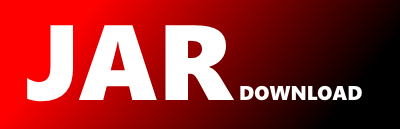
online.sanen.unabo.api.condition.SimpleCondition Maven / Gradle / Ivy
Show all versions of unabo Show documentation
package online.sanen.unabo.api.condition;
import static online.sanen.unabo.api.condition.Condition.Associated.*;
import static online.sanen.unabo.api.condition.Condition.Cs.*;
import java.util.Collection;
import java.util.List;
import java.util.function.Consumer;
import java.util.function.Supplier;
import com.mhdt.degist.Validate;
import com.mhdt.toolkit.NumberUtilly;
import lombok.Getter;
import online.sanen.unabo.api.TypeIdentifier;
import online.sanen.unabo.api.exception.ConditionException;
/**
* Query conditions Instance , In the form of objects embedded in the query
* method .
*
* fieldName - In the name of the field operation .
* value - The value Of the field .
* conditions - The relationship between the field and value
* .
*
* For example :
*
*
*
* new SimpleCondition('name',Cs.EQUALS,'zhangsan');
*
*
*
* @author LazyToshow
* Date: 2016/07/25
* Time: 14:56
*/
public class SimpleCondition implements Condition, TypeIdentifier, Comparable {
/**
* The field name in the condition
*/
private String fieldName;
private String fun = "";
@Getter
private boolean enable = true;
/**
* Concrete conditions, enumerated types
*/
private Cs cs;
/**
* The achieved value of the condition
*/
private Object value;
/**
* @see Associated
*/
private Associated associated = AND;
public SimpleCondition enable(boolean enable) {
this.enable = enable;
return this;
}
public SimpleCondition enable(Supplier supplier) {
this.enable = supplier.get();
return this;
}
/**
* By default, no arguments constructor is recommended, but you can refer to
* others
*/
public SimpleCondition() {
}
/**
* Construct a condition that has no value option
*
* @param fieldName
* @param cs
* @throws ConditionException
*/
public SimpleCondition(String fieldName, Cs cs) throws ConditionException {
this(fieldName, cs, null);
}
/**
*
* Construct a condition that has no value option but contains connection mode
*
*
* If this condition is not need value ( for example select id from tableName
* where name is not null, like this field : 'name' , because it's not neet
* value for itself )
*
* @param fieldName
* @param cs
* @param associated
* @throws ConditionException
*/
public SimpleCondition(String fieldName, Cs cs, Associated associated) throws ConditionException {
if (!cs.equals(IS_EMPTY) && !cs.equals(IS_NOT_EMPTY) && !cs.equals(IS_NULL) && !cs.equals(IS_NOT_NULL))
throw new ConditionException(String.format("this option : %s must match value. \r\n you can try to use @Condition(String fieldName,Object value,Options option)", cs.toString()));
if (associated != null) this.associated = associated;
this.fieldName = fieldName;
this.cs = cs;
}
/**
* Construct a conditional instance with a value option
*
* @param fieldName
* @param cs
* @param value
*/
public SimpleCondition(String fieldName, Cs cs, Object value) {
this(fieldName, cs, value, null);
}
/**
* if the field is need value With the corresponding
*
* @param fieldName
* @param cs
* @param value
* @param associated
*/
public SimpleCondition(String fieldName, Cs cs, Object value, Associated associated) {
if (associated != null) this.associated = associated;
this.fieldName = fieldName;
this.setValue(value);
this.cs = cs;
}
public SimpleCondition(String fieldName, Cs cs, Object value, String fun, Associated associated) {
if (associated != null) this.associated = associated;
if (fun != null) this.fun = fun;
this.fieldName = fieldName;
this.setValue(value);
this.cs = cs;
}
public Cs getCs() {
return cs;
}
public void setCs(String condition) {
this.cs = Cs.valueOf(condition);
}
public void setCs(Cs condition) {
this.cs = condition;
}
public String getFieldName() {
return fieldName;
}
public void setFieldName(String fieldName) {
this.fieldName = fieldName;
}
public Object getValue() {
Object value = null;
if ((this.cs == Cs.IN || this.cs == NOT_IN) && null!=this.value && this.value instanceof String) {
value = this.value.toString().split("\\,");
}
try{
if (valueCastType && this.value != null && this.value.getClass() == String.class && Validate.isNumber(this.value)) {
Number num = NumberUtilly.replace0AndToNumber(this.value.toString());
if (this.value.toString().contains(".")) {
value = num.doubleValue() list = (Collection