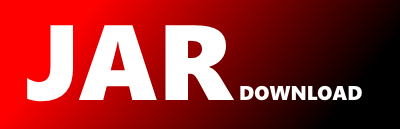
com.opensymphony.xwork2.validator.annotations.Validation Maven / Gradle / Ivy
/*
* Copyright (c) 2002-2006 by OpenSymphony
* All rights reserved.
*/
package com.opensymphony.xwork2.validator.annotations;
import java.lang.annotation.*;
/**
*
* If you want to use annotation based validation, you have to annotate the class or interface with Validation Annotation.
*
*
* Annotation usage:
*
*
* The Validation annotation must be applied at Type level.
*
*
* Annotation parameters:
*
*
*
*
* Parameter
* Required
* Default
* Notes
*
*
* message
* yes
*
* field error message
*
*
* key
* no
*
* i18n key from language specific properties file.
*
*
* fieldName
* no
*
*
*
*
* shortCircuit
* no
* false
* If this validator should be used as shortCircuit.
*
*
* type
* yes
* ValidatorType.FIELD
* Enum value from ValidatorType. Either FIELD or SIMPLE can be used here.
*
*
*
*
* Example code:
*
* An Annotated Interface
*
*
* @Validation()
* public interface AnnotationDataAware {
*
* void setBarObj(Bar b);
*
* Bar getBarObj();
*
* @RequiredFieldValidator(message = "You must enter a value for data.")
* @RequiredStringValidator(message = "You must enter a value for data.")
* void setData(String data);
*
* String getData();
* }
*
*
*
* Example code:
*
* An Annotated Class
*
*
* @Validation()
* public class SimpleAnnotationAction extends ActionSupport {
*
* @RequiredFieldValidator(type = ValidatorType.FIELD, message = "You must enter a value for bar.")
* @IntRangeFieldValidator(type = ValidatorType.FIELD, min = "6", max = "10", message = "bar must be between ${min} and ${max}, current value is ${bar}.")
* public void setBar(int bar) {
* this.bar = bar;
* }
*
* public int getBar() {
* return bar;
* }
*
* @Validations(
* requiredFields =
* {@RequiredFieldValidator(type = ValidatorType.SIMPLE, fieldName = "customfield", message = "You must enter a value for field.")},
* requiredStrings =
* {@RequiredStringValidator(type = ValidatorType.SIMPLE, fieldName = "stringisrequired", message = "You must enter a value for string.")},
* emails =
* { @EmailValidator(type = ValidatorType.SIMPLE, fieldName = "emailaddress", message = "You must enter a value for email.")},
* urls =
* { @UrlValidator(type = ValidatorType.SIMPLE, fieldName = "hreflocation", message = "You must enter a value for email.")},
* stringLengthFields =
* {@StringLengthFieldValidator(type = ValidatorType.SIMPLE, trim = true, minLength="10" , maxLength = "12", fieldName = "needstringlength", message = "You must enter a stringlength.")},
* intRangeFields =
* { @IntRangeFieldValidator(type = ValidatorType.SIMPLE, fieldName = "intfield", min = "6", max = "10", message = "bar must be between ${min} and ${max}, current value is ${bar}.")},
* dateRangeFields =
* {@DateRangeFieldValidator(type = ValidatorType.SIMPLE, fieldName = "datefield", min = "-1", max = "99", message = "bar must be between ${min} and ${max}, current value is ${bar}.")},
* expressions = {
* @ExpressionValidator(expression = "foo > 1", message = "Foo must be greater than Bar 1. Foo = ${foo}, Bar = ${bar}."),
* @ExpressionValidator(expression = "foo > 2", message = "Foo must be greater than Bar 2. Foo = ${foo}, Bar = ${bar}."),
* @ExpressionValidator(expression = "foo > 3", message = "Foo must be greater than Bar 3. Foo = ${foo}, Bar = ${bar}."),
* @ExpressionValidator(expression = "foo > 4", message = "Foo must be greater than Bar 4. Foo = ${foo}, Bar = ${bar}."),
* @ExpressionValidator(expression = "foo > 5", message = "Foo must be greater than Bar 5. Foo = ${foo}, Bar = ${bar}.")
* }
* )
* public String execute() throws Exception {
* return SUCCESS;
* }
* }
*
*
*
*
* @author Rainer Hermanns
* @version $Id: Validation.java 1187 2006-11-13 09:05:32 +0100 (Mon, 13 Nov 2006) mrdon $
*/
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
public @interface Validation {
/**
* Used for class or interface validation rules.
*/
Validations[] validations() default {};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy