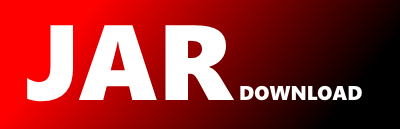
one.empty3.library.core.nurbs.NurbsSurface1 Maven / Gradle / Ivy
/*
*
* * Copyright (c) 2024. Manuel Daniel Dahmen
* *
* *
* * Copyright 2024 Manuel Daniel Dahmen
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*
*/
/*__
* *
* Global license : * Microsoft Public Licence
*
* author Manuel Dahmen [email protected]_
*
* *
*/
package one.empty3.library.core.nurbs;
import one.empty3.library.Point3D;
import one.empty3.library.core.tribase.TRIObjetGenerateurAbstract;
/*__
* Meta Description missing
* @author Manuel Dahmen [email protected]
*/
public class NurbsSurface1 extends TRIObjetGenerateurAbstract {
public static final int type_coordU = 0;
public static final int type_coordV = 1;
/*__
* *
*
* degree:
*
* Degré de la fonction de base
*
* params[]:
*
* Tableau de noeuds weights[]: * Tableau de pondérations pour des courbes
* NURBS rationnelles ; sinon NULL ou 1.0 pour une b-spline polynomiale.
* c_pnts[][3]:
*
* Tableau de points de contrôle Définition : k = degree of basis function N
* = number of knots, degree -2 wi = weights Ci = control points (coordArr, y, z) *
* wi Bi,k = basis functions Par cette équation, le nombre de points de
* contrôle est égal à N+1.
*/
private int degreeU;
private int degreeV;
private Point3D[][] maillage;
private Point3D[][] points;
private double[][] poids;
private double[][] T;
private Intervalle intervalle;
private Point3DPoids forme;
public NurbsSurface1() {
}
@Override
public Point3D coordPoint3D(int u, int v) {
return maillage[u][v];
}
public void creerNurbs() {
if (points != null && T != null && poids != null) {
intervalle = new Intervalle(T);
forme = new Point3DPoids(points, poids);
for (int i = 0; i < forme.m; i++) {
for (int j = 0; j < forme.n; j++) {
forme.set(i, j, points[i][j], poids[i][j]);
}
}
maillage = maillage();
}
}
public double f0sur0egal0(double t1, double t2) {
if (t2 == 0 && t1 == 0) {
return 0;
} else {
return t1 / t2;
}
}
public int coefficients(int type_coord, double t) {
if (t <= intervalle.get(type_coord, 0)) {
return 0;
}
for (int i = 0; i < intervalle.m; i++) {
if ((t >= intervalle.get(type_coord, i)) && (t < intervalle.get(type_coord, i + 1))) {
return i;
}
}
return 1;
}
public void setMaillage(Point3D[][] points, double[][] poids) {
this.points = points;
this.poids = poids;
}
public void setReseauFonction(double[][] T) {
this.T = T;
}
public double N(int type_coord, int i, int deg, double t) {
if (i >= intervalle.m || i < 0) {
return 0;
}
if (deg <= 0) {
if (coefficients(type_coord, t) == i) {
return 1;
} else {
return 0;
}
}
return N(type_coord, i, deg - 1, t)
* f0sur0egal0(t - intervalle.get(type_coord, i),
intervalle.get(type_coord, i + deg - 1) - intervalle.get(type_coord, i))
+ N(type_coord, i + 1, deg - 1, t)
* f0sur0egal0(intervalle.get(type_coord, i + deg + 1) - t,
intervalle.get(type_coord, i + deg + 1) - intervalle.get(type_coord, i + 1));
}
public long C(int i, int n) {
return factorielle(n) / factorielle(i) / factorielle(n - i);
}
protected long factorielle(int n) {
long sum = 1;
for (int i = 1; i <= n; i++) {
sum *= i;
}
return sum;
}
public void setDegreU(int deg) {
this.degreeU = deg;
}
public void setDegreV(int deg) {
this.degreeV = deg;
}
public Point3D[][] maillage() {
Point3D[][] qres = new Point3D[getMaxX() + 1][getMaxY() + 1];
int i;
int npts, mpts;
int k, l;
int p1, p2;
npts = points.length;
mpts = points[0].length;
k = degreeU;
l = degreeV;
double[] b = new double[npts * mpts * 4 + 1];
double[] q = new double[getMaxX() * getMaxY() * 4 + 4];
char[] header = new char[80];
int hdrlen;
/*
Data for the standard test control net.
Comment out to use file input.
*/
p1 = getMaxX();
p2 = getMaxY();
System.out.printf("k,l,npts,mpts,p1,p2 = %d %d %d %d %d %d \n", k, l, npts, mpts, p1, p2);
for (i = 1; i <= b.length - 1; i++) {
b[i] = 0.;
}
for (i = 1; i <= q.length - 1; i++) {
q[i] = 0.;
}
for (i = 1; i < points.length; i++) {
for (int j = 0; j < points[i].length; j++) {
for (int k2 = 0; k2 < 3; k2++) {
b[(j * points[i].length + i) * 4 + k2] = forme.getPoint3D(i, j).get(k2);
}
b[(j * points[i].length + i) * 4 + 3] = forme.getPoids(i, j);
}
}
Nurbs.rbspsurf(k, l, npts, mpts, p1, p2, b, q);
/* for(i=1; i= 0 && i < m && j >= 0 && j < n) {
points[i][j] = p;
poids[i][j] = w;
}
}
}
}