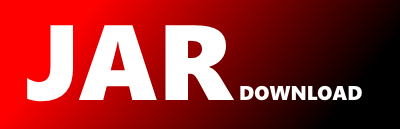
online.sanen.unabo.nosql.Container Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unabo Show documentation
Show all versions of unabo Show documentation
Unabo, the Java™ progressive ORM framework
The newest version!
package online.sanen.unabo.nosql;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Consumer;
import com.mhdt.toolkit.Assert;
import online.sanen.unabo.api.exception.StructuralException;
import online.sanen.unabo.nosql.mongodb.BootstrapMongodb;
public class Container {
static final Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy