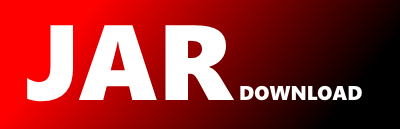
online.sanen.unabo.sql.Assembler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unabo Show documentation
Show all versions of unabo Show documentation
Unabo, the Java™ progressive ORM framework
The newest version!
package online.sanen.unabo.sql;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import com.mhdt.Print;
import com.mhdt.degist.Validate;
import com.mhdt.structure.cache.Cache;
import com.mhdt.structure.cache.FIFOCache;
import com.mhdt.structure.cache.LRUCache;
import online.sanen.unabo.api.Handel;
import online.sanen.unabo.api.PipelineFactory;
import online.sanen.unabo.api.component.Pipeline;
import online.sanen.unabo.api.exception.QueryException;
import online.sanen.unabo.api.structure.ChannelContext;
import online.sanen.unabo.api.structure.enums.QueryType;
import online.sanen.unabo.api.structure.enums.ResultType;
import online.sanen.unabo.sql.pipe.SimplePileline;
/**
* @author online.sanen
* Date: 2017/10/21
* Time: 23:19
*/
public class Assembler implements SimplePileline {
private Assembler() {
}
static Assembler assembler;
public static Assembler instance() {
if (assembler == null)
assembler = new Assembler();
return assembler;
}
/**
* @param queryType
* @param resultType
* @param context
* @param factory
* @return
*/
public Object create(QueryType queryType, ResultType resultType, ChannelContext context,
PipelineFactory factory) {
long lastTime = System.currentTimeMillis();
context.setQueryType(queryType);
context.setResultType(resultType);
Object result = null;
Pipeline pipeline = factory.getPipeline();
try {
for (Handel handel : pipeline.getHandels()) {
Object message = handel.handel(context, result);
result = (message == null ? result : message);
if (pipeline.getLast() == handel) {
print(lastTime, context);
return result;
}
}
} catch (QueryException e) {
System.out.println(String.format("[WARN] Assembler error of sql:%s", getSql(context)));
throw e;
} catch (RuntimeException e) {
System.out.println(String.format("[WARN] Assembler error of sql:%s", getSql(context)));
throw e;
} catch (Exception e) {
throw e;
}
return null;
}
private static ThreadLocal> threadLocal = new ThreadLocal<>();
private synchronized void print(long lastTime, ChannelContext context) {
if (!context.isShowSql() || this.threadLocalGet("sb") == null)
return;
StringBuilder sb = (StringBuilder) this.threadLocalGet("sb");
sb.append(String.format("Time: %ss ", (System.currentTimeMillis() - lastTime) / 1000f));
if (context.isCache() && context.getQueryType().equals(QueryType.select))
sb.append("\tcache:" + getCacheInfo(context));
System.out.println(sb.toString());
}
private String getCacheInfo(ChannelContext structure) {
try {
String cacheName = Validate.isNullOrEmpty(structure.getTableName()) ? SimplePileline.DEFAULT_CACHE
: structure.getTableName();
int size = CacheUtil.getInstance().getCache(cacheName).size();
return cacheName + "/" + size + "/" + CacheUtil.getInstance().getCache(cacheName).getCacheSize();
} catch (Exception e) {
e.printStackTrace();
return "";
}
}
public void threadLocalPut(String sign, Object object) {
if (threadLocal.get() == null)
threadLocal.set(new LRUCache<>(1000, 60));
threadLocal.get().put(String.join("_", Thread.currentThread().getName(), sign), object);
}
public Object threadLocalGet(String sign) {
if (threadLocal.get() == null)
threadLocal.set(new LRUCache<>(1000, 60));
return threadLocal.get().get(String.join("_", Thread.currentThread().getName(), sign));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy