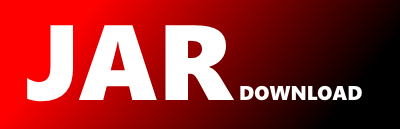
org.abego.commons.blackboard.Blackboard Maven / Gradle / Ivy
/*
* MIT License
*
* Copyright (c) 2020 Udo Borkowski, ([email protected])
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package org.abego.commons.blackboard;
import org.abego.commons.seq.Seq;
import org.eclipse.jdt.annotation.Nullable;
import java.util.function.Predicate;
/**
* A Blackboard holds a collection of items.
*
* A Blackboard is thread-safe, i.e. can be used as a mean of communication
* between multiple threads.
*
* E.g. a test method may start some asynchronous task and wait for that
* task to add a certain entry to the Blackboard.
*
* It is also useful when testing user interface code, as typically two
* threads are involved here, the main thread and the EventDispatchThread.
*/
public interface Blackboard {
/**
* Return all items of this Blackboard, in the order they were added.
*
* The result is an unmodifiable (shallow) copy of the items in the
* Blackboard. The result will not change when this Blackboard is modified.
*/
Seq items();
/**
* Return the item in the Blackboard that matches the condition
.
*
* When several items in the Blackboard match the condition return the
* last added one.
*
* When no item matches the condition throw an
* {@link java.util.NoSuchElementException}.
*/
T itemWith(Predicate condition);
/**
* Return the item in the Blackboard that matches the condition
.
*
* When several items in the Blackboard match the condition return the
* last added one.
*
* When no item matches the condition return null
.
*/
@Nullable
T itemWithOrNull(Predicate condition);
/**
* Return true
when this Blackboard is empty (does not contain
* any item), false
otherwise.
*/
boolean isEmpty();
/**
* Return true
when the Blackboard contains an item that
* matches the condition
, false
otherwise.
*/
boolean containsItemWith(Predicate condition);
/**
* Return true
when the Blackboard contains the given
* item
, false
otherwise.
*/
boolean contains(T item);
/**
* Return the items of the Blackboard as text.
*
* Process the items in the order they were added and concatenate the
* text of each item. Separate each item's text with a newline.
*
* (The text of an item is defined by item.toString()
).
*/
String text();
/**
* Add the item
to this Blackboard.
*
* @param item the item to add.
*/
void add(T item);
/**
* Remove all items from this Blackboard.
*/
void clear();
}