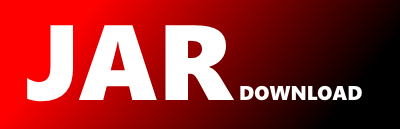
online.sanen.cdm.template.BatchUpdateUtils Maven / Gradle / Ivy
package online.sanen.cdm.template;
import java.lang.reflect.Method;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.List;
import com.mhdt.analyse.Validate;
import com.mhdt.toolkit.Reflect;
public abstract class BatchUpdateUtils {
public static int[] executeBatchUpdate(String sql, final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy