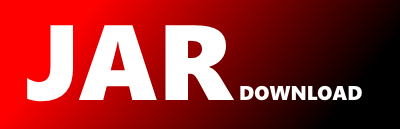
gaia.sdk.api.extension.FlowableExtension.kt Maven / Gradle / Ivy
package gaia.sdk.api.extension
import gaia.sdk.GaiaResponse
import gaia.sdk.response.type.Mutation
import gaia.sdk.response.type.Query
import org.reactivestreams.Publisher
import reactor.core.publisher.Flux
fun map(publisher: Publisher, mapper: (Query) -> T): Publisher {
return Flux.from(publisher)
.flatMap {
if ((it.errors ?: emptyList()).isNotEmpty()) {
val error = it.errors?.first()
return@flatMap Flux.error(RuntimeException(error?.message))
}
return@flatMap Flux.just(mapper(it.data!!))
}
}
fun mapM(publisher: Publisher, mapper: (Mutation) -> T): Publisher {
return Flux.from(publisher)
.flatMap {
if ((it.errors ?: emptyList()).isNotEmpty()) {
val error = it.errors?.first()
return@flatMap Flux.error(RuntimeException(error?.message))
}
return@flatMap Flux.just(mapper(it.data!!))
}
}
fun flatMap(publisher: Publisher, mapper: (Query) -> List): Publisher {
return Flux.from(publisher)
.flatMap {
if ((it.errors ?: emptyList()).isNotEmpty()) {
val error = it.errors?.first()
Flux.error(RuntimeException(error?.message))
} else {
Flux.fromIterable(mapper(it.data!!))
}
}
}
fun flatMapM(publisher: Publisher, mapper: (Mutation) -> List): Publisher {
return Flux.from(publisher)
.flatMap {
if ((it.errors ?: emptyList()).isNotEmpty()) {
val error = it.errors?.first()
Flux.error(RuntimeException(error?.message))
} else {
Flux.fromIterable(mapper(it.data!!))
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy