;
import java.util.ArrayList;
import weka.core.Instances;
import weka.filters.Filter;
import weka.filters.unsupervised.attribute.Add;
/**
* This class is a container for plottable data. Instances form the primary
* data. An optional array of classifier/clusterer predictions (associated 1 for
* 1 with the instances) can also be provided.
*
* @author Mark Hall ([email protected])
* @version $Revision: 10220 $
*/
public class PlotData2D implements Serializable {
/**
* For serialization
*/
private static final long serialVersionUID = -3979972167982697979L;
/** The instances */
protected Instances m_plotInstances = null;
/** The name of this plot */
protected String m_plotName = "new plot";
/**
* The name of this plot (possibly in html) suitable for using in a tool tip
* text.
*/
protected String m_plotNameHTML = null;
/** Custom colour for this plot */
public boolean m_useCustomColour = false;
public Color m_customColour = null;
/** Display all points (ie. those that map to the same display coords) */
public boolean m_displayAllPoints = false;
/**
* If the shape size of a point equals this size then always plot it (i.e.
* even if it is obscured by other points)
*/
public int m_alwaysDisplayPointsOfThisSize = -1;
/** Panel coordinate cache for data points */
protected double[][] m_pointLookup;
/**
* Additional optional information to control the size of points. The default
* is shape size 2
*/
protected int[] m_shapeSize;
/**
* Additional optional information to control the point shape for this data.
* Default is to allow automatic assigning of point shape on the basis of plot
* number
*/
protected int[] m_shapeType;
/**
* Additional optional information to control the drawing of lines between
* consecutive points. Setting an entry in the array to true indicates that
* the associated point should have a line connecting it to the previous
* point.
*/
protected boolean[] m_connectPoints;
/** These are used to determine bounds */
/** The x index */
private int m_xIndex;
/** The y index */
private int m_yIndex;
/** The colouring index */
private int m_cIndex;
/**
* Holds the min and max values of the x, y and colouring attributes for this
* plot
*/
protected double m_maxX;
protected double m_minX;
protected double m_maxY;
protected double m_minY;
protected double m_maxC;
protected double m_minC;
/**
* Construct a new PlotData2D using the supplied instances
*
* @param insts the instances to use.
*/
public PlotData2D(Instances insts) {
m_plotInstances = insts;
m_xIndex = m_yIndex = m_cIndex = 0;
m_pointLookup = new double[m_plotInstances.numInstances()][4];
m_shapeSize = new int[m_plotInstances.numInstances()];
m_shapeType = new int[m_plotInstances.numInstances()];
m_connectPoints = new boolean[m_plotInstances.numInstances()];
for (int i = 0; i < m_plotInstances.numInstances(); i++) {
m_shapeSize[i] = Plot2D.DEFAULT_SHAPE_SIZE; // default shape size
m_shapeType[i] = Plot2D.CONST_AUTOMATIC_SHAPE; // default (automatic shape
// assignment)
}
determineBounds();
}
/**
* Adds an instance number attribute to the plottable instances,
*/
public void addInstanceNumberAttribute() {
String originalRelationName = m_plotInstances.relationName();
int originalClassIndex = m_plotInstances.classIndex();
try {
Add addF = new Add();
addF.setAttributeName("Instance_number");
addF.setAttributeIndex("first");
addF.setInputFormat(m_plotInstances);
m_plotInstances = Filter.useFilter(m_plotInstances, addF);
m_plotInstances.setClassIndex(originalClassIndex + 1);
for (int i = 0; i < m_plotInstances.numInstances(); i++) {
m_plotInstances.instance(i).setValue(0, i);
}
m_plotInstances.setRelationName(originalRelationName);
} catch (Exception ex) {
ex.printStackTrace();
}
}
/**
* Returns the instances for this plot
*
* @return the instances for this plot
*/
public Instances getPlotInstances() {
return new Instances(m_plotInstances);
}
/**
* Set the name of this plot
*
* @param name the name for this plot
*/
public void setPlotName(String name) {
m_plotName = name;
}
/**
* Get the name of this plot
*
* @return the name of this plot
*/
public String getPlotName() {
return m_plotName;
}
/**
* Set the plot name for use in a tool tip text.
*
* @param name the name of the plot for potential use in a tool tip text (may
* use html).
*/
public void setPlotNameHTML(String name) {
m_plotNameHTML = name;
}
/**
* Get the name of the plot for use in a tool tip text. Defaults to the
* standard plot name if it hasn't been set.
*
* @return the name of this plot (possibly in html) for use in a tool tip
* text.
*/
public String getPlotNameHTML() {
if (m_plotNameHTML == null) {
return m_plotName;
}
return m_plotNameHTML;
}
/**
* Set the shape type for the plot data
*
* @param st an array of integers corresponding to shape types (see constants
* defined in Plot2D)
*/
public void setShapeType(int[] st) throws Exception {
m_shapeType = st;
if (m_shapeType.length != m_plotInstances.numInstances()) {
throw new Exception("PlotData2D: Shape type array must have the same "
+ "number of entries as number of data points!");
}
/*
* for (int i = 0; i < st.length; i++) { if (m_shapeType[i] ==
* Plot2D.ERROR_SHAPE) { m_shapeSize[i] = 3; } }
*/
}
/**
* Get the shape types for the plot data
*
* @return the shape types for the plot data
*/
public int[] getShapeType() {
return m_shapeType;
}
/**
* Set the shape type for the plot data
*
* @param st a FastVector of integers corresponding to shape types (see
* constants defined in Plot2D)
*/
public void setShapeType(ArrayList st) throws Exception {
if (st.size() != m_plotInstances.numInstances()) {
throw new Exception("PlotData2D: Shape type vector must have the same "
+ "number of entries as number of data points!");
}
m_shapeType = new int[st.size()];
for (int i = 0; i < st.size(); i++) {
m_shapeType[i] = st.get(i).intValue();
/*
* if (m_shapeType[i] == Plot2D.ERROR_SHAPE) { m_shapeSize[i] = 3; }
*/
}
}
/**
* Set the shape sizes for the plot data
*
* @param ss an array of integers specifying the size of data points
*/
public void setShapeSize(int[] ss) throws Exception {
m_shapeSize = ss;
if (m_shapeType.length != m_plotInstances.numInstances()) {
throw new Exception("PlotData2D: Shape size array must have the same "
+ "number of entries as number of data points!");
}
}
/**
* Get the shape sizes for the plot data
*
* @return the shape sizes for the plot data
*/
public int[] getShapeSize() {
return m_shapeSize;
}
/**
* Set the shape sizes for the plot data
*
* @param ss a FastVector of integers specifying the size of data points
*/
public void setShapeSize(ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy