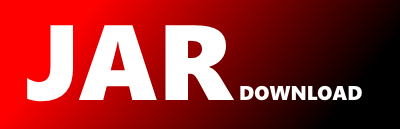
online.sanen.unabo.sql.infomation.MySQLInfomation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unabo Show documentation
Show all versions of unabo Show documentation
Unabo, the Java™ progressive ORM framework
The newest version!
package online.sanen.unabo.sql.infomation;
import java.sql.Timestamp;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import com.mhdt.Print;
import com.mhdt.toolkit.Assert;
import com.mhdt.toolkit.DateUtility;
import online.sanen.unabo.api.Bootstrap;
import online.sanen.unabo.api.structure.Column;
import online.sanen.unabo.api.structure.DataInformation;
/**
* @author LazyToShow Date: 2018/06/12 Time: 09:17
*/
public class MySQLInfomation extends DataInformation {
public MySQLInfomation(Bootstrap bootstrap) {
super(bootstrap);
}
@Override
public List getDatabases() {
String sql = "SHOW DATABASES";
return bootstrap.createSQL(sql).list();
}
@Override
public boolean containsTable(String tableName) {
List tableNames = null;
if (tableName.contains(".")) {
tableNames = bootstrap.createSQL(SQL_TABLE_NAMES, tableName.split("\\.")[0]).list();
return tableNames != null && tableNames.stream().anyMatch(it -> it.toUpperCase().equals(tableName.split("\\.")[1].toUpperCase()));
} else {
tableNames = getTablesAndViews();
return tableNames != null && tableNames.stream().anyMatch(it -> it.toUpperCase().equals(tableName.toUpperCase()));
}
}
String SQL_TABLE_NAMES = "SELECT TABLE_NAME FROM INFORMATION_SCHEMA.TABLES WHERE TABLE_SCHEMA =?";
@Override
public List getTablesAndViews() {
return bootstrap.createSQL(SQL_TABLE_NAMES, bootstrap.manager().databaseName()).list();
}
@Override
public List tables(String schema) {
return bootstrap.createSQL(SQL_TABLE_NAMES + " AND TABLE_TYPE!='VIEW'", schema).list();
}
@Override
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy