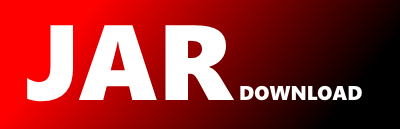
one.empty3.apps.vecmesh.PointsVoronoiHeightMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of empty3-library-3d Show documentation
Show all versions of empty3-library-3d Show documentation
3D rendering engine. Plus modelling. Expected glsl textures 3d and 2d rendering3D primitives, and a lot of scenes' samples to test.+ Game Jogl reworked, Calculator (numbers and vectors). Java code parser implementation starts (<=1.2)
The newest version!
/*
*
* * Copyright (c) 2024. Manuel Daniel Dahmen
* *
* *
* * Copyright 2024 Manuel Daniel Dahmen
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*
*/
package one.empty3.apps.vecmesh;
import one.empty3.feature.QuadTransform2D;
import one.empty3.library.ITexture;
import one.empty3.library.Point3D;
import one.empty3.library.StructureMatrix;
import one.empty3.library.core.nurbs.ParametricSurface;
import one.empty3.library.core.nurbs.Point2Point;
import one.empty3.library.core.nurbs.SurfaceParametriquePolynomialeBezier;
import one.empty3.library1.shader.Vec;
import java.util.Arrays;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.function.Function;
public class PointsVoronoiHeightMap extends ParametricSurface {
private final StructureMatrix points = new StructureMatrix<>(1, Point3D.class);
private StructureMatrix surfaceParametriquePolynomialeBezier
= new StructureMatrix<>(0, SurfaceParametriquePolynomialeBezier.class);
private StructureMatrix columnsCount = new StructureMatrix<>(0, Integer.class);
private StructureMatrix parametricSurface = new StructureMatrix<>(0, ParametricSurface.class);
public PointsVoronoiHeightMap(ParametricSurface parametricSurface, int columnsCount, Point3D ... list) {
terminalU.setElem(parametricSurface.getTerminalU().getElem());
terminalV.setElem(parametricSurface.getTerminalV().getElem());
AtomicInteger i= new AtomicInteger();
Arrays.stream(list).forEach(point3D -> {
points.setElem(point3D, i.intValue());
i.getAndIncrement();}
);
this.columnsCount.setElem(columnsCount);
this.parametricSurface.setElem(parametricSurface);
// init();
}
/*
private void init() {
Point3D[][] ps = new Point3D[columnsCount.getElem()][points.getElem().size() / columnsCount.getElem()];
try {
for (int i = 0; i < vec.getElem().size(); i++) {
ps[i % columnsCount.getElem()][i / columnsCount.getElem()] = new Point3D(vec.getElem().get(i), 0.0, 0.0);
}
surfaceParametriquePolynomialeBezier.setElem(new SurfaceParametriquePolynomialeBezier(ps));
} catch (ArrayIndexOutOfBoundsException ex) {
ex.printStackTrace();
}
}
@Override
public Point3D calculerPoint3D(double u, double v) {
int i = (int) (u * columnsCount.getElem())+vec.getElem().size()*2;
int j = (int) (v * ((vec.getElem().size() / columnsCount.getElem())))+vec.getElem().size()*2;
i = Math.round(i);
j = Math.round(j);
i = i % (columnsCount.getElem());
j = j % (vec.getElem().size()/columnsCount.getElem());
if(surfaceParametriquePolynomialeBezier.getElem()!=null) {
return parametricSurface.getElem().calculerPoint3D(u, v)
.plus(parametricSurface.getElem().calculerNormale3D(u, v)
.mult(surfaceParametriquePolynomialeBezier.getElem().calculerPoint3D(u, v)));
} else {
Double round = getControlAt(i, j);
if (round != null) {
return parametricSurface.getElem().calculerPoint3D(u, v)
.plus(parametricSurface.getElem().calculerNormale3D(u, v)
.mult(round));
}
return null;
}
}
public Double getControlAt(int x, int y) {
Double v = null;
if(y*columnsCount.getElem()+x=0
&&x>=0&&y>=0&&x
© 2015 - 2025 Weber Informatics LLC | Privacy Policy