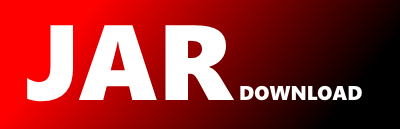
online.sanen.unabo.nosql.mongodb.PipelineNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unabo Show documentation
Show all versions of unabo Show documentation
Unabo, the Java™ progressive ORM framework
The newest version!
package online.sanen.unabo.nosql.mongodb;
import static com.mhdt.Console.log;
import static com.mongodb.client.model.Updates.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import online.sanen.unabo.nosql.mongodb.transaction.Transaction;
import online.sanen.unabo.nosql.mongodb.transaction.TransactionManager;
import org.bson.BasicBSONObject;
import org.bson.Document;
import org.bson.conversions.Bson;
import com.mhdt.degist.Validate;
import com.mhdt.toolkit.Reflect;
import com.mongodb.client.FindIterable;
import com.mongodb.client.MongoCollection;
/**
*
* @author lazyToShow
* Date: 2021年9月14日
* Time: 下午4:53:20
*/
public interface PipelineNode {
Object handel(ChannelContext context, Object message);
default Document objectToDocument(Object object, ChannelContext context) {
Document document = new Document();
for (String field : context.getCommonFields())
document.append(field, getFromObject(field, object));
return document;
}
default Bson objectToUpdate(Object object, ChannelContext context) {
List bsons = new ArrayList<>();
for (String field : context.getCommonFields()){
bsons.add(set(field, getFromObject(field, object)));
}
return combine(bsons);
}
default Object getFromObject(String field, Object object) {
String[] split = field.split("\\.");
if (split.length > 1) {
return getFromObject(field.substring(field.indexOf(".") + 1), getValueFromObject(split[0], object));
} else {
return getValueFromObject(field, object);
}
}
default Object getValueFromObject(String field, Object object) {
Object value = null;
if (object instanceof Map) {
value = ((Map, ?>) object).get(field);
} else {
value = Reflect.getInject(object, field);
}
if (Validate.isEnum(value)) {
return value.toString();
} else {
return value;
}
}
default FindIterable initFilter(ChannelContext context, MongoCollection collection) {
Transaction transaction = TransactionManager.getTransaction(context.getManager().getTemplate().getMongoClient());
if(transaction!=null){
return collection.find(transaction.getSession(),context.getFilter());
}else{
return collection.find(context.getFilter());
}
}
default FindIterable> projection(ChannelContext context, FindIterable> find) {
find.projection(projection(context));
return find;
}
default Document projection(ChannelContext context) {
Document projection = new Document();
context.getCommonFields().forEach(field -> {
if (context.getAlias().containsKey(field)) {
projection.append(context.getAlias().get(field), "$" + (field.equals("id")?"_id":field));
} else {
if (field.equals("_id")) {
projection.append(context.getAlias().getOrDefault("_id", "id"), new BasicBSONObject().append("$toString", "$_id"));
}else if(field.equals("id")){
projection.append(context.getAlias().getOrDefault("id", "id"), new BasicBSONObject().append("$toString", "$_id"));
} else {
projection.append(field, 1);
}
}
});
// (projection.containsKey("id") && context.getEntityClass()==null )
// 返回值是对象类型,不能隐藏id,隐藏后实体类的id就是null,所以返回值不是实体类才去隐藏
if ((!context.getCommonFields().contains("_id") && !context.getCommonFields().contains("id")) || (projection.containsKey("id") && context.getEntityClass() == null)){
projection.append("_id", 0);
}
if(projection.containsValue("$_id")){
projection.append("_id", 0);
}
if(context.getCommonFields().isEmpty()){
return new Document();
}
return projection;
}
default void initLimit(ChannelContext context, FindIterable> find) {
if (context.getLimit() == null || context.getLimit().length == 0 || context.getLimit().length > 2)
return;
if (context.getLimit()[0] != null && (context.getLimit().length == 1 || context.getLimit()[1] == null)) {
find.limit(context.getLimit()[0]);
} else if (context.getLimit().length == 2 && context.getLimit()[0] != null) {
find.skip(context.getLimit()[0]);
find.limit(context.getLimit()[1]);
}
}
default void initSort(ChannelContext context,FindIterable> find) {
find.sort(context.getSort());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy